mirror of
git://source.winehq.org/git/wine.git
synced 2024-09-14 12:06:07 +00:00
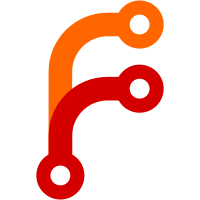
Signed-off-by: Ziqing Hui <zhui@codeweavers.com> Signed-off-by: Henri Verbeet <hverbeet@codeweavers.com> Signed-off-by: Alexandre Julliard <julliard@winehq.org>
156 lines
4.1 KiB
Plaintext
156 lines
4.1 KiB
Plaintext
/*
|
|
* Copyright 2022 Ziqing Hui for CodeWeavers
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2.1 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301, USA
|
|
*/
|
|
|
|
import "d2d1_2.idl";
|
|
|
|
typedef enum D2D1_INK_NIB_SHAPE
|
|
{
|
|
D2D1_INK_NIB_SHAPE_ROUND = 0x0,
|
|
D2D1_INK_NIB_SHAPE_SQUARE = 0x1,
|
|
D2D1_INK_NIB_SHAPE_FORCE_DWORD = 0xffffffff
|
|
} D2D1_INK_NIB_SHAPE;
|
|
|
|
typedef struct D2D1_INK_POINT
|
|
{
|
|
float x;
|
|
float y;
|
|
float radius;
|
|
} D2D1_INK_POINT;
|
|
|
|
typedef struct D2D1_INK_BEZIER_SEGMENT
|
|
{
|
|
D2D1_INK_POINT point1;
|
|
D2D1_INK_POINT point2;
|
|
D2D1_INK_POINT point3;
|
|
} D2D1_INK_BEZIER_SEGMENT;
|
|
|
|
typedef enum D2D1_PATCH_EDGE_MODE
|
|
{
|
|
D2D1_PATCH_EDGE_MODE_ALIASED = 0x0,
|
|
D2D1_PATCH_EDGE_MODE_ANTIALIASED = 0x1,
|
|
D2D1_PATCH_EDGE_MODE_ALIASED_INFLATED = 0x2,
|
|
D2D1_PATCH_EDGE_MODE_FORCE_DWORD = 0xffffffff
|
|
} D2D1_PATCH_EDGE_MODE;
|
|
|
|
typedef struct D2D1_GRADIENT_MESH_PATCH
|
|
{
|
|
D2D1_POINT_2F point00;
|
|
D2D1_POINT_2F point01;
|
|
D2D1_POINT_2F point02;
|
|
D2D1_POINT_2F point03;
|
|
D2D1_POINT_2F point10;
|
|
D2D1_POINT_2F point11;
|
|
D2D1_POINT_2F point12;
|
|
D2D1_POINT_2F point13;
|
|
D2D1_POINT_2F point20;
|
|
D2D1_POINT_2F point21;
|
|
D2D1_POINT_2F point22;
|
|
D2D1_POINT_2F point23;
|
|
D2D1_POINT_2F point30;
|
|
D2D1_POINT_2F point31;
|
|
D2D1_POINT_2F point32;
|
|
D2D1_POINT_2F point33;
|
|
D2D1_COLOR_F color00;
|
|
D2D1_COLOR_F color03;
|
|
D2D1_COLOR_F color30;
|
|
D2D1_COLOR_F color33;
|
|
D2D1_PATCH_EDGE_MODE topEdgeMode;
|
|
D2D1_PATCH_EDGE_MODE leftEdgeMode;
|
|
D2D1_PATCH_EDGE_MODE bottomEdgeMode;
|
|
D2D1_PATCH_EDGE_MODE rightEdgeMode;
|
|
} D2D1_GRADIENT_MESH_PATCH;
|
|
|
|
[
|
|
object,
|
|
uuid(bae8b344-23fc-4071-8cb5-d05d6f073848),
|
|
local,
|
|
]
|
|
interface ID2D1InkStyle : ID2D1Resource
|
|
{
|
|
void SetNibTransform(
|
|
[in] const D2D1_MATRIX_3X2_F *transform
|
|
);
|
|
void GetNibTransform(
|
|
[out] D2D1_MATRIX_3X2_F *transform
|
|
);
|
|
void SetNibShape(
|
|
[in] D2D1_INK_NIB_SHAPE shape
|
|
);
|
|
D2D1_INK_NIB_SHAPE GetNibShape();
|
|
};
|
|
|
|
[
|
|
object,
|
|
uuid(b499923b-7029-478f-a8b3-432c7c5f5312),
|
|
local,
|
|
]
|
|
interface ID2D1Ink : ID2D1Resource
|
|
{
|
|
void SetStartPoint(
|
|
[in] const D2D1_INK_POINT *start_point
|
|
);
|
|
D2D1_INK_POINT GetStartPoint();
|
|
HRESULT AddSegments(
|
|
[in] const D2D1_INK_BEZIER_SEGMENT *segments,
|
|
[in] UINT32 segment_count
|
|
);
|
|
HRESULT RemoveSegmentsAtEnd(
|
|
[in] UINT32 segment_count
|
|
);
|
|
HRESULT SetSegments(
|
|
[in] UINT32 start_segment,
|
|
[in] const D2D1_INK_BEZIER_SEGMENT *segments,
|
|
[in] UINT32 segment_count
|
|
);
|
|
HRESULT SetSegmentAtEnd(
|
|
[in] const D2D1_INK_BEZIER_SEGMENT *segment
|
|
);
|
|
UINT32 GetSegmentCount();
|
|
HRESULT GetSegments(
|
|
[in] UINT32 start_segment,
|
|
[out] D2D1_INK_BEZIER_SEGMENT *segments,
|
|
[in] UINT32 segment_count
|
|
);
|
|
HRESULT StreamAsGeometry(
|
|
[in, optional] ID2D1InkStyle *ink_style,
|
|
[in, optional] const D2D1_MATRIX_3X2_F *world_transform,
|
|
[in] float flattening_tolerance,
|
|
[in] ID2D1SimplifiedGeometrySink *geometry_sink
|
|
);
|
|
HRESULT GetBounds(
|
|
[in, optional] ID2D1InkStyle *ink_style,
|
|
[in, optional] const D2D1_MATRIX_3X2_F *world_transform,
|
|
[out] D2D1_RECT_F *bounds
|
|
);
|
|
};
|
|
|
|
[
|
|
object,
|
|
uuid(f292e401-c050-4cde-83d7-04962d3b23c2),
|
|
local,
|
|
]
|
|
interface ID2D1GradientMesh : ID2D1Resource
|
|
{
|
|
UINT32 GetPatchCount();
|
|
HRESULT GetPatches(
|
|
[in] UINT32 start_index,
|
|
[out] D2D1_GRADIENT_MESH_PATCH *patches,
|
|
[in] UINT32 patch_count
|
|
);
|
|
};
|