mirror of
git://source.winehq.org/git/wine.git
synced 2024-11-05 18:01:34 +00:00
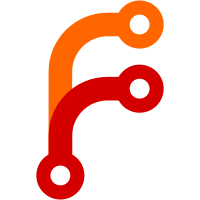
control to it, and then have ntdll load kernel32 using the normal loader mechanisms. Get rid of BUILTIN32_LoadExeModule.
206 lines
7.6 KiB
C
206 lines
7.6 KiB
C
/*
|
|
* Module definitions
|
|
*
|
|
* Copyright 1995 Alexandre Julliard
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2.1 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
|
|
*/
|
|
|
|
#ifndef __WINE_MODULE_H
|
|
#define __WINE_MODULE_H
|
|
|
|
#include <stdarg.h>
|
|
#include <windef.h>
|
|
#include <winbase.h>
|
|
#include <winreg.h>
|
|
#include <wine/windef16.h>
|
|
#include <wine/winbase16.h>
|
|
#include <winternl.h>
|
|
|
|
/* In-memory module structure. See 'Windows Internals' p. 219 */
|
|
typedef struct _NE_MODULE
|
|
{
|
|
WORD magic; /* 00 'NE' signature */
|
|
WORD count; /* 02 Usage count */
|
|
WORD entry_table; /* 04 Near ptr to entry table */
|
|
HMODULE16 next; /* 06 Selector to next module */
|
|
WORD dgroup_entry; /* 08 Near ptr to segment entry for DGROUP */
|
|
WORD fileinfo; /* 0a Near ptr to file info (OFSTRUCT) */
|
|
WORD flags; /* 0c Module flags */
|
|
WORD dgroup; /* 0e Logical segment for DGROUP */
|
|
WORD heap_size; /* 10 Initial heap size */
|
|
WORD stack_size; /* 12 Initial stack size */
|
|
WORD ip; /* 14 Initial ip */
|
|
WORD cs; /* 16 Initial cs (logical segment) */
|
|
WORD sp; /* 18 Initial stack pointer */
|
|
WORD ss; /* 1a Initial ss (logical segment) */
|
|
WORD seg_count; /* 1c Number of segments in segment table */
|
|
WORD modref_count; /* 1e Number of module references */
|
|
WORD nrname_size; /* 20 Size of non-resident names table */
|
|
WORD seg_table; /* 22 Near ptr to segment table */
|
|
WORD res_table; /* 24 Near ptr to resource table */
|
|
WORD name_table; /* 26 Near ptr to resident names table */
|
|
WORD modref_table; /* 28 Near ptr to module reference table */
|
|
WORD import_table; /* 2a Near ptr to imported names table */
|
|
DWORD nrname_fpos; /* 2c File offset of non-resident names table */
|
|
WORD moveable_entries; /* 30 Number of moveable entries in entry table*/
|
|
WORD alignment; /* 32 Alignment shift count */
|
|
WORD truetype; /* 34 Set to 2 if TrueType font */
|
|
BYTE os_flags; /* 36 Operating system flags */
|
|
BYTE misc_flags; /* 37 Misc. flags */
|
|
HANDLE16 dlls_to_init; /* 38 List of DLLs to initialize */
|
|
HANDLE16 nrname_handle; /* 3a Handle to non-resident name table */
|
|
WORD min_swap_area; /* 3c Min. swap area size */
|
|
WORD expected_version; /* 3e Expected Windows version */
|
|
/* From here, these are extra fields not present in normal Windows */
|
|
HMODULE module32; /* 40 PE module handle for Win32 modules */
|
|
HMODULE16 self; /* 44 Handle for this module */
|
|
WORD self_loading_sel; /* 46 Selector used for self-loading apps. */
|
|
LPVOID hRsrcMap; /* HRSRC 16->32 map (for 32-bit modules) */
|
|
HANDLE fd; /* handle to the binary file */
|
|
} NE_MODULE;
|
|
|
|
|
|
typedef struct {
|
|
BYTE type;
|
|
BYTE flags;
|
|
BYTE segnum;
|
|
WORD offs WINE_PACKED;
|
|
} ET_ENTRY;
|
|
|
|
typedef struct {
|
|
WORD first; /* ordinal */
|
|
WORD last; /* ordinal */
|
|
WORD next; /* bundle */
|
|
} ET_BUNDLE;
|
|
|
|
|
|
/* In-memory segment table */
|
|
typedef struct
|
|
{
|
|
WORD filepos; /* Position in file, in sectors */
|
|
WORD size; /* Segment size on disk */
|
|
WORD flags; /* Segment flags */
|
|
WORD minsize; /* Min. size of segment in memory */
|
|
HANDLE16 hSeg; /* Selector or handle (selector - 1) */
|
|
/* of segment in memory */
|
|
} SEGTABLEENTRY;
|
|
|
|
|
|
/* Self-loading modules contain this structure in their first segment */
|
|
|
|
#include <pshpack1.h>
|
|
|
|
typedef struct
|
|
{
|
|
WORD version; /* Must be "A0" (0x3041) */
|
|
WORD reserved;
|
|
FARPROC16 BootApp; /* startup procedure */
|
|
FARPROC16 LoadAppSeg; /* procedure to load a segment */
|
|
FARPROC16 reserved2;
|
|
FARPROC16 MyAlloc; /* memory allocation procedure,
|
|
* wine must write this field */
|
|
FARPROC16 EntryAddrProc;
|
|
FARPROC16 ExitProc; /* exit procedure */
|
|
WORD reserved3[4];
|
|
FARPROC16 SetOwner; /* Set Owner procedure, exported by wine */
|
|
} SELFLOADHEADER;
|
|
|
|
typedef struct
|
|
{
|
|
LPSTR lpEnvAddress;
|
|
LPSTR lpCmdLine;
|
|
UINT16 *lpCmdShow;
|
|
DWORD dwReserved;
|
|
} LOADPARAMS;
|
|
|
|
#include <poppack.h>
|
|
|
|
/* Resource types */
|
|
|
|
#define NE_SEG_TABLE(pModule) \
|
|
((SEGTABLEENTRY *)((char *)(pModule) + (pModule)->seg_table))
|
|
|
|
#define NE_MODULE_TABLE(pModule) \
|
|
((WORD *)((char *)(pModule) + (pModule)->modref_table))
|
|
|
|
#define NE_MODULE_NAME(pModule) \
|
|
(((OFSTRUCT *)((char*)(pModule) + (pModule)->fileinfo))->szPathName)
|
|
|
|
|
|
enum loadorder_type
|
|
{
|
|
LOADORDER_INVALID = 0, /* Must be 0 */
|
|
LOADORDER_DLL, /* Native DLLs */
|
|
LOADORDER_BI, /* Built-in modules */
|
|
LOADORDER_NTYPES
|
|
};
|
|
|
|
/* return values for MODULE_GetBinaryType */
|
|
enum binary_type
|
|
{
|
|
BINARY_UNKNOWN,
|
|
BINARY_PE_EXE,
|
|
BINARY_PE_DLL,
|
|
BINARY_WIN16,
|
|
BINARY_OS216,
|
|
BINARY_DOS,
|
|
BINARY_UNIX_EXE,
|
|
BINARY_UNIX_LIB
|
|
};
|
|
|
|
/* module.c */
|
|
extern NTSTATUS MODULE_DllThreadAttach( LPVOID lpReserved );
|
|
extern enum binary_type MODULE_GetBinaryType( HANDLE hfile );
|
|
extern FARPROC16 WINAPI WIN32_GetProcAddress16( HMODULE hmodule, LPCSTR name );
|
|
extern void MODULE_WalkModref( DWORD id );
|
|
|
|
/* loader/ne/module.c */
|
|
extern NE_MODULE *NE_GetPtr( HMODULE16 hModule );
|
|
extern void NE_DumpModule( HMODULE16 hModule );
|
|
extern void NE_WalkModules(void);
|
|
extern void NE_InitResourceHandler( NE_MODULE *pModule );
|
|
extern void NE_RegisterModule( NE_MODULE *pModule );
|
|
extern WORD NE_GetOrdinal( HMODULE16 hModule, const char *name );
|
|
extern FARPROC16 WINAPI NE_GetEntryPoint( HMODULE16 hModule, WORD ordinal );
|
|
extern FARPROC16 NE_GetEntryPointEx( HMODULE16 hModule, WORD ordinal, BOOL16 snoop );
|
|
extern BOOL16 NE_SetEntryPoint( HMODULE16 hModule, WORD ordinal, WORD offset );
|
|
extern HANDLE NE_OpenFile( NE_MODULE *pModule );
|
|
extern DWORD NE_StartTask(void);
|
|
|
|
/* loader/ne/resource.c */
|
|
extern HGLOBAL16 WINAPI NE_DefResourceHandler(HGLOBAL16,HMODULE16,HRSRC16);
|
|
|
|
/* loader/ne/segment.c */
|
|
extern BOOL NE_LoadSegment( NE_MODULE *pModule, WORD segnum );
|
|
extern BOOL NE_LoadAllSegments( NE_MODULE *pModule );
|
|
extern BOOL NE_CreateSegment( NE_MODULE *pModule, int segnum );
|
|
extern BOOL NE_CreateAllSegments( NE_MODULE *pModule );
|
|
extern HINSTANCE16 NE_GetInstance( NE_MODULE *pModule );
|
|
extern void NE_InitializeDLLs( HMODULE16 hModule );
|
|
extern void NE_DllProcessAttach( HMODULE16 hModule );
|
|
extern void NE_CallUserSignalProc( HMODULE16 hModule, UINT16 code );
|
|
|
|
/* loader/pe_resource.c */
|
|
extern HRSRC PE_FindResourceW(HMODULE,LPCWSTR,LPCWSTR);
|
|
extern HRSRC PE_FindResourceExW(HMODULE,LPCWSTR,LPCWSTR,WORD);
|
|
|
|
/* loader/loadorder.c */
|
|
extern void MODULE_GetLoadOrderW( enum loadorder_type plo[], const WCHAR *app_name,
|
|
const WCHAR *path, BOOL win32 );
|
|
extern void MODULE_GetLoadOrderA( enum loadorder_type plo[], const WCHAR *app_name,
|
|
const char *path, BOOL win32 );
|
|
|
|
#endif /* __WINE_MODULE_H */
|