mirror of
https://github.com/torvalds/linux
synced 2024-10-19 17:58:44 +00:00
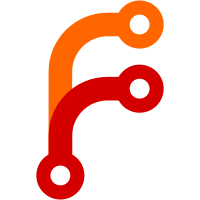
Michael reported that when using the "ocelot-8021q" tagging protocol,
the switch driver module must be manually loaded before the tagging
protocol can be loaded/is available.
This appears to be the same problem described here:
https://lore.kernel.org/netdev/20210908220834.d7gmtnwrorhharna@skbuf/
where due to the fact that DSA tagging protocols make use of symbols
exported by the switch drivers, circular dependencies appear and this
breaks module autoloading.
The ocelot_8021q driver needs the ocelot_can_inject() and
ocelot_port_inject_frame() functions from the switch library. Previously
the wrong approach was taken to solve that dependency: shims were
provided for the case where the ocelot switch library was compiled out,
but that turns out to be insufficient, because the dependency when the
switch lib _is_ compiled is problematic too.
We cannot declare ocelot_can_inject() and ocelot_port_inject_frame() as
static inline functions, because these access I/O functions like
__ocelot_write_ix() which is called by ocelot_write_rix(). Making those
static inline basically means exposing the whole guts of the ocelot
switch library, not ideal...
We already have one tagging protocol driver which calls into the switch
driver during xmit but not using any exported symbol: sja1105_defer_xmit.
We can do the same thing here: create a kthread worker and one work item
per skb, and let the switch driver itself do the register accesses to
send the skb, and then consume it.
Fixes: 0a6f17c6ae
("net: dsa: tag_ocelot_8021q: add support for PTP timestamping")
Reported-by: Michael Walle <michael@walle.cc>
Signed-off-by: Vladimir Oltean <vladimir.oltean@nxp.com>
Signed-off-by: Jakub Kicinski <kuba@kernel.org>
72 lines
2.4 KiB
C
72 lines
2.4 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/* Copyright 2019 NXP
|
|
*/
|
|
#ifndef _MSCC_FELIX_H
|
|
#define _MSCC_FELIX_H
|
|
|
|
#define ocelot_to_felix(o) container_of((o), struct felix, ocelot)
|
|
#define FELIX_MAC_QUIRKS OCELOT_QUIRK_PCS_PERFORMS_RATE_ADAPTATION
|
|
|
|
/* Platform-specific information */
|
|
struct felix_info {
|
|
const struct resource *target_io_res;
|
|
const struct resource *port_io_res;
|
|
const struct resource *imdio_res;
|
|
const struct reg_field *regfields;
|
|
const u32 *const *map;
|
|
const struct ocelot_ops *ops;
|
|
int num_mact_rows;
|
|
const struct ocelot_stat_layout *stats_layout;
|
|
unsigned int num_stats;
|
|
int num_ports;
|
|
int num_tx_queues;
|
|
struct vcap_props *vcap;
|
|
int switch_pci_bar;
|
|
int imdio_pci_bar;
|
|
const struct ptp_clock_info *ptp_caps;
|
|
|
|
/* Some Ocelot switches are integrated into the SoC without the
|
|
* extraction IRQ line connected to the ARM GIC. By enabling this
|
|
* workaround, the few packets that are delivered to the CPU port
|
|
* module (currently only PTP) are copied not only to the hardware CPU
|
|
* port module, but also to the 802.1Q Ethernet CPU port, and polling
|
|
* the extraction registers is triggered once the DSA tagger sees a PTP
|
|
* frame. The Ethernet frame is only used as a notification: it is
|
|
* dropped, and the original frame is extracted over MMIO and annotated
|
|
* with the RX timestamp.
|
|
*/
|
|
bool quirk_no_xtr_irq;
|
|
|
|
int (*mdio_bus_alloc)(struct ocelot *ocelot);
|
|
void (*mdio_bus_free)(struct ocelot *ocelot);
|
|
void (*phylink_validate)(struct ocelot *ocelot, int port,
|
|
unsigned long *supported,
|
|
struct phylink_link_state *state);
|
|
int (*prevalidate_phy_mode)(struct ocelot *ocelot, int port,
|
|
phy_interface_t phy_mode);
|
|
int (*port_setup_tc)(struct dsa_switch *ds, int port,
|
|
enum tc_setup_type type, void *type_data);
|
|
void (*port_sched_speed_set)(struct ocelot *ocelot, int port,
|
|
u32 speed);
|
|
};
|
|
|
|
extern const struct dsa_switch_ops felix_switch_ops;
|
|
|
|
/* DSA glue / front-end for struct ocelot */
|
|
struct felix {
|
|
struct dsa_switch *ds;
|
|
const struct felix_info *info;
|
|
struct ocelot ocelot;
|
|
struct mii_bus *imdio;
|
|
struct lynx_pcs **pcs;
|
|
resource_size_t switch_base;
|
|
resource_size_t imdio_base;
|
|
enum dsa_tag_protocol tag_proto;
|
|
struct kthread_worker *xmit_worker;
|
|
};
|
|
|
|
struct net_device *felix_port_to_netdev(struct ocelot *ocelot, int port);
|
|
int felix_netdev_to_port(struct net_device *dev);
|
|
|
|
#endif
|