mirror of
https://github.com/Jguer/yay
synced 2024-09-14 13:51:12 +00:00
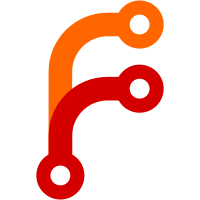
This moves the config parsing from out of alpm and into the go-pacmanconf libary plus some boilerplate code to get it into our alpm config. This makes sense as many config options such as UseColor and CleanMethod have nothing to do with alpm and only relate to pacman. pacman-conf is used instead of direct config parsing. This tool resolves defaults and includes for us, so we don't need to handle it. It is now safe to drop all the config parsing from go-alpm.
200 lines
3.8 KiB
Go
200 lines
3.8 KiB
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"io/ioutil"
|
|
"os"
|
|
"path/filepath"
|
|
)
|
|
|
|
// GetPkgbuild gets the pkgbuild of the package 'pkg' trying the ABS first and then the AUR trying the ABS first and then the AUR.
|
|
|
|
// RemovePackage removes package from VCS information
|
|
func removeVCSPackage(pkgs []string) {
|
|
updated := false
|
|
|
|
for _, pkgName := range pkgs {
|
|
if _, ok := savedInfo[pkgName]; ok {
|
|
delete(savedInfo, pkgName)
|
|
updated = true
|
|
}
|
|
}
|
|
|
|
if updated {
|
|
saveVCSInfo()
|
|
}
|
|
}
|
|
|
|
// CleanDependencies removes all dangling dependencies in system
|
|
func cleanDependencies(removeOptional bool) error {
|
|
hanging, err := hangingPackages(removeOptional)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
if len(hanging) != 0 {
|
|
return cleanRemove(hanging)
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// CleanRemove sends a full removal command to pacman with the pkgName slice
|
|
func cleanRemove(pkgNames []string) (err error) {
|
|
if len(pkgNames) == 0 {
|
|
return nil
|
|
}
|
|
|
|
arguments := makeArguments()
|
|
arguments.addArg("R")
|
|
arguments.addTarget(pkgNames...)
|
|
|
|
return show(passToPacman(arguments))
|
|
}
|
|
|
|
func syncClean(parser *arguments) error {
|
|
var err error
|
|
keepInstalled := false
|
|
keepCurrent := false
|
|
|
|
_, removeAll, _ := parser.getArg("c", "clean")
|
|
|
|
for _, v := range pacmanConf.CleanMethod {
|
|
if v == "KeepInstalled" {
|
|
keepInstalled = true
|
|
} else if v == "KeepCurrent" {
|
|
keepCurrent = true
|
|
}
|
|
}
|
|
|
|
if mode == ModeRepo || mode == ModeAny {
|
|
if err = show(passToPacman(parser)); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
|
|
if !(mode == ModeAUR || mode == ModeAny) {
|
|
return nil
|
|
}
|
|
|
|
var question string
|
|
if removeAll {
|
|
question = "Do you want to remove ALL AUR packages from cache?"
|
|
} else {
|
|
question = "Do you want to remove all other AUR packages from cache?"
|
|
}
|
|
|
|
fmt.Printf("\nBuild directory: %s\n", config.BuildDir)
|
|
|
|
if continueTask(question, true) {
|
|
err = cleanAUR(keepInstalled, keepCurrent, removeAll)
|
|
}
|
|
|
|
if err != nil || removeAll {
|
|
return err
|
|
}
|
|
|
|
if continueTask("Do you want to remove ALL untracked AUR files?", true) {
|
|
return cleanUntracked()
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
func cleanAUR(keepInstalled, keepCurrent, removeAll bool) error {
|
|
fmt.Println("removing AUR packages from cache...")
|
|
|
|
installedBases := make(stringSet)
|
|
inAURBases := make(stringSet)
|
|
|
|
_, remotePackages, _, _, err := filterPackages()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
files, err := ioutil.ReadDir(config.BuildDir)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
cachedPackages := make([]string, 0, len(files))
|
|
for _, file := range files {
|
|
if !file.IsDir() {
|
|
continue
|
|
}
|
|
|
|
cachedPackages = append(cachedPackages, file.Name())
|
|
}
|
|
|
|
// Most people probably don't use keep current and that is the only
|
|
// case where this is needed.
|
|
// Querying the AUR is slow and needs internet so don't do it if we
|
|
// don't need to.
|
|
if keepCurrent {
|
|
info, err := aurInfo(cachedPackages, &aurWarnings{})
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
for _, pkg := range info {
|
|
inAURBases.set(pkg.PackageBase)
|
|
}
|
|
}
|
|
|
|
for _, pkg := range remotePackages {
|
|
if pkg.Base() != "" {
|
|
installedBases.set(pkg.Base())
|
|
} else {
|
|
installedBases.set(pkg.Name())
|
|
}
|
|
}
|
|
|
|
for _, file := range files {
|
|
if !file.IsDir() {
|
|
continue
|
|
}
|
|
|
|
if !removeAll {
|
|
if keepInstalled && installedBases.get(file.Name()) {
|
|
continue
|
|
}
|
|
|
|
if keepCurrent && inAURBases.get(file.Name()) {
|
|
continue
|
|
}
|
|
}
|
|
|
|
err = os.RemoveAll(filepath.Join(config.BuildDir, file.Name()))
|
|
if err != nil {
|
|
return nil
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
func cleanUntracked() error {
|
|
fmt.Println("removing Untracked AUR files from cache...")
|
|
|
|
files, err := ioutil.ReadDir(config.BuildDir)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
for _, file := range files {
|
|
if !file.IsDir() {
|
|
continue
|
|
}
|
|
|
|
dir := filepath.Join(config.BuildDir, file.Name())
|
|
|
|
if shouldUseGit(dir) {
|
|
if err = show(passToGit(dir, "clean", "-fx")); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|