mirror of
https://github.com/golang/go
synced 2024-09-04 23:44:16 +00:00
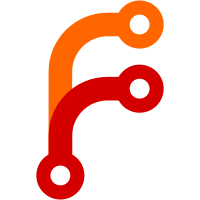
In the dict info, we need to save the SelectorExpr of a generic method call when making its sub-dictionary entry. The generic method call will eventually be transformed into a function call on the method shape instantiation, so we may not always have the selector info available when we need it to create a dictionary. We use this SelectorExpr as needed if the relevant call node has already been transformed. Similarly, we save the InstExpr of generic function calls, since the InstExpr will be dropped when the function call is transformed to a call to a shape instantiation. We use this InstExpr if the relevant function call has already been transformed. Added an extra generic function Some2 and a call to it from Some that exercises the generic function case. The existing test already tests the method call case. Fixes #50264 Change-Id: I2c7c7d79a8e33ca36a5e88e64e913c57500c97f9 Reviewed-on: https://go-review.googlesource.com/c/go/+/373754 Reviewed-by: Keith Randall <khr@golang.org> Trust: Dan Scales <danscales@google.com> Run-TryBot: Dan Scales <danscales@google.com> TryBot-Result: Gopher Robot <gobot@golang.org>
46 lines
836 B
Go
46 lines
836 B
Go
// run -gcflags=-G=3
|
|
|
|
// Copyright 2021 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
type hello struct{}
|
|
|
|
func main() {
|
|
_ = Some(hello{})
|
|
res := Applicative2(func(a int, b int) int {
|
|
return 0
|
|
})
|
|
_ = res
|
|
}
|
|
|
|
type NoneType[T any] struct{}
|
|
|
|
func (r NoneType[T]) Recover() any {
|
|
return nil
|
|
}
|
|
|
|
type Func2[A1, A2, R any] func(a1 A1, a2 A2) R
|
|
|
|
func Some[T any](v T) any {
|
|
_ = Some2[T](v)
|
|
return NoneType[T]{}.Recover()
|
|
}
|
|
|
|
//go:noinline
|
|
func Some2[T any](v T) any {
|
|
return v
|
|
}
|
|
|
|
type Nil struct{}
|
|
|
|
type ApplicativeFunctor2[H, HT, A1, A2, R any] struct {
|
|
h any
|
|
}
|
|
|
|
func Applicative2[A1, A2, R any](fn Func2[A1, A2, R]) ApplicativeFunctor2[Nil, Nil, A1, A2, R] {
|
|
return ApplicativeFunctor2[Nil, Nil, A1, A2, R]{Some(Nil{})}
|
|
}
|