mirror of
git://source.winehq.org/git/wine.git
synced 2024-09-18 10:26:33 +00:00
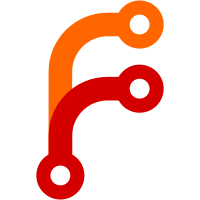
Pierre Mageau Don't update the combo box selection when closing the dialog only when clicking on OK button. Adjust file dialog size when help button isn't present. Don Kelly. Fixes problems with open dialog box filters. Ulrich Czekalla Prevents the help button from displaying on OpenFile dialogs unless the proper flag is set in the OPENFILENAME struct. Yuxi Zhang Fixed memory leak. Jean-Claude Batista Add tooltips to the file Dialog toolbar. Sylvain Bouchard, Bill Jin Three new functions EnumSelectedPidls, GetNumSelected, FILEDLG95_OnOpenUsingView: corrections Instead of passing in a copy of ofn, passing in the pointer of ofn. David Golding A "!" was missing in a check against lpstrInitialDir. Rick Mutzke Fixed crash: if dialog has no filetypes appearing in the dropdown list. Matt Robertson, Ulrich Czekalla Fixed problems occurring with selection of files inside openfiledlg.
97 lines
1.8 KiB
C
97 lines
1.8 KiB
C
/*
|
|
* COMMDLG - File Dialogs
|
|
*
|
|
* Copyright 1994 Martin Ayotte
|
|
* Copyright 1996 Albrecht Kleine
|
|
*/
|
|
|
|
#include <string.h>
|
|
|
|
#include "wine/winestring.h"
|
|
#include "winbase.h"
|
|
#include "commdlg.h"
|
|
#include "debugtools.h"
|
|
|
|
#include "heap.h" /* Has to go */
|
|
|
|
DEFAULT_DEBUG_CHANNEL(commdlg)
|
|
|
|
#include "cdlg.h"
|
|
|
|
/***********************************************************************
|
|
* GetFileTitleA (COMDLG32.8)
|
|
*
|
|
*/
|
|
short WINAPI GetFileTitleA(LPCSTR lpFile, LPSTR lpTitle, UINT cbBuf)
|
|
{
|
|
int i, len;
|
|
|
|
TRACE("(%p %p %d); \n", lpFile, lpTitle, cbBuf);
|
|
|
|
if(lpFile == NULL || lpTitle == NULL)
|
|
return -1;
|
|
|
|
len = strlen(lpFile);
|
|
|
|
if (len == 0)
|
|
return -1;
|
|
|
|
if(strpbrk(lpFile, "*[]"))
|
|
return -1;
|
|
|
|
len--;
|
|
|
|
if(lpFile[len] == '/' || lpFile[len] == '\\' || lpFile[len] == ':')
|
|
return -1;
|
|
|
|
for(i = len; i >= 0; i--)
|
|
{
|
|
if (lpFile[i] == '/' || lpFile[i] == '\\' || lpFile[i] == ':')
|
|
{
|
|
i++;
|
|
break;
|
|
}
|
|
}
|
|
|
|
if(i == -1)
|
|
i++;
|
|
|
|
TRACE("---> '%s' \n", &lpFile[i]);
|
|
|
|
len = strlen(lpFile+i)+1;
|
|
if(cbBuf < len)
|
|
return len;
|
|
|
|
strncpy(lpTitle, &lpFile[i], len);
|
|
return 0;
|
|
}
|
|
|
|
|
|
/***********************************************************************
|
|
* GetFileTitleW (COMDLG32.9)
|
|
*
|
|
*/
|
|
short WINAPI GetFileTitleW(LPCWSTR lpFile, LPWSTR lpTitle, UINT cbBuf)
|
|
{
|
|
LPSTR file = HEAP_strdupWtoA(GetProcessHeap(), 0, lpFile); /* Has to go */
|
|
LPSTR title = HeapAlloc(GetProcessHeap(), 0, cbBuf);
|
|
short ret;
|
|
|
|
ret = GetFileTitleA(file, title, cbBuf);
|
|
|
|
lstrcpynAtoW(lpTitle, title, cbBuf);
|
|
HeapFree(GetProcessHeap(), 0, file);
|
|
HeapFree(GetProcessHeap(), 0, title);
|
|
return ret;
|
|
}
|
|
|
|
|
|
/***********************************************************************
|
|
* GetFileTitle16 (COMMDLG.27)
|
|
*/
|
|
short WINAPI GetFileTitle16(LPCSTR lpFile, LPSTR lpTitle, UINT16 cbBuf)
|
|
{
|
|
return GetFileTitleA(lpFile, lpTitle, cbBuf);
|
|
}
|
|
|