mirror of
git://source.winehq.org/git/wine.git
synced 2024-09-16 06:06:50 +00:00
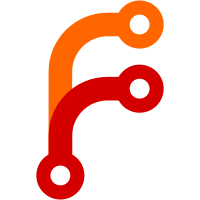
data to be exchanged. Split request and reply structures to make backwards compatibility easier. Moved many console functions to dlls/kernel, added code page support, changed a few requests to behave properly with the new protocol.
1787 lines
57 KiB
C
1787 lines
57 KiB
C
/* -*- C -*-
|
|
*
|
|
* Wine server protocol definition
|
|
*
|
|
* Copyright (C) 2001 Alexandre Julliard
|
|
*
|
|
* This file is used by tools/make_requests to build the
|
|
* protocol structures in include/wine/server_protocol.h
|
|
*/
|
|
|
|
@HEADER /* start of C declarations */
|
|
|
|
#include <stdlib.h>
|
|
#include <time.h>
|
|
#include "winbase.h"
|
|
|
|
struct request_header
|
|
{
|
|
int req; /* request code */
|
|
size_t request_size; /* request variable part size */
|
|
size_t reply_size; /* reply variable part maximum size */
|
|
};
|
|
|
|
struct reply_header
|
|
{
|
|
unsigned int error; /* error result */
|
|
size_t reply_size; /* reply variable part size */
|
|
};
|
|
|
|
/* placeholder structure for the maximum allowed request size */
|
|
/* this is used to construct the generic_request union */
|
|
struct request_max_size
|
|
{
|
|
int pad[16]; /* the max request size is 16 ints */
|
|
};
|
|
|
|
/* max size of the variable part of a request */
|
|
#define REQUEST_MAX_VAR_SIZE 1024
|
|
|
|
typedef int handle_t;
|
|
typedef unsigned short atom_t;
|
|
typedef unsigned int user_handle_t;
|
|
|
|
#define FIRST_USER_HANDLE 0x0020 /* first possible value for low word of user handle */
|
|
#define LAST_USER_HANDLE 0xffef /* last possible value for low word of user handle */
|
|
|
|
|
|
/* definitions of the event data depending on the event code */
|
|
struct debug_event_exception
|
|
{
|
|
EXCEPTION_RECORD record; /* exception record */
|
|
int first; /* first chance exception? */
|
|
};
|
|
struct debug_event_create_thread
|
|
{
|
|
handle_t handle; /* handle to the new thread */
|
|
void *teb; /* thread teb (in debugged process address space) */
|
|
void *start; /* thread startup routine */
|
|
};
|
|
struct debug_event_create_process
|
|
{
|
|
handle_t file; /* handle to the process exe file */
|
|
handle_t process; /* handle to the new process */
|
|
handle_t thread; /* handle to the new thread */
|
|
void *base; /* base of executable image */
|
|
int dbg_offset; /* offset of debug info in file */
|
|
int dbg_size; /* size of debug info */
|
|
void *teb; /* thread teb (in debugged process address space) */
|
|
void *start; /* thread startup routine */
|
|
void *name; /* image name (optional) */
|
|
int unicode; /* is it Unicode? */
|
|
};
|
|
struct debug_event_exit
|
|
{
|
|
int exit_code; /* thread or process exit code */
|
|
};
|
|
struct debug_event_load_dll
|
|
{
|
|
handle_t handle; /* file handle for the dll */
|
|
void *base; /* base address of the dll */
|
|
int dbg_offset; /* offset of debug info in file */
|
|
int dbg_size; /* size of debug info */
|
|
void *name; /* image name (optional) */
|
|
int unicode; /* is it Unicode? */
|
|
};
|
|
struct debug_event_unload_dll
|
|
{
|
|
void *base; /* base address of the dll */
|
|
};
|
|
struct debug_event_output_string
|
|
{
|
|
void *string; /* string to display (in debugged process address space) */
|
|
int unicode; /* is it Unicode? */
|
|
int length; /* string length */
|
|
};
|
|
struct debug_event_rip_info
|
|
{
|
|
int error; /* ??? */
|
|
int type; /* ??? */
|
|
};
|
|
union debug_event_data
|
|
{
|
|
struct debug_event_exception exception;
|
|
struct debug_event_create_thread create_thread;
|
|
struct debug_event_create_process create_process;
|
|
struct debug_event_exit exit;
|
|
struct debug_event_load_dll load_dll;
|
|
struct debug_event_unload_dll unload_dll;
|
|
struct debug_event_output_string output_string;
|
|
struct debug_event_rip_info rip_info;
|
|
};
|
|
|
|
/* debug event data */
|
|
typedef struct
|
|
{
|
|
int code; /* event code */
|
|
union debug_event_data info; /* event information */
|
|
} debug_event_t;
|
|
|
|
/* structure used in sending an fd from client to server */
|
|
struct send_fd
|
|
{
|
|
void *tid; /* thread id */
|
|
int fd; /* file descriptor on client-side */
|
|
};
|
|
|
|
/* structure sent by the server on the wait fifo */
|
|
struct wake_up_reply
|
|
{
|
|
void *cookie; /* magic cookie that was passed in select_request */
|
|
int signaled; /* wait result */
|
|
};
|
|
|
|
/* structure returned in the list of window properties */
|
|
typedef struct
|
|
{
|
|
atom_t atom; /* property atom */
|
|
short string; /* was atom a string originally? */
|
|
handle_t handle; /* handle stored in property */
|
|
} property_data_t;
|
|
|
|
/* structure to specify window rectangles */
|
|
typedef struct
|
|
{
|
|
int left;
|
|
int top;
|
|
int right;
|
|
int bottom;
|
|
} rectangle_t;
|
|
|
|
/* structure for console char/attribute info */
|
|
typedef struct
|
|
{
|
|
WCHAR ch;
|
|
unsigned short attr;
|
|
} char_info_t;
|
|
|
|
/****************************************************************/
|
|
/* Request declarations */
|
|
|
|
/* Create a new process from the context of the parent */
|
|
@REQ(new_process)
|
|
int inherit_all; /* inherit all handles from parent */
|
|
int create_flags; /* creation flags */
|
|
int start_flags; /* flags from startup info */
|
|
handle_t exe_file; /* file handle for main exe */
|
|
handle_t hstdin; /* handle for stdin */
|
|
handle_t hstdout; /* handle for stdout */
|
|
handle_t hstderr; /* handle for stderr */
|
|
int cmd_show; /* main window show mode */
|
|
VARARG(filename,string); /* file name of main exe */
|
|
@REPLY
|
|
handle_t info; /* new process info handle */
|
|
@END
|
|
|
|
|
|
/* Retrieve information about a newly started process */
|
|
@REQ(get_new_process_info)
|
|
handle_t info; /* info handle returned from new_process_request */
|
|
int pinherit; /* process handle inherit flag */
|
|
int tinherit; /* thread handle inherit flag */
|
|
@REPLY
|
|
void* pid; /* process id */
|
|
handle_t phandle; /* process handle (in the current process) */
|
|
void* tid; /* thread id */
|
|
handle_t thandle; /* thread handle (in the current process) */
|
|
handle_t event; /* event handle to signal startup */
|
|
@END
|
|
|
|
|
|
/* Create a new thread from the context of the parent */
|
|
@REQ(new_thread)
|
|
int suspend; /* new thread should be suspended on creation */
|
|
int inherit; /* inherit flag */
|
|
int request_fd; /* fd for request pipe */
|
|
@REPLY
|
|
void* tid; /* thread id */
|
|
handle_t handle; /* thread handle (in the current process) */
|
|
@END
|
|
|
|
|
|
/* Signal that we are finished booting on the client side */
|
|
@REQ(boot_done)
|
|
int debug_level; /* new debug level */
|
|
@END
|
|
|
|
|
|
/* Initialize a process; called from the new process context */
|
|
@REQ(init_process)
|
|
void* ldt_copy; /* addr of LDT copy */
|
|
int ppid; /* parent Unix pid */
|
|
@REPLY
|
|
int create_flags; /* creation flags */
|
|
int start_flags; /* flags from startup info */
|
|
unsigned int server_start; /* server start time (GetTickCount) */
|
|
handle_t exe_file; /* file handle for main exe */
|
|
handle_t hstdin; /* handle for stdin */
|
|
handle_t hstdout; /* handle for stdout */
|
|
handle_t hstderr; /* handle for stderr */
|
|
int cmd_show; /* main window show mode */
|
|
VARARG(filename,string); /* file name of main exe */
|
|
@END
|
|
|
|
|
|
/* Signal the end of the process initialization */
|
|
@REQ(init_process_done)
|
|
void* module; /* main module base address */
|
|
void* entry; /* process entry point */
|
|
void* name; /* ptr to ptr to name (in process addr space) */
|
|
handle_t exe_file; /* file handle for main exe */
|
|
int gui; /* is it a GUI process? */
|
|
@REPLY
|
|
int debugged; /* being debugged? */
|
|
@END
|
|
|
|
|
|
/* Initialize a thread; called from the child after fork()/clone() */
|
|
@REQ(init_thread)
|
|
int unix_pid; /* Unix pid of new thread */
|
|
void* teb; /* TEB of new thread (in thread address space) */
|
|
void* entry; /* thread entry point (in thread address space) */
|
|
int reply_fd; /* fd for reply pipe */
|
|
int wait_fd; /* fd for blocking calls pipe */
|
|
@REPLY
|
|
void* pid; /* process id of the new thread's process */
|
|
void* tid; /* thread id of the new thread */
|
|
int boot; /* is this the boot thread? */
|
|
int version; /* protocol version */
|
|
@END
|
|
|
|
|
|
/* Terminate a process */
|
|
@REQ(terminate_process)
|
|
handle_t handle; /* process handle to terminate */
|
|
int exit_code; /* process exit code */
|
|
@REPLY
|
|
int self; /* suicide? */
|
|
@END
|
|
|
|
|
|
/* Terminate a thread */
|
|
@REQ(terminate_thread)
|
|
handle_t handle; /* thread handle to terminate */
|
|
int exit_code; /* thread exit code */
|
|
@REPLY
|
|
int self; /* suicide? */
|
|
int last; /* last thread in this process? */
|
|
@END
|
|
|
|
|
|
/* Retrieve information about a process */
|
|
@REQ(get_process_info)
|
|
handle_t handle; /* process handle */
|
|
@REPLY
|
|
void* pid; /* server process id */
|
|
int debugged; /* debugged? */
|
|
int exit_code; /* process exit code */
|
|
int priority; /* priority class */
|
|
int process_affinity; /* process affinity mask */
|
|
int system_affinity; /* system affinity mask */
|
|
@END
|
|
|
|
|
|
/* Set a process informations */
|
|
@REQ(set_process_info)
|
|
handle_t handle; /* process handle */
|
|
int mask; /* setting mask (see below) */
|
|
int priority; /* priority class */
|
|
int affinity; /* affinity mask */
|
|
@END
|
|
#define SET_PROCESS_INFO_PRIORITY 0x01
|
|
#define SET_PROCESS_INFO_AFFINITY 0x02
|
|
|
|
|
|
/* Retrieve information about a thread */
|
|
@REQ(get_thread_info)
|
|
handle_t handle; /* thread handle */
|
|
void* tid_in; /* thread id (optional) */
|
|
@REPLY
|
|
void* tid; /* server thread id */
|
|
void* teb; /* thread teb pointer */
|
|
int exit_code; /* thread exit code */
|
|
int priority; /* thread priority level */
|
|
@END
|
|
|
|
|
|
/* Set a thread informations */
|
|
@REQ(set_thread_info)
|
|
handle_t handle; /* thread handle */
|
|
int mask; /* setting mask (see below) */
|
|
int priority; /* priority class */
|
|
int affinity; /* affinity mask */
|
|
@END
|
|
#define SET_THREAD_INFO_PRIORITY 0x01
|
|
#define SET_THREAD_INFO_AFFINITY 0x02
|
|
|
|
|
|
/* Suspend a thread */
|
|
@REQ(suspend_thread)
|
|
handle_t handle; /* thread handle */
|
|
@REPLY
|
|
int count; /* new suspend count */
|
|
@END
|
|
|
|
|
|
/* Resume a thread */
|
|
@REQ(resume_thread)
|
|
handle_t handle; /* thread handle */
|
|
@REPLY
|
|
int count; /* new suspend count */
|
|
@END
|
|
|
|
|
|
/* Notify the server that a dll has been loaded */
|
|
@REQ(load_dll)
|
|
handle_t handle; /* file handle */
|
|
void* base; /* base address */
|
|
int dbg_offset; /* debug info offset */
|
|
int dbg_size; /* debug info size */
|
|
void* name; /* ptr to ptr to name (in process addr space) */
|
|
@END
|
|
|
|
|
|
/* Notify the server that a dll is being unloaded */
|
|
@REQ(unload_dll)
|
|
void* base; /* base address */
|
|
@END
|
|
|
|
|
|
/* Queue an APC for a thread */
|
|
@REQ(queue_apc)
|
|
handle_t handle; /* thread handle */
|
|
int user; /* user or system apc? */
|
|
void* func; /* function to call */
|
|
void* param; /* param for function to call */
|
|
@END
|
|
|
|
|
|
/* Get next APC to call */
|
|
@REQ(get_apc)
|
|
int alertable; /* is thread alertable? */
|
|
@REPLY
|
|
void* func; /* function to call */
|
|
int type; /* function type */
|
|
VARARG(args,ptrs); /* function arguments */
|
|
@END
|
|
enum apc_type { APC_NONE, APC_USER, APC_TIMER, APC_ASYNC };
|
|
|
|
|
|
/* Close a handle for the current process */
|
|
@REQ(close_handle)
|
|
handle_t handle; /* handle to close */
|
|
@REPLY
|
|
int fd; /* associated fd to close */
|
|
@END
|
|
|
|
|
|
/* Set a handle information */
|
|
@REQ(set_handle_info)
|
|
handle_t handle; /* handle we are interested in */
|
|
int flags; /* new handle flags */
|
|
int mask; /* mask for flags to set */
|
|
int fd; /* file descriptor or -1 */
|
|
@REPLY
|
|
int old_flags; /* old flag value */
|
|
int cur_fd; /* current file descriptor */
|
|
@END
|
|
|
|
|
|
/* Duplicate a handle */
|
|
@REQ(dup_handle)
|
|
handle_t src_process; /* src process handle */
|
|
handle_t src_handle; /* src handle to duplicate */
|
|
handle_t dst_process; /* dst process handle */
|
|
unsigned int access; /* wanted access rights */
|
|
int inherit; /* inherit flag */
|
|
int options; /* duplicate options (see below) */
|
|
@REPLY
|
|
handle_t handle; /* duplicated handle in dst process */
|
|
int fd; /* associated fd to close */
|
|
@END
|
|
#define DUP_HANDLE_CLOSE_SOURCE DUPLICATE_CLOSE_SOURCE
|
|
#define DUP_HANDLE_SAME_ACCESS DUPLICATE_SAME_ACCESS
|
|
#define DUP_HANDLE_MAKE_GLOBAL 0x80000000 /* Not a Windows flag */
|
|
|
|
|
|
/* Open a handle to a process */
|
|
@REQ(open_process)
|
|
void* pid; /* process id to open */
|
|
unsigned int access; /* wanted access rights */
|
|
int inherit; /* inherit flag */
|
|
@REPLY
|
|
handle_t handle; /* handle to the process */
|
|
@END
|
|
|
|
|
|
/* Wait for handles */
|
|
@REQ(select)
|
|
int flags; /* wait flags (see below) */
|
|
void* cookie; /* magic cookie to return to client */
|
|
int sec; /* absolute timeout */
|
|
int usec; /* absolute timeout */
|
|
VARARG(handles,handles); /* handles to select on */
|
|
@END
|
|
#define SELECT_ALL 1
|
|
#define SELECT_ALERTABLE 2
|
|
#define SELECT_INTERRUPTIBLE 4
|
|
#define SELECT_TIMEOUT 8
|
|
|
|
|
|
/* Create an event */
|
|
@REQ(create_event)
|
|
int manual_reset; /* manual reset event */
|
|
int initial_state; /* initial state of the event */
|
|
int inherit; /* inherit flag */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
handle_t handle; /* handle to the event */
|
|
@END
|
|
|
|
/* Event operation */
|
|
@REQ(event_op)
|
|
handle_t handle; /* handle to event */
|
|
int op; /* event operation (see below) */
|
|
@END
|
|
enum event_op { PULSE_EVENT, SET_EVENT, RESET_EVENT };
|
|
|
|
|
|
/* Open an event */
|
|
@REQ(open_event)
|
|
unsigned int access; /* wanted access rights */
|
|
int inherit; /* inherit flag */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
handle_t handle; /* handle to the event */
|
|
@END
|
|
|
|
|
|
/* Create a mutex */
|
|
@REQ(create_mutex)
|
|
int owned; /* initially owned? */
|
|
int inherit; /* inherit flag */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
handle_t handle; /* handle to the mutex */
|
|
@END
|
|
|
|
|
|
/* Release a mutex */
|
|
@REQ(release_mutex)
|
|
handle_t handle; /* handle to the mutex */
|
|
@END
|
|
|
|
|
|
/* Open a mutex */
|
|
@REQ(open_mutex)
|
|
unsigned int access; /* wanted access rights */
|
|
int inherit; /* inherit flag */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
handle_t handle; /* handle to the mutex */
|
|
@END
|
|
|
|
|
|
/* Create a semaphore */
|
|
@REQ(create_semaphore)
|
|
unsigned int initial; /* initial count */
|
|
unsigned int max; /* maximum count */
|
|
int inherit; /* inherit flag */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
handle_t handle; /* handle to the semaphore */
|
|
@END
|
|
|
|
|
|
/* Release a semaphore */
|
|
@REQ(release_semaphore)
|
|
handle_t handle; /* handle to the semaphore */
|
|
unsigned int count; /* count to add to semaphore */
|
|
@REPLY
|
|
unsigned int prev_count; /* previous semaphore count */
|
|
@END
|
|
|
|
|
|
/* Open a semaphore */
|
|
@REQ(open_semaphore)
|
|
unsigned int access; /* wanted access rights */
|
|
int inherit; /* inherit flag */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
handle_t handle; /* handle to the semaphore */
|
|
@END
|
|
|
|
|
|
/* Create a file */
|
|
@REQ(create_file)
|
|
unsigned int access; /* wanted access rights */
|
|
int inherit; /* inherit flag */
|
|
unsigned int sharing; /* sharing flags */
|
|
int create; /* file create action */
|
|
unsigned int attrs; /* file attributes for creation */
|
|
int drive_type; /* type of drive the file is on */
|
|
VARARG(filename,string); /* file name */
|
|
@REPLY
|
|
handle_t handle; /* handle to the file */
|
|
@END
|
|
|
|
|
|
/* Allocate a file handle for a Unix fd */
|
|
@REQ(alloc_file_handle)
|
|
unsigned int access; /* wanted access rights */
|
|
int inherit; /* inherit flag */
|
|
int fd; /* file descriptor on the client side */
|
|
@REPLY
|
|
handle_t handle; /* handle to the file */
|
|
@END
|
|
|
|
|
|
/* Get a Unix fd to access a file */
|
|
@REQ(get_handle_fd)
|
|
handle_t handle; /* handle to the file */
|
|
unsigned int access; /* wanted access rights */
|
|
@REPLY
|
|
int fd; /* file descriptor */
|
|
int type; /* the type of file */
|
|
@END
|
|
#define FD_TYPE_INVALID 0
|
|
#define FD_TYPE_DEFAULT 1
|
|
#define FD_TYPE_CONSOLE 2
|
|
#define FD_TYPE_OVERLAPPED 3
|
|
#define FD_TYPE_TIMEOUT 4
|
|
|
|
|
|
/* Set a file current position */
|
|
@REQ(set_file_pointer)
|
|
handle_t handle; /* handle to the file */
|
|
int low; /* position low word */
|
|
int high; /* position high word */
|
|
int whence; /* whence to seek */
|
|
@REPLY
|
|
int new_low; /* new position low word */
|
|
int new_high; /* new position high word */
|
|
@END
|
|
|
|
|
|
/* Truncate (or extend) a file */
|
|
@REQ(truncate_file)
|
|
handle_t handle; /* handle to the file */
|
|
@END
|
|
|
|
|
|
/* Set a file access and modification times */
|
|
@REQ(set_file_time)
|
|
handle_t handle; /* handle to the file */
|
|
time_t access_time; /* last access time */
|
|
time_t write_time; /* last write time */
|
|
@END
|
|
|
|
|
|
/* Flush a file buffers */
|
|
@REQ(flush_file)
|
|
handle_t handle; /* handle to the file */
|
|
@END
|
|
|
|
|
|
/* Get information about a file */
|
|
@REQ(get_file_info)
|
|
handle_t handle; /* handle to the file */
|
|
@REPLY
|
|
int type; /* file type */
|
|
int attr; /* file attributes */
|
|
time_t access_time; /* last access time */
|
|
time_t write_time; /* last write time */
|
|
int size_high; /* file size */
|
|
int size_low; /* file size */
|
|
int links; /* number of links */
|
|
int index_high; /* unique index */
|
|
int index_low; /* unique index */
|
|
unsigned int serial; /* volume serial number */
|
|
@END
|
|
|
|
|
|
/* Lock a region of a file */
|
|
@REQ(lock_file)
|
|
handle_t handle; /* handle to the file */
|
|
unsigned int offset_low; /* offset of start of lock */
|
|
unsigned int offset_high; /* offset of start of lock */
|
|
unsigned int count_low; /* count of bytes to lock */
|
|
unsigned int count_high; /* count of bytes to lock */
|
|
@END
|
|
|
|
|
|
/* Unlock a region of a file */
|
|
@REQ(unlock_file)
|
|
handle_t handle; /* handle to the file */
|
|
unsigned int offset_low; /* offset of start of unlock */
|
|
unsigned int offset_high; /* offset of start of unlock */
|
|
unsigned int count_low; /* count of bytes to unlock */
|
|
unsigned int count_high; /* count of bytes to unlock */
|
|
@END
|
|
|
|
|
|
/* Create an anonymous pipe */
|
|
@REQ(create_pipe)
|
|
int inherit; /* inherit flag */
|
|
@REPLY
|
|
handle_t handle_read; /* handle to the read-side of the pipe */
|
|
handle_t handle_write; /* handle to the write-side of the pipe */
|
|
@END
|
|
|
|
|
|
/* Create a socket */
|
|
@REQ(create_socket)
|
|
unsigned int access; /* wanted access rights */
|
|
int inherit; /* inherit flag */
|
|
int family; /* family, see socket manpage */
|
|
int type; /* type, see socket manpage */
|
|
int protocol; /* protocol, see socket manpage */
|
|
@REPLY
|
|
handle_t handle; /* handle to the new socket */
|
|
@END
|
|
|
|
|
|
/* Accept a socket */
|
|
@REQ(accept_socket)
|
|
handle_t lhandle; /* handle to the listening socket */
|
|
unsigned int access; /* wanted access rights */
|
|
int inherit; /* inherit flag */
|
|
@REPLY
|
|
handle_t handle; /* handle to the new socket */
|
|
@END
|
|
|
|
|
|
/* Set socket event parameters */
|
|
@REQ(set_socket_event)
|
|
handle_t handle; /* handle to the socket */
|
|
unsigned int mask; /* event mask */
|
|
handle_t event; /* event object */
|
|
@END
|
|
|
|
|
|
/* Get socket event parameters */
|
|
@REQ(get_socket_event)
|
|
handle_t handle; /* handle to the socket */
|
|
int service; /* clear pending? */
|
|
handle_t s_event; /* "expected" event object */
|
|
handle_t c_event; /* event to clear */
|
|
@REPLY
|
|
unsigned int mask; /* event mask */
|
|
unsigned int pmask; /* pending events */
|
|
unsigned int state; /* status bits */
|
|
VARARG(errors,ints); /* event errors */
|
|
@END
|
|
|
|
|
|
/* Reenable pending socket events */
|
|
@REQ(enable_socket_event)
|
|
handle_t handle; /* handle to the socket */
|
|
unsigned int mask; /* events to re-enable */
|
|
unsigned int sstate; /* status bits to set */
|
|
unsigned int cstate; /* status bits to clear */
|
|
@END
|
|
|
|
|
|
/* Allocate a console (only used by a console renderer) */
|
|
@REQ(alloc_console)
|
|
unsigned int access; /* wanted access rights */
|
|
int inherit; /* inherit flag */
|
|
void* pid; /* pid of process which shall be attached to the console */
|
|
@REPLY
|
|
handle_t handle_in; /* handle to console input */
|
|
handle_t event; /* handle to renderer events change notification */
|
|
@END
|
|
|
|
|
|
/* Free the console of the current process */
|
|
@REQ(free_console)
|
|
@END
|
|
|
|
|
|
#define CONSOLE_RENDERER_NONE_EVENT 0x00
|
|
#define CONSOLE_RENDERER_TITLE_EVENT 0x01
|
|
#define CONSOLE_RENDERER_ACTIVE_SB_EVENT 0x02
|
|
#define CONSOLE_RENDERER_SB_RESIZE_EVENT 0x03
|
|
#define CONSOLE_RENDERER_UPDATE_EVENT 0x04
|
|
#define CONSOLE_RENDERER_CURSOR_POS_EVENT 0x05
|
|
#define CONSOLE_RENDERER_CURSOR_GEOM_EVENT 0x06
|
|
#define CONSOLE_RENDERER_DISPLAY_EVENT 0x07
|
|
#define CONSOLE_RENDERER_EXIT_EVENT 0x08
|
|
struct console_renderer_event
|
|
{
|
|
short event;
|
|
union
|
|
{
|
|
struct update
|
|
{
|
|
short top;
|
|
short bottom;
|
|
} update;
|
|
struct resize
|
|
{
|
|
short width;
|
|
short height;
|
|
} resize;
|
|
struct cursor_pos
|
|
{
|
|
short x;
|
|
short y;
|
|
} cursor_pos;
|
|
struct cursor_geom
|
|
{
|
|
short visible;
|
|
short size;
|
|
} cursor_geom;
|
|
struct display
|
|
{
|
|
short left;
|
|
short top;
|
|
short width;
|
|
short height;
|
|
} display;
|
|
} u;
|
|
};
|
|
|
|
/* retrieve console events for the renderer */
|
|
@REQ(get_console_renderer_events)
|
|
handle_t handle; /* handle to console input events */
|
|
@REPLY
|
|
VARARG(data,bytes); /* the various console_renderer_events */
|
|
@END
|
|
|
|
|
|
/* Open a handle to the process console */
|
|
@REQ(open_console)
|
|
int from; /* 0 (resp 1) input (resp output) of current process console */
|
|
/* otherwise console_in handle to get active screen buffer? */
|
|
unsigned int access; /* wanted access rights */
|
|
int inherit; /* inherit flag */
|
|
int share; /* share mask (only for output handles) */
|
|
@REPLY
|
|
handle_t handle; /* handle to the console */
|
|
@END
|
|
|
|
|
|
/* Get a console mode (input or output) */
|
|
@REQ(get_console_mode)
|
|
handle_t handle; /* handle to the console */
|
|
@REPLY
|
|
int mode; /* console mode */
|
|
@END
|
|
|
|
|
|
/* Set a console mode (input or output) */
|
|
@REQ(set_console_mode)
|
|
handle_t handle; /* handle to the console */
|
|
int mode; /* console mode */
|
|
@END
|
|
|
|
|
|
/* Set info about a console (input only) */
|
|
@REQ(set_console_input_info)
|
|
handle_t handle; /* handle to console input, or 0 for process' console */
|
|
int mask; /* setting mask (see below) */
|
|
handle_t active_sb; /* active screen buffer */
|
|
int history_mode; /* whether we duplicate lines in history */
|
|
int history_size; /* number of lines in history */
|
|
VARARG(title,unicode_str); /* console title */
|
|
@END
|
|
#define SET_CONSOLE_INPUT_INFO_ACTIVE_SB 0x01
|
|
#define SET_CONSOLE_INPUT_INFO_TITLE 0x02
|
|
#define SET_CONSOLE_INPUT_INFO_HISTORY_MODE 0x04
|
|
#define SET_CONSOLE_INPUT_INFO_HISTORY_SIZE 0x08
|
|
|
|
|
|
/* Get info about a console (input only) */
|
|
@REQ(get_console_input_info)
|
|
handle_t handle; /* handle to console input, or 0 for process' console */
|
|
@REPLY
|
|
int history_mode; /* whether we duplicate lines in history */
|
|
int history_size; /* number of lines in history */
|
|
int history_index; /* number of used lines in history */
|
|
VARARG(title,unicode_str); /* console title */
|
|
@END
|
|
|
|
|
|
/* appends a string to console's history */
|
|
@REQ(append_console_input_history)
|
|
handle_t handle; /* handle to console input, or 0 for process' console */
|
|
VARARG(line,unicode_str); /* line to add */
|
|
@END
|
|
|
|
|
|
/* appends a string to console's history */
|
|
@REQ(get_console_input_history)
|
|
handle_t handle; /* handle to console input, or 0 for process' console */
|
|
int index; /* index to get line from */
|
|
@REPLY
|
|
int total; /* total length of line in Unicode chars */
|
|
VARARG(line,unicode_str); /* line to add */
|
|
@END
|
|
|
|
|
|
/* creates a new screen buffer on process' console */
|
|
@REQ(create_console_output)
|
|
handle_t handle_in; /* handle to console input, or 0 for process' console */
|
|
int access; /* wanted access rights */
|
|
int share; /* sharing credentials */
|
|
int inherit; /* inherit flag */
|
|
@REPLY
|
|
handle_t handle_out; /* handle to the screen buffer */
|
|
@END
|
|
|
|
|
|
/* Set info about a console (output only) */
|
|
@REQ(set_console_output_info)
|
|
handle_t handle; /* handle to the console */
|
|
int mask; /* setting mask (see below) */
|
|
short int cursor_size; /* size of cursor (percentage filled) */
|
|
short int cursor_visible;/* cursor visibility flag */
|
|
short int cursor_x; /* position of cursor (x, y) */
|
|
short int cursor_y;
|
|
short int width; /* width of the screen buffer */
|
|
short int height; /* height of the screen buffer */
|
|
short int attr; /* default attribute */
|
|
short int win_left; /* window actually displayed by renderer */
|
|
short int win_top; /* the rect area is expressed withing the */
|
|
short int win_right; /* boundaries of the screen buffer */
|
|
short int win_bottom;
|
|
short int max_width; /* maximum size (width x height) for the window */
|
|
short int max_height;
|
|
@END
|
|
#define SET_CONSOLE_OUTPUT_INFO_CURSOR_GEOM 0x01
|
|
#define SET_CONSOLE_OUTPUT_INFO_CURSOR_POS 0x02
|
|
#define SET_CONSOLE_OUTPUT_INFO_SIZE 0x04
|
|
#define SET_CONSOLE_OUTPUT_INFO_ATTR 0x08
|
|
#define SET_CONSOLE_OUTPUT_INFO_DISPLAY_WINDOW 0x10
|
|
#define SET_CONSOLE_OUTPUT_INFO_MAX_SIZE 0x20
|
|
|
|
|
|
/* Get info about a console (output only) */
|
|
@REQ(get_console_output_info)
|
|
handle_t handle; /* handle to the console */
|
|
@REPLY
|
|
short int cursor_size; /* size of cursor (percentage filled) */
|
|
short int cursor_visible;/* cursor visibility flag */
|
|
short int cursor_x; /* position of cursor (x, y) */
|
|
short int cursor_y;
|
|
short int width; /* width of the screen buffer */
|
|
short int height; /* height of the screen buffer */
|
|
short int attr; /* default attribute */
|
|
short int win_left; /* window actually displayed by renderer */
|
|
short int win_top; /* the rect area is expressed withing the */
|
|
short int win_right; /* boundaries of the screen buffer */
|
|
short int win_bottom;
|
|
short int max_width; /* maximum size (width x height) for the window */
|
|
short int max_height;
|
|
@END
|
|
|
|
/* Add input records to a console input queue */
|
|
@REQ(write_console_input)
|
|
handle_t handle; /* handle to the console input */
|
|
VARARG(rec,input_records); /* input records */
|
|
@REPLY
|
|
int written; /* number of records written */
|
|
@END
|
|
|
|
|
|
/* Fetch input records from a console input queue */
|
|
@REQ(read_console_input)
|
|
handle_t handle; /* handle to the console input */
|
|
int flush; /* flush the retrieved records from the queue? */
|
|
@REPLY
|
|
int read; /* number of records read */
|
|
VARARG(rec,input_records); /* input records */
|
|
@END
|
|
|
|
|
|
/* write data (chars and/or attributes) in a screen buffer */
|
|
@REQ(write_console_output)
|
|
handle_t handle; /* handle to the console output */
|
|
int x; /* position where to start writing */
|
|
int y;
|
|
int mode; /* char info (see below) */
|
|
int wrap; /* wrap around at end of line? */
|
|
VARARG(data,bytes); /* info to write */
|
|
@REPLY
|
|
int written; /* number of char infos actually written */
|
|
int width; /* width of screen buffer */
|
|
int height; /* height of screen buffer */
|
|
@END
|
|
enum char_info_mode
|
|
{
|
|
CHAR_INFO_MODE_TEXT, /* characters only */
|
|
CHAR_INFO_MODE_ATTR, /* attributes only */
|
|
CHAR_INFO_MODE_TEXTATTR, /* both characters and attributes */
|
|
CHAR_INFO_MODE_TEXTSTDATTR /* characters but use standard attributes */
|
|
};
|
|
|
|
|
|
/* fill a screen buffer with constant data (chars and/or attributes) */
|
|
@REQ(fill_console_output)
|
|
handle_t handle; /* handle to the console output */
|
|
int x; /* position where to start writing */
|
|
int y;
|
|
int mode; /* char info mode */
|
|
int count; /* number to write */
|
|
int wrap; /* wrap around at end of line? */
|
|
char_info_t data; /* data to write */
|
|
@REPLY
|
|
int written; /* number of char infos actually written */
|
|
@END
|
|
|
|
|
|
/* read data (chars and/or attributes) from a screen buffer */
|
|
@REQ(read_console_output)
|
|
handle_t handle; /* handle to the console output */
|
|
int x; /* position (x,y) where to start reading */
|
|
int y;
|
|
int mode; /* char info mode */
|
|
int wrap; /* wrap around at end of line? */
|
|
@REPLY
|
|
int width; /* width of screen buffer */
|
|
int height; /* height of screen buffer */
|
|
VARARG(data,bytes);
|
|
@END
|
|
|
|
/* move a rect (of data) in screen buffer content */
|
|
@REQ(move_console_output)
|
|
handle_t handle; /* handle to the console output */
|
|
short int x_src; /* position (x, y) of rect to start moving from */
|
|
short int y_src;
|
|
short int x_dst; /* position (x, y) of rect to move to */
|
|
short int y_dst;
|
|
short int w; /* size of the rect (width, height) to move */
|
|
short int h;
|
|
@END
|
|
|
|
|
|
/* Create a change notification */
|
|
@REQ(create_change_notification)
|
|
int subtree; /* watch all the subtree */
|
|
int filter; /* notification filter */
|
|
@REPLY
|
|
handle_t handle; /* handle to the change notification */
|
|
@END
|
|
|
|
|
|
/* Create a file mapping */
|
|
@REQ(create_mapping)
|
|
int size_high; /* mapping size */
|
|
int size_low; /* mapping size */
|
|
int protect; /* protection flags (see below) */
|
|
int inherit; /* inherit flag */
|
|
handle_t file_handle; /* file handle */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
handle_t handle; /* handle to the mapping */
|
|
@END
|
|
/* protection flags */
|
|
#define VPROT_READ 0x01
|
|
#define VPROT_WRITE 0x02
|
|
#define VPROT_EXEC 0x04
|
|
#define VPROT_WRITECOPY 0x08
|
|
#define VPROT_GUARD 0x10
|
|
#define VPROT_NOCACHE 0x20
|
|
#define VPROT_COMMITTED 0x40
|
|
#define VPROT_IMAGE 0x80
|
|
|
|
|
|
/* Open a mapping */
|
|
@REQ(open_mapping)
|
|
unsigned int access; /* wanted access rights */
|
|
int inherit; /* inherit flag */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
handle_t handle; /* handle to the mapping */
|
|
@END
|
|
|
|
|
|
/* Get information about a file mapping */
|
|
@REQ(get_mapping_info)
|
|
handle_t handle; /* handle to the mapping */
|
|
@REPLY
|
|
int size_high; /* mapping size */
|
|
int size_low; /* mapping size */
|
|
int protect; /* protection flags */
|
|
int header_size; /* header size (for VPROT_IMAGE mapping) */
|
|
void* base; /* default base addr (for VPROT_IMAGE mapping) */
|
|
handle_t shared_file; /* shared mapping file handle */
|
|
int shared_size; /* shared mapping size */
|
|
int drive_type; /* type of drive the file is on */
|
|
@END
|
|
|
|
|
|
/* Create a device */
|
|
@REQ(create_device)
|
|
unsigned int access; /* wanted access rights */
|
|
int inherit; /* inherit flag */
|
|
int id; /* client private id */
|
|
@REPLY
|
|
handle_t handle; /* handle to the device */
|
|
@END
|
|
|
|
|
|
/* Create a snapshot */
|
|
@REQ(create_snapshot)
|
|
int inherit; /* inherit flag */
|
|
int flags; /* snapshot flags (TH32CS_*) */
|
|
void* pid; /* process id */
|
|
@REPLY
|
|
handle_t handle; /* handle to the snapshot */
|
|
@END
|
|
|
|
|
|
/* Get the next process from a snapshot */
|
|
@REQ(next_process)
|
|
handle_t handle; /* handle to the snapshot */
|
|
int reset; /* reset snapshot position? */
|
|
@REPLY
|
|
int count; /* process usage count */
|
|
void* pid; /* process id */
|
|
int threads; /* number of threads */
|
|
int priority; /* process priority */
|
|
@END
|
|
|
|
|
|
/* Get the next thread from a snapshot */
|
|
@REQ(next_thread)
|
|
handle_t handle; /* handle to the snapshot */
|
|
int reset; /* reset snapshot position? */
|
|
@REPLY
|
|
int count; /* thread usage count */
|
|
void* pid; /* process id */
|
|
void* tid; /* thread id */
|
|
int base_pri; /* base priority */
|
|
int delta_pri; /* delta priority */
|
|
@END
|
|
|
|
|
|
/* Get the next module from a snapshot */
|
|
@REQ(next_module)
|
|
handle_t handle; /* handle to the snapshot */
|
|
int reset; /* reset snapshot position? */
|
|
@REPLY
|
|
void* pid; /* process id */
|
|
void* base; /* module base address */
|
|
@END
|
|
|
|
|
|
/* Wait for a debug event */
|
|
@REQ(wait_debug_event)
|
|
int get_handle; /* should we alloc a handle for waiting? */
|
|
@REPLY
|
|
void* pid; /* process id */
|
|
void* tid; /* thread id */
|
|
handle_t wait; /* wait handle if no event ready */
|
|
VARARG(event,debug_event); /* debug event data */
|
|
@END
|
|
|
|
|
|
/* Queue an exception event */
|
|
@REQ(queue_exception_event)
|
|
int first; /* first chance exception? */
|
|
VARARG(record,exc_event); /* thread context followed by exception record */
|
|
@REPLY
|
|
handle_t handle; /* handle to the queued event */
|
|
@END
|
|
|
|
|
|
/* Retrieve the status of an exception event */
|
|
@REQ(get_exception_status)
|
|
handle_t handle; /* handle to the queued event */
|
|
@REPLY
|
|
int status; /* event continuation status */
|
|
VARARG(context,context); /* modified thread context */
|
|
@END
|
|
|
|
|
|
/* Send an output string to the debugger */
|
|
@REQ(output_debug_string)
|
|
void* string; /* string to display (in debugged process address space) */
|
|
int unicode; /* is it Unicode? */
|
|
int length; /* string length */
|
|
@END
|
|
|
|
|
|
/* Continue a debug event */
|
|
@REQ(continue_debug_event)
|
|
void* pid; /* process id to continue */
|
|
void* tid; /* thread id to continue */
|
|
int status; /* continuation status */
|
|
@END
|
|
|
|
|
|
/* Start debugging an existing process */
|
|
@REQ(debug_process)
|
|
void* pid; /* id of the process to debug */
|
|
@END
|
|
|
|
|
|
/* Read data from a process address space */
|
|
@REQ(read_process_memory)
|
|
handle_t handle; /* process handle */
|
|
void* addr; /* addr to read from */
|
|
@REPLY
|
|
VARARG(data,bytes); /* result data */
|
|
@END
|
|
|
|
|
|
/* Write data to a process address space */
|
|
@REQ(write_process_memory)
|
|
handle_t handle; /* process handle */
|
|
void* addr; /* addr to write to (must be int-aligned) */
|
|
unsigned int first_mask; /* mask for first word */
|
|
unsigned int last_mask; /* mask for last word */
|
|
VARARG(data,bytes); /* data to write */
|
|
@END
|
|
|
|
|
|
/* Create a registry key */
|
|
@REQ(create_key)
|
|
handle_t parent; /* handle to the parent key */
|
|
unsigned int access; /* desired access rights */
|
|
unsigned int options; /* creation options */
|
|
time_t modif; /* last modification time */
|
|
size_t namelen; /* length of key name in bytes */
|
|
VARARG(name,unicode_str,namelen); /* key name */
|
|
VARARG(class,unicode_str); /* class name */
|
|
@REPLY
|
|
handle_t hkey; /* handle to the created key */
|
|
int created; /* has it been newly created? */
|
|
@END
|
|
|
|
/* Open a registry key */
|
|
@REQ(open_key)
|
|
handle_t parent; /* handle to the parent key */
|
|
unsigned int access; /* desired access rights */
|
|
VARARG(name,unicode_str); /* key name */
|
|
@REPLY
|
|
handle_t hkey; /* handle to the open key */
|
|
@END
|
|
|
|
|
|
/* Delete a registry key */
|
|
@REQ(delete_key)
|
|
handle_t hkey; /* handle to the key */
|
|
@END
|
|
|
|
|
|
/* Enumerate registry subkeys */
|
|
@REQ(enum_key)
|
|
handle_t hkey; /* handle to registry key */
|
|
int index; /* index of subkey (or -1 for current key) */
|
|
int info_class; /* requested information class */
|
|
@REPLY
|
|
int subkeys; /* number of subkeys */
|
|
int max_subkey; /* longest subkey name */
|
|
int max_class; /* longest class name */
|
|
int values; /* number of values */
|
|
int max_value; /* longest value name */
|
|
int max_data; /* longest value data */
|
|
time_t modif; /* last modification time */
|
|
size_t total; /* total length needed for full name and class */
|
|
size_t namelen; /* length of key name in bytes */
|
|
VARARG(name,unicode_str,namelen); /* key name */
|
|
VARARG(class,unicode_str); /* class name */
|
|
@END
|
|
|
|
|
|
/* Set a value of a registry key */
|
|
@REQ(set_key_value)
|
|
handle_t hkey; /* handle to registry key */
|
|
int type; /* value type */
|
|
size_t namelen; /* length of value name in bytes */
|
|
VARARG(name,unicode_str,namelen); /* value name */
|
|
VARARG(data,bytes); /* value data */
|
|
@END
|
|
|
|
|
|
/* Retrieve the value of a registry key */
|
|
@REQ(get_key_value)
|
|
handle_t hkey; /* handle to registry key */
|
|
VARARG(name,unicode_str); /* value name */
|
|
@REPLY
|
|
int type; /* value type */
|
|
size_t total; /* total length needed for data */
|
|
VARARG(data,bytes); /* value data */
|
|
@END
|
|
|
|
|
|
/* Enumerate a value of a registry key */
|
|
@REQ(enum_key_value)
|
|
handle_t hkey; /* handle to registry key */
|
|
int index; /* value index */
|
|
int info_class; /* requested information class */
|
|
@REPLY
|
|
int type; /* value type */
|
|
size_t total; /* total length needed for full name and data */
|
|
size_t namelen; /* length of value name in bytes */
|
|
VARARG(name,unicode_str,namelen); /* value name */
|
|
VARARG(data,bytes); /* value data */
|
|
@END
|
|
|
|
|
|
/* Delete a value of a registry key */
|
|
@REQ(delete_key_value)
|
|
handle_t hkey; /* handle to registry key */
|
|
VARARG(name,unicode_str); /* value name */
|
|
@END
|
|
|
|
|
|
/* Load a registry branch from a file */
|
|
@REQ(load_registry)
|
|
handle_t hkey; /* root key to load to */
|
|
handle_t file; /* file to load from */
|
|
VARARG(name,unicode_str); /* subkey name */
|
|
@END
|
|
|
|
|
|
/* Save a registry branch to a file */
|
|
@REQ(save_registry)
|
|
handle_t hkey; /* key to save */
|
|
handle_t file; /* file to save to */
|
|
@END
|
|
|
|
|
|
/* Save a registry branch at server exit */
|
|
@REQ(save_registry_atexit)
|
|
handle_t hkey; /* key to save */
|
|
VARARG(file,string); /* file to save to */
|
|
@END
|
|
|
|
|
|
/* Set the current and saving level for the registry */
|
|
@REQ(set_registry_levels)
|
|
int current; /* new current level */
|
|
int saving; /* new saving level */
|
|
int period; /* duration between periodic saves (milliseconds) */
|
|
@END
|
|
|
|
|
|
/* Create a waitable timer */
|
|
@REQ(create_timer)
|
|
int inherit; /* inherit flag */
|
|
int manual; /* manual reset */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
handle_t handle; /* handle to the timer */
|
|
@END
|
|
|
|
|
|
/* Open a waitable timer */
|
|
@REQ(open_timer)
|
|
unsigned int access; /* wanted access rights */
|
|
int inherit; /* inherit flag */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
handle_t handle; /* handle to the timer */
|
|
@END
|
|
|
|
/* Set a waitable timer */
|
|
@REQ(set_timer)
|
|
handle_t handle; /* handle to the timer */
|
|
int sec; /* next expiration absolute time */
|
|
int usec; /* next expiration absolute time */
|
|
int period; /* timer period in ms */
|
|
void* callback; /* callback function */
|
|
void* arg; /* callback argument */
|
|
@END
|
|
|
|
/* Cancel a waitable timer */
|
|
@REQ(cancel_timer)
|
|
handle_t handle; /* handle to the timer */
|
|
@END
|
|
|
|
|
|
/* Retrieve the current context of a thread */
|
|
@REQ(get_thread_context)
|
|
handle_t handle; /* thread handle */
|
|
unsigned int flags; /* context flags */
|
|
@REPLY
|
|
VARARG(context,context); /* thread context */
|
|
@END
|
|
|
|
|
|
/* Set the current context of a thread */
|
|
@REQ(set_thread_context)
|
|
handle_t handle; /* thread handle */
|
|
unsigned int flags; /* context flags */
|
|
VARARG(context,context); /* thread context */
|
|
@END
|
|
|
|
|
|
/* Fetch a selector entry for a thread */
|
|
@REQ(get_selector_entry)
|
|
handle_t handle; /* thread handle */
|
|
int entry; /* LDT entry */
|
|
@REPLY
|
|
unsigned int base; /* selector base */
|
|
unsigned int limit; /* selector limit */
|
|
unsigned char flags; /* selector flags */
|
|
@END
|
|
|
|
|
|
/* Add an atom */
|
|
@REQ(add_atom)
|
|
int local; /* is atom in local process table? */
|
|
VARARG(name,unicode_str); /* atom name */
|
|
@REPLY
|
|
atom_t atom; /* resulting atom */
|
|
@END
|
|
|
|
|
|
/* Delete an atom */
|
|
@REQ(delete_atom)
|
|
atom_t atom; /* atom handle */
|
|
int local; /* is atom in local process table? */
|
|
@END
|
|
|
|
|
|
/* Find an atom */
|
|
@REQ(find_atom)
|
|
int local; /* is atom in local process table? */
|
|
VARARG(name,unicode_str); /* atom name */
|
|
@REPLY
|
|
atom_t atom; /* atom handle */
|
|
@END
|
|
|
|
|
|
/* Get an atom name */
|
|
@REQ(get_atom_name)
|
|
atom_t atom; /* atom handle */
|
|
int local; /* is atom in local process table? */
|
|
@REPLY
|
|
int count; /* atom lock count */
|
|
VARARG(name,unicode_str); /* atom name */
|
|
@END
|
|
|
|
|
|
/* Init the process atom table */
|
|
@REQ(init_atom_table)
|
|
int entries; /* number of entries */
|
|
@END
|
|
|
|
|
|
/* Get the message queue of the current thread */
|
|
@REQ(get_msg_queue)
|
|
@REPLY
|
|
handle_t handle; /* handle to the queue */
|
|
@END
|
|
|
|
|
|
/* Set the current message queue wakeup mask */
|
|
@REQ(set_queue_mask)
|
|
unsigned int wake_mask; /* wakeup bits mask */
|
|
unsigned int changed_mask; /* changed bits mask */
|
|
int skip_wait; /* will we skip waiting if signaled? */
|
|
@REPLY
|
|
unsigned int wake_bits; /* current wake bits */
|
|
unsigned int changed_bits; /* current changed bits */
|
|
@END
|
|
|
|
|
|
/* Get the current message queue status */
|
|
@REQ(get_queue_status)
|
|
int clear; /* should we clear the change bits? */
|
|
@REPLY
|
|
unsigned int wake_bits; /* wake bits */
|
|
unsigned int changed_bits; /* changed bits since last time */
|
|
@END
|
|
|
|
|
|
/* Wait for a process to start waiting on input */
|
|
@REQ(wait_input_idle)
|
|
handle_t handle; /* process handle */
|
|
int timeout; /* timeout */
|
|
@REPLY
|
|
handle_t event; /* handle to idle event */
|
|
@END
|
|
|
|
|
|
/* Send a message to a thread queue */
|
|
@REQ(send_message)
|
|
void* id; /* thread id */
|
|
int type; /* message type (see below) */
|
|
user_handle_t win; /* window handle */
|
|
unsigned int msg; /* message code */
|
|
unsigned int wparam; /* parameters */
|
|
unsigned int lparam; /* parameters */
|
|
int x; /* x position */
|
|
int y; /* y position */
|
|
unsigned int time; /* message time */
|
|
unsigned int info; /* extra info */
|
|
int timeout; /* timeout for reply */
|
|
VARARG(data,bytes); /* message data for sent messages */
|
|
@END
|
|
|
|
enum message_type
|
|
{
|
|
MSG_ASCII, /* Ascii message (from SendMessageA) */
|
|
MSG_UNICODE, /* Unicode message (from SendMessageW) */
|
|
MSG_NOTIFY, /* notify message (from SendNotifyMessageW), always Unicode */
|
|
MSG_CALLBACK, /* callback message (from SendMessageCallbackW), always Unicode */
|
|
MSG_OTHER_PROCESS, /* sent from other process, may include vararg data, always Unicode */
|
|
MSG_POSTED, /* posted message (from PostMessageW), always Unicode */
|
|
MSG_HARDWARE_RAW, /* raw hardware message */
|
|
MSG_HARDWARE_COOKED /* cooked hardware message */
|
|
};
|
|
|
|
|
|
/* Get a message from the current queue */
|
|
@REQ(get_message)
|
|
int flags; /* see below */
|
|
user_handle_t get_win; /* window handle to get */
|
|
unsigned int get_first; /* first message code to get */
|
|
unsigned int get_last; /* last message code to get */
|
|
@REPLY
|
|
int type; /* message type */
|
|
user_handle_t win; /* window handle */
|
|
unsigned int msg; /* message code */
|
|
unsigned int wparam; /* parameters */
|
|
unsigned int lparam; /* parameters */
|
|
int x; /* x position */
|
|
int y; /* y position */
|
|
unsigned int time; /* message time */
|
|
unsigned int info; /* extra info */
|
|
size_t total; /* total size of extra data */
|
|
VARARG(data,bytes); /* message data for sent messages */
|
|
@END
|
|
#define GET_MSG_REMOVE 1 /* remove the message */
|
|
#define GET_MSG_SENT_ONLY 2 /* only get sent messages */
|
|
#define GET_MSG_REMOVE_LAST 4 /* remove last message returned before checking for a new one */
|
|
|
|
/* Reply to a sent message */
|
|
@REQ(reply_message)
|
|
unsigned int result; /* message result */
|
|
int remove; /* should we remove the message? */
|
|
VARARG(data,bytes); /* message data for sent messages */
|
|
@END
|
|
|
|
|
|
/* Retrieve the reply for the last message sent */
|
|
@REQ(get_message_reply)
|
|
int cancel; /* cancel message if not ready? */
|
|
@REPLY
|
|
unsigned int result; /* message result */
|
|
VARARG(data,bytes); /* message data for sent messages */
|
|
@END
|
|
|
|
|
|
/* Set a window timer */
|
|
@REQ(set_win_timer)
|
|
user_handle_t win; /* window handle */
|
|
unsigned int msg; /* message to post */
|
|
unsigned int id; /* timer id */
|
|
unsigned int rate; /* timer rate in ms */
|
|
unsigned int lparam; /* message lparam (callback proc) */
|
|
@END
|
|
|
|
|
|
/* Kill a window timer */
|
|
@REQ(kill_win_timer)
|
|
user_handle_t win; /* window handle */
|
|
unsigned int msg; /* message to post */
|
|
unsigned int id; /* timer id */
|
|
@END
|
|
|
|
|
|
/* Open a serial port */
|
|
@REQ(create_serial)
|
|
unsigned int access; /* wanted access rights */
|
|
int inherit; /* inherit flag */
|
|
unsigned int attributes; /* eg. FILE_FLAG_OVERLAPPED */
|
|
unsigned int sharing; /* sharing flags */
|
|
VARARG(name,string); /* file name */
|
|
@REPLY
|
|
handle_t handle; /* handle to the port */
|
|
@END
|
|
|
|
|
|
/* Retrieve info about a serial port */
|
|
@REQ(get_serial_info)
|
|
handle_t handle; /* handle to comm port */
|
|
@REPLY
|
|
unsigned int readinterval;
|
|
unsigned int readconst;
|
|
unsigned int readmult;
|
|
unsigned int writeconst;
|
|
unsigned int writemult;
|
|
unsigned int eventmask;
|
|
unsigned int commerror;
|
|
@END
|
|
|
|
|
|
/* Set info about a serial port */
|
|
@REQ(set_serial_info)
|
|
handle_t handle; /* handle to comm port */
|
|
int flags; /* bitmask to set values (see below) */
|
|
unsigned int readinterval;
|
|
unsigned int readconst;
|
|
unsigned int readmult;
|
|
unsigned int writeconst;
|
|
unsigned int writemult;
|
|
unsigned int eventmask;
|
|
unsigned int commerror;
|
|
@END
|
|
#define SERIALINFO_SET_TIMEOUTS 0x01
|
|
#define SERIALINFO_SET_MASK 0x02
|
|
#define SERIALINFO_SET_ERROR 0x04
|
|
|
|
|
|
/* Create an async I/O */
|
|
@REQ(create_async)
|
|
handle_t file_handle; /* handle to comm port, socket or file */
|
|
int count;
|
|
int type;
|
|
@REPLY
|
|
int timeout;
|
|
@END
|
|
#define ASYNC_TYPE_READ 0x01
|
|
#define ASYNC_TYPE_WRITE 0x02
|
|
#define ASYNC_TYPE_WAIT 0x03
|
|
|
|
|
|
/* Create a named pipe */
|
|
@REQ(create_named_pipe)
|
|
unsigned int openmode;
|
|
unsigned int pipemode;
|
|
unsigned int maxinstances;
|
|
unsigned int outsize;
|
|
unsigned int insize;
|
|
unsigned int timeout;
|
|
VARARG(filename,string); /* pipe name */
|
|
@REPLY
|
|
handle_t handle; /* handle to the pipe */
|
|
@END
|
|
|
|
|
|
/* Open an existing named pipe */
|
|
@REQ(open_named_pipe)
|
|
unsigned int access;
|
|
VARARG(filename,string); /* pipe name */
|
|
@REPLY
|
|
handle_t handle; /* handle to the pipe */
|
|
@END
|
|
|
|
|
|
/* Connect to a named pipe */
|
|
@REQ(connect_named_pipe)
|
|
handle_t handle;
|
|
void* overlapped;
|
|
void* func;
|
|
@END
|
|
|
|
|
|
/* Wait for a named pipe */
|
|
@REQ(wait_named_pipe)
|
|
unsigned int timeout;
|
|
void* overlapped;
|
|
void* func;
|
|
VARARG(filename,string); /* pipe name */
|
|
@END
|
|
|
|
|
|
/* Disconnect a named pipe */
|
|
@REQ(disconnect_named_pipe)
|
|
handle_t handle;
|
|
@END
|
|
|
|
|
|
@REQ(get_named_pipe_info)
|
|
handle_t handle;
|
|
@REPLY
|
|
unsigned int flags;
|
|
unsigned int maxinstances;
|
|
unsigned int outsize;
|
|
unsigned int insize;
|
|
@END
|
|
|
|
|
|
/* Create a window */
|
|
@REQ(create_window)
|
|
user_handle_t parent; /* parent window */
|
|
user_handle_t owner; /* owner window */
|
|
atom_t atom; /* class atom */
|
|
@REPLY
|
|
user_handle_t handle; /* created window */
|
|
@END
|
|
|
|
|
|
/* Link a window into the tree */
|
|
@REQ(link_window)
|
|
user_handle_t handle; /* handle to the window */
|
|
user_handle_t parent; /* handle to the parent */
|
|
user_handle_t previous; /* previous child in Z-order */
|
|
@REPLY
|
|
user_handle_t full_parent; /* full handle of new parent */
|
|
@END
|
|
|
|
|
|
/* Destroy a window */
|
|
@REQ(destroy_window)
|
|
user_handle_t handle; /* handle to the window */
|
|
@END
|
|
|
|
|
|
/* Set a window owner */
|
|
@REQ(set_window_owner)
|
|
user_handle_t handle; /* handle to the window */
|
|
user_handle_t owner; /* new owner */
|
|
@REPLY
|
|
user_handle_t full_owner; /* full handle of new owner */
|
|
@END
|
|
|
|
|
|
/* Get information from a window handle */
|
|
@REQ(get_window_info)
|
|
user_handle_t handle; /* handle to the window */
|
|
@REPLY
|
|
user_handle_t full_handle; /* full 32-bit handle */
|
|
void* pid; /* process owning the window */
|
|
void* tid; /* thread owning the window */
|
|
atom_t atom; /* class atom */
|
|
@END
|
|
|
|
|
|
/* Set some information in a window */
|
|
@REQ(set_window_info)
|
|
user_handle_t handle; /* handle to the window */
|
|
unsigned int flags; /* flags for fields to set (see below) */
|
|
unsigned int style; /* window style */
|
|
unsigned int ex_style; /* window extended style */
|
|
unsigned int id; /* window id */
|
|
void* instance; /* creator instance */
|
|
void* user_data; /* user-specific data */
|
|
@REPLY
|
|
unsigned int old_style; /* old window style */
|
|
unsigned int old_ex_style; /* old window extended style */
|
|
unsigned int old_id; /* old window id */
|
|
void* old_instance; /* old creator instance */
|
|
void* old_user_data; /* old user-specific data */
|
|
@END
|
|
#define SET_WIN_STYLE 0x01
|
|
#define SET_WIN_EXSTYLE 0x02
|
|
#define SET_WIN_ID 0x04
|
|
#define SET_WIN_INSTANCE 0x08
|
|
#define SET_WIN_USERDATA 0x10
|
|
|
|
|
|
/* Get a list of the window parents, up to the root of the tree */
|
|
@REQ(get_window_parents)
|
|
user_handle_t handle; /* handle to the window */
|
|
@REPLY
|
|
int count; /* total count of parents */
|
|
VARARG(parents,user_handles); /* parent handles */
|
|
@END
|
|
|
|
|
|
/* Get a list of the window children */
|
|
@REQ(get_window_children)
|
|
user_handle_t parent; /* parent window */
|
|
atom_t atom; /* class atom for the listed children */
|
|
void* tid; /* thread owning the listed children */
|
|
@REPLY
|
|
int count; /* total count of children */
|
|
VARARG(children,user_handles); /* children handles */
|
|
@END
|
|
|
|
|
|
/* Get window tree information from a window handle */
|
|
@REQ(get_window_tree)
|
|
user_handle_t handle; /* handle to the window */
|
|
@REPLY
|
|
user_handle_t parent; /* parent window */
|
|
user_handle_t owner; /* owner window */
|
|
user_handle_t next_sibling; /* next sibling in Z-order */
|
|
user_handle_t prev_sibling; /* prev sibling in Z-order */
|
|
user_handle_t first_sibling; /* first sibling in Z-order */
|
|
user_handle_t last_sibling; /* last sibling in Z-order */
|
|
user_handle_t first_child; /* first child */
|
|
user_handle_t last_child; /* last child */
|
|
@END
|
|
|
|
/* Set the window and client rectangles of a window */
|
|
@REQ(set_window_rectangles)
|
|
user_handle_t handle; /* handle to the window */
|
|
rectangle_t window; /* window rectangle */
|
|
rectangle_t client; /* client rectangle */
|
|
@END
|
|
|
|
|
|
/* Get the window and client rectangles of a window */
|
|
@REQ(get_window_rectangles)
|
|
user_handle_t handle; /* handle to the window */
|
|
@REPLY
|
|
rectangle_t window; /* window rectangle */
|
|
rectangle_t client; /* client rectangle */
|
|
@END
|
|
|
|
|
|
/* Get the window text */
|
|
@REQ(get_window_text)
|
|
user_handle_t handle; /* handle to the window */
|
|
@REPLY
|
|
VARARG(text,unicode_str); /* window text */
|
|
@END
|
|
|
|
|
|
/* Set the window text */
|
|
@REQ(set_window_text)
|
|
user_handle_t handle; /* handle to the window */
|
|
VARARG(text,unicode_str); /* window text */
|
|
@END
|
|
|
|
|
|
/* Increment the window paint count */
|
|
@REQ(inc_window_paint_count)
|
|
user_handle_t handle; /* handle to the window */
|
|
int incr; /* increment (can be negative) */
|
|
@END
|
|
|
|
|
|
/* Get the coordinates offset between two windows */
|
|
@REQ(get_windows_offset)
|
|
user_handle_t from; /* handle to the first window */
|
|
user_handle_t to; /* handle to the second window */
|
|
@REPLY
|
|
int x; /* x coordinate offset */
|
|
int y; /* y coordinate offset */
|
|
@END
|
|
|
|
|
|
/* Set a window property */
|
|
@REQ(set_window_property)
|
|
user_handle_t window; /* handle to the window */
|
|
atom_t atom; /* property atom (high-word set if it was a string) */
|
|
int string; /* was atom a string originally? */
|
|
handle_t handle; /* handle to store */
|
|
@END
|
|
|
|
|
|
/* Remove a window property */
|
|
@REQ(remove_window_property)
|
|
user_handle_t window; /* handle to the window */
|
|
atom_t atom; /* property atom */
|
|
@REPLY
|
|
handle_t handle; /* handle stored in property */
|
|
@END
|
|
|
|
|
|
/* Get a window property */
|
|
@REQ(get_window_property)
|
|
user_handle_t window; /* handle to the window */
|
|
atom_t atom; /* property atom */
|
|
@REPLY
|
|
handle_t handle; /* handle stored in property */
|
|
@END
|
|
|
|
|
|
/* Get the list of properties of a window */
|
|
@REQ(get_window_properties)
|
|
user_handle_t window; /* handle to the window */
|
|
@REPLY
|
|
int total; /* total number of properties */
|
|
VARARG(props,properties); /* list of properties */
|
|
@END
|