mirror of
https://github.com/godotengine/godot
synced 2024-11-02 10:25:48 +00:00
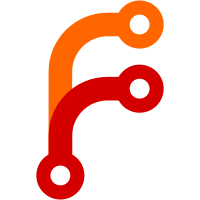
Also finally move freetype to its own env and disable warnings for it. Still needs some work to fix the awkward situation of the freetype and svg modules used in scene/ and editor/ respectively.
94 lines
3.2 KiB
Python
94 lines
3.2 KiB
Python
#!/usr/bin/env python
|
|
|
|
Import('env')
|
|
|
|
env.editor_sources = []
|
|
|
|
import os
|
|
import os.path
|
|
from platform_methods import run_in_subprocess
|
|
from compat import open_utf8
|
|
import editor_builders
|
|
|
|
|
|
def _make_doc_data_class_path(to_path):
|
|
# NOTE: It is safe to generate this file here, since this is still executed serially
|
|
g = open_utf8(os.path.join(to_path, "doc_data_class_path.gen.h"), "w")
|
|
g.write("static const int _doc_data_class_path_count = " + str(len(env.doc_class_path)) + ";\n")
|
|
g.write("struct _DocDataClassPath { const char* name; const char* path; };\n")
|
|
|
|
g.write("static const _DocDataClassPath _doc_data_class_paths[" + str(len(env.doc_class_path) + 1) + "] = {\n");
|
|
for c in sorted(env.doc_class_path):
|
|
g.write("\t{\"" + c + "\", \"" + env.doc_class_path[c] + "\"},\n")
|
|
g.write("\t{NULL, NULL}\n")
|
|
g.write("};\n")
|
|
|
|
g.close()
|
|
|
|
|
|
if env['tools']:
|
|
# Register exporters
|
|
reg_exporters_inc = '#include "register_exporters.h"\n'
|
|
reg_exporters = 'void register_exporters() {\n'
|
|
for e in env.platform_exporters:
|
|
env.editor_sources.append("#platform/" + e + "/export/export.cpp")
|
|
reg_exporters += '\tregister_' + e + '_exporter();\n'
|
|
reg_exporters_inc += '#include "platform/' + e + '/export/export.h"\n'
|
|
reg_exporters += '}\n'
|
|
|
|
# NOTE: It is safe to generate this file here, since this is still executed serially
|
|
with open_utf8("register_exporters.gen.cpp", "w") as f:
|
|
f.write(reg_exporters_inc)
|
|
f.write(reg_exporters)
|
|
|
|
# API documentation
|
|
docs = []
|
|
doc_dirs = ["doc/classes"]
|
|
|
|
for p in env.doc_class_path.values():
|
|
if p not in doc_dirs:
|
|
doc_dirs.append(p)
|
|
|
|
for d in doc_dirs:
|
|
try:
|
|
for f in os.listdir(os.path.join(env.Dir('#').abspath, d)):
|
|
docs.append("#" + os.path.join(d, f))
|
|
except OSError:
|
|
pass
|
|
|
|
_make_doc_data_class_path(os.path.join(env.Dir('#').abspath, "editor/doc"))
|
|
|
|
docs = sorted(docs)
|
|
env.Depends("#editor/doc_data_compressed.gen.h", docs)
|
|
env.CommandNoCache("#editor/doc_data_compressed.gen.h", docs, run_in_subprocess(editor_builders.make_doc_header))
|
|
|
|
import glob
|
|
|
|
path = env.Dir('.').abspath
|
|
|
|
# Translations
|
|
tlist = glob.glob(path + "/translations/*.po")
|
|
env.Depends('#editor/translations.gen.h', tlist)
|
|
env.CommandNoCache('#editor/translations.gen.h', tlist, run_in_subprocess(editor_builders.make_translations_header))
|
|
|
|
# Fonts
|
|
flist = glob.glob(path + "/../thirdparty/fonts/*.ttf")
|
|
flist.extend(glob.glob(path + "/../thirdparty/fonts/*.otf"))
|
|
flist.sort()
|
|
env.Depends('#editor/builtin_fonts.gen.h', flist)
|
|
env.CommandNoCache('#editor/builtin_fonts.gen.h', flist, run_in_subprocess(editor_builders.make_fonts_header))
|
|
|
|
env.add_source_files(env.editor_sources, "*.cpp")
|
|
env_thirdparty = env.Clone()
|
|
env_thirdparty.disable_warnings()
|
|
env_thirdparty.add_source_files(env.editor_sources, ["#thirdparty/misc/clipper.cpp"])
|
|
|
|
SConscript('collada/SCsub')
|
|
SConscript('doc/SCsub')
|
|
SConscript('fileserver/SCsub')
|
|
SConscript('icons/SCsub')
|
|
SConscript('import/SCsub')
|
|
SConscript('plugins/SCsub')
|
|
|
|
lib = env.add_library("editor", env.editor_sources)
|
|
env.Prepend(LIBS=[lib])
|