mirror of
git://source.winehq.org/git/wine.git
synced 2024-10-31 10:13:56 +00:00
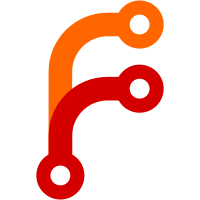
Sun Feb 15 12:02:59 1998 Alexandre Julliard <julliard@lrc.epfl.ch> * [graphics/x11drv/*.c] [objects/*.c] A few X11 critical section optimizations, mostly with XGet/PutPixel. * [scheduler/sysdeps.c] [misc/main.c] Make sure X11 critical section is available before any Xlib call. * [if1632/relay.c] [tools/build.c] Yet another attempt at fixing Catch/Throw. * [loader/pe_image.c] Fixed broken PE DLL loading. * [include/winnt.h] [scheduler/handle.c] [scheduler/*.c] Implemented handle access rights. Added Get/SetHandleInformation. Sun Feb 15 09:45:23 1997 Andreas Mohr <100.30936@germany.net> * [misc/winsock.c] Fixed bug in WSACleanup which lead to crashes in WINSOCK_HandleIO. * [graphics/fontengine.c] [include/font.h] Minor improvements. * [memory/global.c] Implemented GlobalEntryHandle. * [misc/toolhelp.c] Fixed a memory bug in Notify*register. * [misc/w32scomb.c] Improved Get16DLLAddress. * [objects/gdiobj.c] Implemented GdiSeeGdiDo. Sat Feb 14 14:57:39 1998 John Richardson <jrichard@zko.dec.com> * [win32/console.c] Added the console implementation, AllocConsole, FreeConsole, CONSOLE_InheritConsole. * [documentation/console] Some documentation on the console. * [include/winerror.h] Added some error defines. * [scheduler/k32obj.c] Registered the scheduler ops. Fri Feb 13 19:35:35 1998 James Moody <013263m@dragon.acadiau.ca> * [ole/ole2nls.c] Some English language fixes for missing values. * [controls/listbox.c] Fix to allow an empty listbox to deselect all items. * [relay32/user32.spec] [windows/keyboard.c] CreateAcceleratorTableA stub method. * [windows/sysmetrics.c] Added missing SM_CXCURSOR & SM_CYCURSOR initializers. * [windows/message.c] PostThreadMessage32A stub method. Fri Feb 13 17:12:24 1998 Jim Peterson <jspeter@roanoke.infi.net> * [libtest/hello3res.rc] [libtest/hello3.c] [libtest/Makefile.in] Updated the 'hello3' test so that it functions properly again. Fri Feb 13 14:08:07 1998 Martin Boehme <boehme@informatik.mu-luebeck.de> * [graphics/mapping.c] Fixed the embarrassing bugs I introduced into DPtoLP and LPtoDP. * [windows/scroll.c] Prevent ScrollWindow32 from sending WM_ERASEBKGND. Thu Feb 12 22:46:53 1998 Huw D M Davies <h.davies1@physics.oxford.ac.uk> * [objects/metafile] [include/ldt.h] Fix to cope with records longer than 64K. * [windows/clipboard.c] Clean up bitmaps and metapicts properly. Mon Feb 3 21:52:18 1998 Karl Backström <karl_b@geocities.com> * [programs/winhelp/Sw.rc] [resources/sysres_Sw.rc] Minor update of Swedish language support.
125 lines
3.1 KiB
C
125 lines
3.1 KiB
C
/*
|
|
* Emulator initialisation code
|
|
*
|
|
*/
|
|
|
|
#include <stdio.h>
|
|
#include "windows.h"
|
|
#include "callback.h"
|
|
#include "debugger.h"
|
|
#include "miscemu.h"
|
|
#include "module.h"
|
|
#include "options.h"
|
|
|
|
|
|
/***********************************************************************
|
|
* Emulator initialisation
|
|
*/
|
|
BOOL32 MAIN_EmulatorInit(void)
|
|
{
|
|
extern BOOL32 MAIN_KernelInit(void);
|
|
extern BOOL32 MAIN_UserInit(void);
|
|
extern BOOL32 WIN16DRV_Init(void);
|
|
extern BOOL32 RELAY_Init(void);
|
|
|
|
/* Initialize the kernel */
|
|
if (!MAIN_KernelInit()) return FALSE;
|
|
|
|
/* Initialize relay code */
|
|
if (!RELAY_Init()) return FALSE;
|
|
|
|
/* Initialize signal handling */
|
|
if (!SIGNAL_InitEmulator()) return FALSE;
|
|
|
|
/* Create the Win16 printer driver */
|
|
if (!WIN16DRV_Init()) return FALSE;
|
|
|
|
/* Initialize all the USER stuff */
|
|
return MAIN_UserInit();
|
|
}
|
|
|
|
|
|
/**********************************************************************
|
|
* main
|
|
*/
|
|
int main( int argc, char *argv[] )
|
|
{
|
|
extern BOOL32 PROCESS_Init(void);
|
|
extern BOOL32 MAIN_WineInit( int *argc, char *argv[] );
|
|
extern void *CALL32_Init(void);
|
|
extern char * DEBUG_argv0;
|
|
|
|
int i,loaded;
|
|
HINSTANCE32 handle;
|
|
|
|
__winelib = 0; /* First of all, clear the Winelib flag */
|
|
|
|
/*
|
|
* Save this so that the internal debugger can get a hold of it if
|
|
* it needs to.
|
|
*/
|
|
DEBUG_argv0 = argv[0];
|
|
|
|
/* Create the initial process */
|
|
if (!PROCESS_Init()) return FALSE;
|
|
|
|
/* Parse command-line */
|
|
if (!MAIN_WineInit( &argc, argv )) return 1;
|
|
|
|
/* Handle -dll option (hack) */
|
|
|
|
if (Options.dllFlags)
|
|
{
|
|
if (!BUILTIN_ParseDLLOptions( Options.dllFlags ))
|
|
{
|
|
fprintf( stderr, "%s: Syntax: -dll +xxx,... or -dll -xxx,...\n",
|
|
argv[0] );
|
|
BUILTIN_PrintDLLs();
|
|
exit(1);
|
|
}
|
|
}
|
|
|
|
/* Initialize everything */
|
|
|
|
if (!MAIN_EmulatorInit()) return 1;
|
|
|
|
/* Initialize CALL32 routines */
|
|
/* This needs to be done just before task-switching starts */
|
|
IF1632_CallLargeStack = (int (*)(int (*func)(), void *arg))CALL32_Init();
|
|
|
|
loaded=0;
|
|
for (i = 1; i < argc; i++)
|
|
{
|
|
if ((handle = WinExec32( argv[i], SW_SHOWNORMAL )) < 32)
|
|
{
|
|
fprintf(stderr, "wine: can't exec '%s': ", argv[i]);
|
|
switch (handle)
|
|
{
|
|
case 2: fprintf( stderr, "file not found\n" ); break;
|
|
case 11: fprintf( stderr, "invalid exe file\n" ); break;
|
|
case 21: fprintf( stderr, "win32 executable\n" ); break;
|
|
default: fprintf( stderr, "error=%d\n", handle ); break;
|
|
}
|
|
return 1;
|
|
}
|
|
loaded++;
|
|
}
|
|
|
|
if (!loaded) { /* nothing loaded */
|
|
extern void MAIN_Usage(char*);
|
|
MAIN_Usage(argv[0]);
|
|
return 1;
|
|
}
|
|
|
|
if (!GetNumTasks())
|
|
{
|
|
fprintf( stderr, "wine: no executable file found.\n" );
|
|
return 0;
|
|
}
|
|
|
|
if (Options.debug) DEBUG_AddModuleBreakpoints();
|
|
|
|
Yield16(); /* Start the first task */
|
|
fprintf( stderr, "WinMain: Should never happen: returned from Yield()\n" );
|
|
return 0;
|
|
}
|