mirror of
git://source.winehq.org/git/wine.git
synced 2024-09-15 04:34:48 +00:00
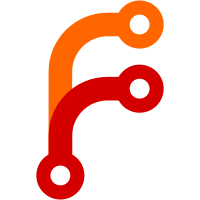
This is needed to return the correct value when one of the handles being waited on is set to signaled state by the APC.
2915 lines
100 KiB
C
2915 lines
100 KiB
C
/* -*- C -*-
|
|
*
|
|
* Wine server protocol definition
|
|
*
|
|
* Copyright (C) 2001 Alexandre Julliard
|
|
*
|
|
* This file is used by tools/make_requests to build the
|
|
* protocol structures in include/wine/server_protocol.h
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2.1 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301, USA
|
|
*/
|
|
|
|
@HEADER /* start of C declarations */
|
|
|
|
#include <stdarg.h>
|
|
#include <stdlib.h>
|
|
#include <time.h>
|
|
|
|
#include <windef.h>
|
|
#include <winbase.h>
|
|
|
|
typedef void *obj_handle_t;
|
|
typedef void *user_handle_t;
|
|
typedef unsigned short atom_t;
|
|
typedef unsigned int process_id_t;
|
|
typedef unsigned int thread_id_t;
|
|
typedef unsigned int data_size_t;
|
|
typedef unsigned int ioctl_code_t;
|
|
|
|
struct request_header
|
|
{
|
|
int req; /* request code */
|
|
data_size_t request_size; /* request variable part size */
|
|
data_size_t reply_size; /* reply variable part maximum size */
|
|
};
|
|
|
|
struct reply_header
|
|
{
|
|
unsigned int error; /* error result */
|
|
data_size_t reply_size; /* reply variable part size */
|
|
};
|
|
|
|
/* placeholder structure for the maximum allowed request size */
|
|
/* this is used to construct the generic_request union */
|
|
struct request_max_size
|
|
{
|
|
int pad[16]; /* the max request size is 16 ints */
|
|
};
|
|
|
|
#define FIRST_USER_HANDLE 0x0020 /* first possible value for low word of user handle */
|
|
#define LAST_USER_HANDLE 0xffef /* last possible value for low word of user handle */
|
|
|
|
|
|
/* definitions of the event data depending on the event code */
|
|
struct debug_event_exception
|
|
{
|
|
EXCEPTION_RECORD record; /* exception record */
|
|
int first; /* first chance exception? */
|
|
};
|
|
struct debug_event_create_thread
|
|
{
|
|
obj_handle_t handle; /* handle to the new thread */
|
|
void *teb; /* thread teb (in debugged process address space) */
|
|
void *start; /* thread startup routine */
|
|
};
|
|
struct debug_event_create_process
|
|
{
|
|
obj_handle_t file; /* handle to the process exe file */
|
|
obj_handle_t process; /* handle to the new process */
|
|
obj_handle_t thread; /* handle to the new thread */
|
|
void *base; /* base of executable image */
|
|
int dbg_offset; /* offset of debug info in file */
|
|
int dbg_size; /* size of debug info */
|
|
void *teb; /* thread teb (in debugged process address space) */
|
|
void *start; /* thread startup routine */
|
|
void *name; /* image name (optional) */
|
|
int unicode; /* is it Unicode? */
|
|
};
|
|
struct debug_event_exit
|
|
{
|
|
int exit_code; /* thread or process exit code */
|
|
};
|
|
struct debug_event_load_dll
|
|
{
|
|
obj_handle_t handle; /* file handle for the dll */
|
|
void *base; /* base address of the dll */
|
|
int dbg_offset; /* offset of debug info in file */
|
|
int dbg_size; /* size of debug info */
|
|
void *name; /* image name (optional) */
|
|
int unicode; /* is it Unicode? */
|
|
};
|
|
struct debug_event_unload_dll
|
|
{
|
|
void *base; /* base address of the dll */
|
|
};
|
|
struct debug_event_output_string
|
|
{
|
|
void *string; /* string to display (in debugged process address space) */
|
|
int unicode; /* is it Unicode? */
|
|
int length; /* string length */
|
|
};
|
|
struct debug_event_rip_info
|
|
{
|
|
int error; /* ??? */
|
|
int type; /* ??? */
|
|
};
|
|
union debug_event_data
|
|
{
|
|
struct debug_event_exception exception;
|
|
struct debug_event_create_thread create_thread;
|
|
struct debug_event_create_process create_process;
|
|
struct debug_event_exit exit;
|
|
struct debug_event_load_dll load_dll;
|
|
struct debug_event_unload_dll unload_dll;
|
|
struct debug_event_output_string output_string;
|
|
struct debug_event_rip_info rip_info;
|
|
};
|
|
|
|
/* debug event data */
|
|
typedef struct
|
|
{
|
|
int code; /* event code */
|
|
union debug_event_data info; /* event information */
|
|
} debug_event_t;
|
|
|
|
/* structure used in sending an fd from client to server */
|
|
struct send_fd
|
|
{
|
|
thread_id_t tid; /* thread id */
|
|
int fd; /* file descriptor on client-side */
|
|
};
|
|
|
|
/* structure sent by the server on the wait fifo */
|
|
struct wake_up_reply
|
|
{
|
|
void *cookie; /* magic cookie that was passed in select_request */
|
|
int signaled; /* wait result */
|
|
};
|
|
|
|
/* NT-style timeout, in 100ns units, negative means relative timeout */
|
|
typedef __int64 timeout_t;
|
|
#define TIMEOUT_INFINITE (((timeout_t)0x7fffffff) << 32 | 0xffffffff)
|
|
|
|
/* structure returned in the list of window properties */
|
|
typedef struct
|
|
{
|
|
atom_t atom; /* property atom */
|
|
short string; /* was atom a string originally? */
|
|
obj_handle_t handle; /* handle stored in property */
|
|
} property_data_t;
|
|
|
|
/* structure to specify window rectangles */
|
|
typedef struct
|
|
{
|
|
int left;
|
|
int top;
|
|
int right;
|
|
int bottom;
|
|
} rectangle_t;
|
|
|
|
/* structure for parameters of async I/O calls */
|
|
typedef struct
|
|
{
|
|
void *callback; /* client-side callback to call upon end of async */
|
|
void *iosb; /* I/O status block in client addr space */
|
|
void *arg; /* opaque user data to pass to callback */
|
|
void *apc; /* user apc to call */
|
|
obj_handle_t event; /* event to signal when done */
|
|
} async_data_t;
|
|
|
|
/* structures for extra message data */
|
|
|
|
struct callback_msg_data
|
|
{
|
|
void *callback; /* callback function */
|
|
unsigned long data; /* user data for callback */
|
|
unsigned long result; /* message result */
|
|
};
|
|
|
|
struct winevent_msg_data
|
|
{
|
|
user_handle_t hook; /* hook handle */
|
|
thread_id_t tid; /* thread id */
|
|
void *hook_proc; /* hook proc address */
|
|
/* followed by module name if any */
|
|
};
|
|
|
|
typedef union
|
|
{
|
|
unsigned char bytes[1]; /* raw data for sent messages */
|
|
struct callback_msg_data callback;
|
|
struct winevent_msg_data winevent;
|
|
} message_data_t;
|
|
|
|
/* structure for console char/attribute info */
|
|
typedef struct
|
|
{
|
|
WCHAR ch;
|
|
unsigned short attr;
|
|
} char_info_t;
|
|
|
|
typedef struct
|
|
{
|
|
unsigned int low_part;
|
|
int high_part;
|
|
} luid_t;
|
|
|
|
#define MAX_ACL_LEN 65535
|
|
|
|
struct security_descriptor
|
|
{
|
|
unsigned int control; /* SE_ flags */
|
|
data_size_t owner_len;
|
|
data_size_t group_len;
|
|
data_size_t sacl_len;
|
|
data_size_t dacl_len;
|
|
/* VARARGS(owner,SID); */
|
|
/* VARARGS(group,SID); */
|
|
/* VARARGS(sacl,ACL); */
|
|
/* VARARGS(dacl,ACL); */
|
|
};
|
|
|
|
struct token_groups
|
|
{
|
|
unsigned int count;
|
|
/* unsigned int attributes[count]; */
|
|
/* VARARGS(sids,SID); */
|
|
};
|
|
|
|
enum apc_type
|
|
{
|
|
APC_NONE,
|
|
APC_USER,
|
|
APC_TIMER,
|
|
APC_ASYNC_IO,
|
|
APC_VIRTUAL_ALLOC,
|
|
APC_VIRTUAL_FREE,
|
|
APC_VIRTUAL_QUERY,
|
|
APC_VIRTUAL_PROTECT,
|
|
APC_VIRTUAL_FLUSH,
|
|
APC_VIRTUAL_LOCK,
|
|
APC_VIRTUAL_UNLOCK,
|
|
APC_MAP_VIEW,
|
|
APC_UNMAP_VIEW,
|
|
APC_CREATE_THREAD
|
|
};
|
|
|
|
typedef union
|
|
{
|
|
enum apc_type type;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_USER */
|
|
void (__stdcall *func)(unsigned long,unsigned long,unsigned long);
|
|
unsigned long args[3]; /* arguments for user function */
|
|
} user;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_TIMER */
|
|
void (__stdcall *func)(void*, unsigned int, unsigned int);
|
|
timeout_t time; /* absolute time of expiration */
|
|
void *arg; /* user argument */
|
|
} timer;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_ASYNC_IO */
|
|
unsigned int (*func)(void*, void*, unsigned int);
|
|
void *user; /* user pointer */
|
|
void *sb; /* status block */
|
|
unsigned int status; /* I/O status */
|
|
} async_io;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_VIRTUAL_ALLOC */
|
|
void *addr; /* requested address */
|
|
unsigned long size; /* allocation size */
|
|
unsigned int zero_bits; /* allocation alignment */
|
|
unsigned int op_type; /* type of operation */
|
|
unsigned int prot; /* memory protection flags */
|
|
} virtual_alloc;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_VIRTUAL_FREE */
|
|
void *addr; /* requested address */
|
|
unsigned long size; /* allocation size */
|
|
unsigned int op_type; /* type of operation */
|
|
} virtual_free;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_VIRTUAL_QUERY */
|
|
const void *addr; /* requested address */
|
|
} virtual_query;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_VIRTUAL_PROTECT */
|
|
void *addr; /* requested address */
|
|
unsigned long size; /* requested address */
|
|
unsigned int prot; /* new protection flags */
|
|
} virtual_protect;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_VIRTUAL_FLUSH */
|
|
const void *addr; /* requested address */
|
|
unsigned long size; /* requested address */
|
|
} virtual_flush;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_VIRTUAL_LOCK */
|
|
void *addr; /* requested address */
|
|
unsigned long size; /* requested address */
|
|
} virtual_lock;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_VIRTUAL_UNLOCK */
|
|
void *addr; /* requested address */
|
|
unsigned long size; /* requested address */
|
|
} virtual_unlock;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_MAP_VIEW */
|
|
obj_handle_t handle; /* mapping handle */
|
|
void *addr; /* requested address */
|
|
unsigned long size; /* allocation size */
|
|
unsigned int offset_low;/* file offset */
|
|
unsigned int offset_high;
|
|
unsigned int zero_bits; /* allocation alignment */
|
|
unsigned int alloc_type;/* allocation type */
|
|
unsigned int prot; /* memory protection flags */
|
|
} map_view;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_UNMAP_VIEW */
|
|
void *addr; /* view address */
|
|
} unmap_view;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_CREATE_THREAD */
|
|
void (__stdcall *func)(void*); /* start function */
|
|
void *arg; /* argument for start function */
|
|
unsigned long reserve; /* reserve size for thread stack */
|
|
unsigned long commit; /* commit size for thread stack */
|
|
int suspend; /* suspended thread? */
|
|
} create_thread;
|
|
} apc_call_t;
|
|
|
|
typedef union
|
|
{
|
|
enum apc_type type;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_ASYNC_IO */
|
|
unsigned int status; /* new status of async operation */
|
|
} async_io;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_VIRTUAL_ALLOC */
|
|
unsigned int status; /* status returned by call */
|
|
void *addr; /* resulting address */
|
|
unsigned long size; /* resulting size */
|
|
} virtual_alloc;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_VIRTUAL_FREE */
|
|
unsigned int status; /* status returned by call */
|
|
void *addr; /* resulting address */
|
|
unsigned long size; /* resulting size */
|
|
} virtual_free;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_VIRTUAL_QUERY */
|
|
unsigned int status; /* status returned by call */
|
|
void *base; /* resulting base address */
|
|
void *alloc_base;/* resulting allocation base */
|
|
unsigned long size; /* resulting region size */
|
|
unsigned int state; /* resulting region state */
|
|
unsigned int prot; /* resulting region protection */
|
|
unsigned int alloc_prot;/* resulting allocation protection */
|
|
unsigned int alloc_type;/* resulting region allocation type */
|
|
} virtual_query;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_VIRTUAL_PROTECT */
|
|
unsigned int status; /* status returned by call */
|
|
void *addr; /* resulting address */
|
|
unsigned long size; /* resulting size */
|
|
unsigned int prot; /* old protection flags */
|
|
} virtual_protect;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_VIRTUAL_FLUSH */
|
|
unsigned int status; /* status returned by call */
|
|
const void *addr; /* resulting address */
|
|
unsigned long size; /* resulting size */
|
|
} virtual_flush;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_VIRTUAL_LOCK */
|
|
unsigned int status; /* status returned by call */
|
|
void *addr; /* resulting address */
|
|
unsigned long size; /* resulting size */
|
|
} virtual_lock;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_VIRTUAL_UNLOCK */
|
|
unsigned int status; /* status returned by call */
|
|
void *addr; /* resulting address */
|
|
unsigned long size; /* resulting size */
|
|
} virtual_unlock;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_MAP_VIEW */
|
|
unsigned int status; /* status returned by call */
|
|
void *addr; /* resulting address */
|
|
unsigned long size; /* resulting size */
|
|
} map_view;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_MAP_VIEW */
|
|
unsigned int status; /* status returned by call */
|
|
} unmap_view;
|
|
struct
|
|
{
|
|
enum apc_type type; /* APC_CREATE_THREAD */
|
|
unsigned int status; /* status returned by call */
|
|
thread_id_t tid; /* thread id */
|
|
obj_handle_t handle; /* handle to new thread */
|
|
} create_thread;
|
|
} apc_result_t;
|
|
|
|
/****************************************************************/
|
|
/* Request declarations */
|
|
|
|
/* Create a new process from the context of the parent */
|
|
@REQ(new_process)
|
|
int inherit_all; /* inherit all handles from parent */
|
|
unsigned int create_flags; /* creation flags */
|
|
int socket_fd; /* file descriptor for process socket */
|
|
obj_handle_t exe_file; /* file handle for main exe */
|
|
obj_handle_t hstdin; /* handle for stdin */
|
|
obj_handle_t hstdout; /* handle for stdout */
|
|
obj_handle_t hstderr; /* handle for stderr */
|
|
unsigned int process_access; /* access rights for process object */
|
|
unsigned int process_attr; /* attributes for process object */
|
|
unsigned int thread_access; /* access rights for thread object */
|
|
unsigned int thread_attr; /* attributes for thread object */
|
|
VARARG(info,startup_info); /* startup information */
|
|
VARARG(env,unicode_str); /* environment for new process */
|
|
@REPLY
|
|
obj_handle_t info; /* new process info handle */
|
|
process_id_t pid; /* process id */
|
|
obj_handle_t phandle; /* process handle (in the current process) */
|
|
thread_id_t tid; /* thread id */
|
|
obj_handle_t thandle; /* thread handle (in the current process) */
|
|
@END
|
|
|
|
|
|
/* Retrieve information about a newly started process */
|
|
@REQ(get_new_process_info)
|
|
obj_handle_t info; /* info handle returned from new_process_request */
|
|
@REPLY
|
|
int success; /* did the process start successfully? */
|
|
int exit_code; /* process exit code if failed */
|
|
@END
|
|
|
|
|
|
/* Create a new thread from the context of the parent */
|
|
@REQ(new_thread)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
int suspend; /* new thread should be suspended on creation */
|
|
int request_fd; /* fd for request pipe */
|
|
@REPLY
|
|
thread_id_t tid; /* thread id */
|
|
obj_handle_t handle; /* thread handle (in the current process) */
|
|
@END
|
|
|
|
|
|
/* Retrieve the new process startup info */
|
|
@REQ(get_startup_info)
|
|
@REPLY
|
|
obj_handle_t exe_file; /* file handle for main exe */
|
|
obj_handle_t hstdin; /* handle for stdin */
|
|
obj_handle_t hstdout; /* handle for stdout */
|
|
obj_handle_t hstderr; /* handle for stderr */
|
|
VARARG(info,startup_info); /* startup information */
|
|
VARARG(env,unicode_str); /* environment */
|
|
@END
|
|
|
|
|
|
/* Signal the end of the process initialization */
|
|
@REQ(init_process_done)
|
|
void* module; /* main module base address */
|
|
void* entry; /* process entry point */
|
|
int gui; /* is it a GUI process? */
|
|
@END
|
|
|
|
|
|
/* Initialize a thread; called from the child after fork()/clone() */
|
|
@REQ(init_thread)
|
|
int unix_pid; /* Unix pid of new thread */
|
|
int unix_tid; /* Unix tid of new thread */
|
|
int debug_level; /* new debug level */
|
|
void* teb; /* TEB of new thread (in thread address space) */
|
|
void* peb; /* address of PEB (in thread address space) */
|
|
void* entry; /* thread entry point (in thread address space) */
|
|
void* ldt_copy; /* address of LDT copy (in thread address space) */
|
|
int reply_fd; /* fd for reply pipe */
|
|
int wait_fd; /* fd for blocking calls pipe */
|
|
@REPLY
|
|
process_id_t pid; /* process id of the new thread's process */
|
|
thread_id_t tid; /* thread id of the new thread */
|
|
data_size_t info_size; /* total size of startup info */
|
|
timeout_t server_start; /* server start time */
|
|
int version; /* protocol version */
|
|
@END
|
|
|
|
|
|
/* Terminate a process */
|
|
@REQ(terminate_process)
|
|
obj_handle_t handle; /* process handle to terminate */
|
|
int exit_code; /* process exit code */
|
|
@REPLY
|
|
int self; /* suicide? */
|
|
@END
|
|
|
|
|
|
/* Terminate a thread */
|
|
@REQ(terminate_thread)
|
|
obj_handle_t handle; /* thread handle to terminate */
|
|
int exit_code; /* thread exit code */
|
|
@REPLY
|
|
int self; /* suicide? */
|
|
int last; /* last thread in this process? */
|
|
@END
|
|
|
|
|
|
/* Retrieve information about a process */
|
|
@REQ(get_process_info)
|
|
obj_handle_t handle; /* process handle */
|
|
@REPLY
|
|
process_id_t pid; /* server process id */
|
|
process_id_t ppid; /* server process id of parent */
|
|
int exit_code; /* process exit code */
|
|
int priority; /* priority class */
|
|
int affinity; /* process affinity mask */
|
|
void* peb; /* PEB address in process address space */
|
|
timeout_t start_time; /* process start time */
|
|
timeout_t end_time; /* process end time */
|
|
@END
|
|
|
|
|
|
/* Set a process informations */
|
|
@REQ(set_process_info)
|
|
obj_handle_t handle; /* process handle */
|
|
int mask; /* setting mask (see below) */
|
|
int priority; /* priority class */
|
|
int affinity; /* affinity mask */
|
|
@END
|
|
#define SET_PROCESS_INFO_PRIORITY 0x01
|
|
#define SET_PROCESS_INFO_AFFINITY 0x02
|
|
|
|
|
|
/* Retrieve information about a thread */
|
|
@REQ(get_thread_info)
|
|
obj_handle_t handle; /* thread handle */
|
|
thread_id_t tid_in; /* thread id (optional) */
|
|
@REPLY
|
|
process_id_t pid; /* server process id */
|
|
thread_id_t tid; /* server thread id */
|
|
void* teb; /* thread teb pointer */
|
|
int exit_code; /* thread exit code */
|
|
int priority; /* thread priority level */
|
|
int affinity; /* thread affinity mask */
|
|
timeout_t creation_time; /* thread creation time */
|
|
timeout_t exit_time; /* thread exit time */
|
|
int last; /* last thread in process */
|
|
@END
|
|
|
|
|
|
/* Set a thread informations */
|
|
@REQ(set_thread_info)
|
|
obj_handle_t handle; /* thread handle */
|
|
int mask; /* setting mask (see below) */
|
|
int priority; /* priority class */
|
|
int affinity; /* affinity mask */
|
|
obj_handle_t token; /* impersonation token */
|
|
@END
|
|
#define SET_THREAD_INFO_PRIORITY 0x01
|
|
#define SET_THREAD_INFO_AFFINITY 0x02
|
|
#define SET_THREAD_INFO_TOKEN 0x04
|
|
|
|
|
|
/* Retrieve information about a module */
|
|
@REQ(get_dll_info)
|
|
obj_handle_t handle; /* process handle */
|
|
void* base_address; /* base address of module */
|
|
@REPLY
|
|
size_t size; /* module size */
|
|
void* entry_point;
|
|
VARARG(filename,unicode_str); /* file name of module */
|
|
@END
|
|
|
|
|
|
/* Suspend a thread */
|
|
@REQ(suspend_thread)
|
|
obj_handle_t handle; /* thread handle */
|
|
@REPLY
|
|
int count; /* new suspend count */
|
|
@END
|
|
|
|
|
|
/* Resume a thread */
|
|
@REQ(resume_thread)
|
|
obj_handle_t handle; /* thread handle */
|
|
@REPLY
|
|
int count; /* new suspend count */
|
|
@END
|
|
|
|
|
|
/* Notify the server that a dll has been loaded */
|
|
@REQ(load_dll)
|
|
obj_handle_t handle; /* file handle */
|
|
void* base; /* base address */
|
|
size_t size; /* dll size */
|
|
int dbg_offset; /* debug info offset */
|
|
int dbg_size; /* debug info size */
|
|
void* name; /* ptr to ptr to name (in process addr space) */
|
|
VARARG(filename,unicode_str); /* file name of dll */
|
|
@END
|
|
|
|
|
|
/* Notify the server that a dll is being unloaded */
|
|
@REQ(unload_dll)
|
|
void* base; /* base address */
|
|
@END
|
|
|
|
|
|
/* Queue an APC for a thread or process */
|
|
@REQ(queue_apc)
|
|
obj_handle_t thread; /* thread handle */
|
|
obj_handle_t process; /* process handle */
|
|
apc_call_t call; /* call arguments */
|
|
@REPLY
|
|
obj_handle_t handle; /* APC handle */
|
|
int self; /* run APC in caller itself? */
|
|
@END
|
|
|
|
|
|
/* Get the result of an APC call */
|
|
@REQ(get_apc_result)
|
|
obj_handle_t handle; /* handle to the APC */
|
|
@REPLY
|
|
apc_result_t result; /* result of the APC */
|
|
@END
|
|
|
|
|
|
/* Close a handle for the current process */
|
|
@REQ(close_handle)
|
|
obj_handle_t handle; /* handle to close */
|
|
@END
|
|
|
|
|
|
/* Set a handle information */
|
|
@REQ(set_handle_info)
|
|
obj_handle_t handle; /* handle we are interested in */
|
|
int flags; /* new handle flags */
|
|
int mask; /* mask for flags to set */
|
|
@REPLY
|
|
int old_flags; /* old flag value */
|
|
@END
|
|
|
|
|
|
/* Duplicate a handle */
|
|
@REQ(dup_handle)
|
|
obj_handle_t src_process; /* src process handle */
|
|
obj_handle_t src_handle; /* src handle to duplicate */
|
|
obj_handle_t dst_process; /* dst process handle */
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
unsigned int options; /* duplicate options (see below) */
|
|
@REPLY
|
|
obj_handle_t handle; /* duplicated handle in dst process */
|
|
int self; /* is the source the current process? */
|
|
int closed; /* whether the source handle has been closed */
|
|
@END
|
|
#define DUP_HANDLE_CLOSE_SOURCE DUPLICATE_CLOSE_SOURCE
|
|
#define DUP_HANDLE_SAME_ACCESS DUPLICATE_SAME_ACCESS
|
|
#define DUP_HANDLE_MAKE_GLOBAL 0x80000000 /* Not a Windows flag */
|
|
|
|
|
|
/* Open a handle to a process */
|
|
@REQ(open_process)
|
|
process_id_t pid; /* process id to open */
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the process */
|
|
@END
|
|
|
|
|
|
/* Open a handle to a thread */
|
|
@REQ(open_thread)
|
|
thread_id_t tid; /* thread id to open */
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the thread */
|
|
@END
|
|
|
|
|
|
/* Wait for handles */
|
|
@REQ(select)
|
|
int flags; /* wait flags (see below) */
|
|
void* cookie; /* magic cookie to return to client */
|
|
obj_handle_t signal; /* object to signal (0 if none) */
|
|
obj_handle_t prev_apc; /* handle to previous APC */
|
|
timeout_t timeout; /* timeout */
|
|
VARARG(result,apc_result); /* result of previous APC */
|
|
VARARG(handles,handles); /* handles to select on */
|
|
@REPLY
|
|
obj_handle_t apc_handle; /* handle to next APC */
|
|
timeout_t timeout; /* timeout converted to absolute */
|
|
apc_call_t call; /* APC call arguments */
|
|
@END
|
|
#define SELECT_ALL 1
|
|
#define SELECT_ALERTABLE 2
|
|
#define SELECT_INTERRUPTIBLE 4
|
|
|
|
|
|
/* Create an event */
|
|
@REQ(create_event)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
int manual_reset; /* manual reset event */
|
|
int initial_state; /* initial state of the event */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the event */
|
|
@END
|
|
|
|
/* Event operation */
|
|
@REQ(event_op)
|
|
obj_handle_t handle; /* handle to event */
|
|
int op; /* event operation (see below) */
|
|
@END
|
|
enum event_op { PULSE_EVENT, SET_EVENT, RESET_EVENT };
|
|
|
|
|
|
/* Open an event */
|
|
@REQ(open_event)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the event */
|
|
@END
|
|
|
|
|
|
/* Create a mutex */
|
|
@REQ(create_mutex)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
int owned; /* initially owned? */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the mutex */
|
|
@END
|
|
|
|
|
|
/* Release a mutex */
|
|
@REQ(release_mutex)
|
|
obj_handle_t handle; /* handle to the mutex */
|
|
@REPLY
|
|
unsigned int prev_count; /* value of internal counter, before release */
|
|
@END
|
|
|
|
|
|
/* Open a mutex */
|
|
@REQ(open_mutex)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the mutex */
|
|
@END
|
|
|
|
|
|
/* Create a semaphore */
|
|
@REQ(create_semaphore)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
unsigned int initial; /* initial count */
|
|
unsigned int max; /* maximum count */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the semaphore */
|
|
@END
|
|
|
|
|
|
/* Release a semaphore */
|
|
@REQ(release_semaphore)
|
|
obj_handle_t handle; /* handle to the semaphore */
|
|
unsigned int count; /* count to add to semaphore */
|
|
@REPLY
|
|
unsigned int prev_count; /* previous semaphore count */
|
|
@END
|
|
|
|
|
|
/* Open a semaphore */
|
|
@REQ(open_semaphore)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the semaphore */
|
|
@END
|
|
|
|
|
|
/* Create a file */
|
|
@REQ(create_file)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
unsigned int sharing; /* sharing flags */
|
|
int create; /* file create action */
|
|
unsigned int options; /* file options */
|
|
unsigned int attrs; /* file attributes for creation */
|
|
VARARG(filename,string); /* file name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the file */
|
|
@END
|
|
|
|
|
|
/* Open a file object */
|
|
@REQ(open_file_object)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* open attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
unsigned int sharing; /* sharing flags */
|
|
unsigned int options; /* file options */
|
|
VARARG(filename,unicode_str); /* file name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the file */
|
|
@END
|
|
|
|
|
|
/* Allocate a file handle for a Unix fd */
|
|
@REQ(alloc_file_handle)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
int fd; /* file descriptor on the client side */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the file */
|
|
@END
|
|
|
|
|
|
/* Get a Unix fd to access a file */
|
|
@REQ(get_handle_fd)
|
|
obj_handle_t handle; /* handle to the file */
|
|
@REPLY
|
|
int type; /* file type (see below) */
|
|
int removable; /* is file removable? */
|
|
unsigned int access; /* file access rights */
|
|
unsigned int options; /* file open options */
|
|
@END
|
|
enum server_fd_type
|
|
{
|
|
FD_TYPE_INVALID, /* invalid file (no associated fd) */
|
|
FD_TYPE_FILE, /* regular file */
|
|
FD_TYPE_DIR, /* directory */
|
|
FD_TYPE_SOCKET, /* socket */
|
|
FD_TYPE_SERIAL, /* serial port */
|
|
FD_TYPE_PIPE, /* named pipe */
|
|
FD_TYPE_MAILSLOT, /* mailslot */
|
|
FD_TYPE_CHAR, /* unspecified char device */
|
|
FD_TYPE_DEVICE, /* Windows device file */
|
|
FD_TYPE_NB_TYPES
|
|
};
|
|
|
|
|
|
/* Flush a file buffers */
|
|
@REQ(flush_file)
|
|
obj_handle_t handle; /* handle to the file */
|
|
@REPLY
|
|
obj_handle_t event; /* event set when finished */
|
|
@END
|
|
|
|
|
|
/* Lock a region of a file */
|
|
@REQ(lock_file)
|
|
obj_handle_t handle; /* handle to the file */
|
|
unsigned int offset_low; /* offset of start of lock */
|
|
unsigned int offset_high; /* offset of start of lock */
|
|
unsigned int count_low; /* count of bytes to lock */
|
|
unsigned int count_high; /* count of bytes to lock */
|
|
int shared; /* shared or exclusive lock? */
|
|
int wait; /* do we want to wait? */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to wait on */
|
|
int overlapped; /* is it an overlapped I/O handle? */
|
|
@END
|
|
|
|
|
|
/* Unlock a region of a file */
|
|
@REQ(unlock_file)
|
|
obj_handle_t handle; /* handle to the file */
|
|
unsigned int offset_low; /* offset of start of unlock */
|
|
unsigned int offset_high; /* offset of start of unlock */
|
|
unsigned int count_low; /* count of bytes to unlock */
|
|
unsigned int count_high; /* count of bytes to unlock */
|
|
@END
|
|
|
|
|
|
/* Create a socket */
|
|
@REQ(create_socket)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
int family; /* family, see socket manpage */
|
|
int type; /* type, see socket manpage */
|
|
int protocol; /* protocol, see socket manpage */
|
|
unsigned int flags; /* socket flags */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the new socket */
|
|
@END
|
|
|
|
|
|
/* Accept a socket */
|
|
@REQ(accept_socket)
|
|
obj_handle_t lhandle; /* handle to the listening socket */
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the new socket */
|
|
@END
|
|
|
|
|
|
/* Set socket event parameters */
|
|
@REQ(set_socket_event)
|
|
obj_handle_t handle; /* handle to the socket */
|
|
unsigned int mask; /* event mask */
|
|
obj_handle_t event; /* event object */
|
|
user_handle_t window; /* window to send the message to */
|
|
unsigned int msg; /* message to send */
|
|
@END
|
|
|
|
|
|
/* Get socket event parameters */
|
|
@REQ(get_socket_event)
|
|
obj_handle_t handle; /* handle to the socket */
|
|
int service; /* clear pending? */
|
|
obj_handle_t c_event; /* event to clear */
|
|
@REPLY
|
|
unsigned int mask; /* event mask */
|
|
unsigned int pmask; /* pending events */
|
|
unsigned int state; /* status bits */
|
|
VARARG(errors,ints); /* event errors */
|
|
@END
|
|
|
|
|
|
/* Reenable pending socket events */
|
|
@REQ(enable_socket_event)
|
|
obj_handle_t handle; /* handle to the socket */
|
|
unsigned int mask; /* events to re-enable */
|
|
unsigned int sstate; /* status bits to set */
|
|
unsigned int cstate; /* status bits to clear */
|
|
@END
|
|
|
|
@REQ(set_socket_deferred)
|
|
obj_handle_t handle; /* handle to the socket */
|
|
obj_handle_t deferred; /* handle to the socket for which accept() is deferred */
|
|
@END
|
|
|
|
/* Allocate a console (only used by a console renderer) */
|
|
@REQ(alloc_console)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
process_id_t pid; /* pid of process which shall be attached to the console */
|
|
@REPLY
|
|
obj_handle_t handle_in; /* handle to console input */
|
|
obj_handle_t event; /* handle to renderer events change notification */
|
|
@END
|
|
|
|
|
|
/* Free the console of the current process */
|
|
@REQ(free_console)
|
|
@END
|
|
|
|
|
|
#define CONSOLE_RENDERER_NONE_EVENT 0x00
|
|
#define CONSOLE_RENDERER_TITLE_EVENT 0x01
|
|
#define CONSOLE_RENDERER_ACTIVE_SB_EVENT 0x02
|
|
#define CONSOLE_RENDERER_SB_RESIZE_EVENT 0x03
|
|
#define CONSOLE_RENDERER_UPDATE_EVENT 0x04
|
|
#define CONSOLE_RENDERER_CURSOR_POS_EVENT 0x05
|
|
#define CONSOLE_RENDERER_CURSOR_GEOM_EVENT 0x06
|
|
#define CONSOLE_RENDERER_DISPLAY_EVENT 0x07
|
|
#define CONSOLE_RENDERER_EXIT_EVENT 0x08
|
|
struct console_renderer_event
|
|
{
|
|
short event;
|
|
union
|
|
{
|
|
struct update
|
|
{
|
|
short top;
|
|
short bottom;
|
|
} update;
|
|
struct resize
|
|
{
|
|
short width;
|
|
short height;
|
|
} resize;
|
|
struct cursor_pos
|
|
{
|
|
short x;
|
|
short y;
|
|
} cursor_pos;
|
|
struct cursor_geom
|
|
{
|
|
short visible;
|
|
short size;
|
|
} cursor_geom;
|
|
struct display
|
|
{
|
|
short left;
|
|
short top;
|
|
short width;
|
|
short height;
|
|
} display;
|
|
} u;
|
|
};
|
|
|
|
/* retrieve console events for the renderer */
|
|
@REQ(get_console_renderer_events)
|
|
obj_handle_t handle; /* handle to console input events */
|
|
@REPLY
|
|
VARARG(data,bytes); /* the various console_renderer_events */
|
|
@END
|
|
|
|
|
|
/* Open a handle to the process console */
|
|
@REQ(open_console)
|
|
obj_handle_t from; /* 0 (resp 1) input (resp output) of current process console */
|
|
/* otherwise console_in handle to get active screen buffer? */
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
int share; /* share mask (only for output handles) */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the console */
|
|
@END
|
|
|
|
|
|
/* Get the input queue wait event */
|
|
@REQ(get_console_wait_event)
|
|
@REPLY
|
|
obj_handle_t handle;
|
|
@END
|
|
|
|
/* Get a console mode (input or output) */
|
|
@REQ(get_console_mode)
|
|
obj_handle_t handle; /* handle to the console */
|
|
@REPLY
|
|
int mode; /* console mode */
|
|
@END
|
|
|
|
|
|
/* Set a console mode (input or output) */
|
|
@REQ(set_console_mode)
|
|
obj_handle_t handle; /* handle to the console */
|
|
int mode; /* console mode */
|
|
@END
|
|
|
|
|
|
/* Set info about a console (input only) */
|
|
@REQ(set_console_input_info)
|
|
obj_handle_t handle; /* handle to console input, or 0 for process' console */
|
|
int mask; /* setting mask (see below) */
|
|
obj_handle_t active_sb; /* active screen buffer */
|
|
int history_mode; /* whether we duplicate lines in history */
|
|
int history_size; /* number of lines in history */
|
|
int edition_mode; /* index to the edition mode flavors */
|
|
int input_cp; /* console input codepage */
|
|
int output_cp; /* console output codepage */
|
|
VARARG(title,unicode_str); /* console title */
|
|
@END
|
|
#define SET_CONSOLE_INPUT_INFO_ACTIVE_SB 0x01
|
|
#define SET_CONSOLE_INPUT_INFO_TITLE 0x02
|
|
#define SET_CONSOLE_INPUT_INFO_HISTORY_MODE 0x04
|
|
#define SET_CONSOLE_INPUT_INFO_HISTORY_SIZE 0x08
|
|
#define SET_CONSOLE_INPUT_INFO_EDITION_MODE 0x10
|
|
#define SET_CONSOLE_INPUT_INFO_INPUT_CODEPAGE 0x20
|
|
#define SET_CONSOLE_INPUT_INFO_OUTPUT_CODEPAGE 0x40
|
|
|
|
|
|
/* Get info about a console (input only) */
|
|
@REQ(get_console_input_info)
|
|
obj_handle_t handle; /* handle to console input, or 0 for process' console */
|
|
@REPLY
|
|
int history_mode; /* whether we duplicate lines in history */
|
|
int history_size; /* number of lines in history */
|
|
int history_index; /* number of used lines in history */
|
|
int edition_mode; /* index to the edition mode flavors */
|
|
int input_cp; /* console input codepage */
|
|
int output_cp; /* console output codepage */
|
|
VARARG(title,unicode_str); /* console title */
|
|
@END
|
|
|
|
|
|
/* appends a string to console's history */
|
|
@REQ(append_console_input_history)
|
|
obj_handle_t handle; /* handle to console input, or 0 for process' console */
|
|
VARARG(line,unicode_str); /* line to add */
|
|
@END
|
|
|
|
|
|
/* appends a string to console's history */
|
|
@REQ(get_console_input_history)
|
|
obj_handle_t handle; /* handle to console input, or 0 for process' console */
|
|
int index; /* index to get line from */
|
|
@REPLY
|
|
int total; /* total length of line in Unicode chars */
|
|
VARARG(line,unicode_str); /* line to add */
|
|
@END
|
|
|
|
|
|
/* creates a new screen buffer on process' console */
|
|
@REQ(create_console_output)
|
|
obj_handle_t handle_in; /* handle to console input, or 0 for process' console */
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
unsigned int share; /* sharing credentials */
|
|
@REPLY
|
|
obj_handle_t handle_out; /* handle to the screen buffer */
|
|
@END
|
|
|
|
|
|
/* Set info about a console (output only) */
|
|
@REQ(set_console_output_info)
|
|
obj_handle_t handle; /* handle to the console */
|
|
int mask; /* setting mask (see below) */
|
|
short int cursor_size; /* size of cursor (percentage filled) */
|
|
short int cursor_visible;/* cursor visibility flag */
|
|
short int cursor_x; /* position of cursor (x, y) */
|
|
short int cursor_y;
|
|
short int width; /* width of the screen buffer */
|
|
short int height; /* height of the screen buffer */
|
|
short int attr; /* default attribute */
|
|
short int win_left; /* window actually displayed by renderer */
|
|
short int win_top; /* the rect area is expressed withing the */
|
|
short int win_right; /* boundaries of the screen buffer */
|
|
short int win_bottom;
|
|
short int max_width; /* maximum size (width x height) for the window */
|
|
short int max_height;
|
|
@END
|
|
#define SET_CONSOLE_OUTPUT_INFO_CURSOR_GEOM 0x01
|
|
#define SET_CONSOLE_OUTPUT_INFO_CURSOR_POS 0x02
|
|
#define SET_CONSOLE_OUTPUT_INFO_SIZE 0x04
|
|
#define SET_CONSOLE_OUTPUT_INFO_ATTR 0x08
|
|
#define SET_CONSOLE_OUTPUT_INFO_DISPLAY_WINDOW 0x10
|
|
#define SET_CONSOLE_OUTPUT_INFO_MAX_SIZE 0x20
|
|
|
|
|
|
/* Get info about a console (output only) */
|
|
@REQ(get_console_output_info)
|
|
obj_handle_t handle; /* handle to the console */
|
|
@REPLY
|
|
short int cursor_size; /* size of cursor (percentage filled) */
|
|
short int cursor_visible;/* cursor visibility flag */
|
|
short int cursor_x; /* position of cursor (x, y) */
|
|
short int cursor_y;
|
|
short int width; /* width of the screen buffer */
|
|
short int height; /* height of the screen buffer */
|
|
short int attr; /* default attribute */
|
|
short int win_left; /* window actually displayed by renderer */
|
|
short int win_top; /* the rect area is expressed withing the */
|
|
short int win_right; /* boundaries of the screen buffer */
|
|
short int win_bottom;
|
|
short int max_width; /* maximum size (width x height) for the window */
|
|
short int max_height;
|
|
@END
|
|
|
|
/* Add input records to a console input queue */
|
|
@REQ(write_console_input)
|
|
obj_handle_t handle; /* handle to the console input */
|
|
VARARG(rec,input_records); /* input records */
|
|
@REPLY
|
|
int written; /* number of records written */
|
|
@END
|
|
|
|
|
|
/* Fetch input records from a console input queue */
|
|
@REQ(read_console_input)
|
|
obj_handle_t handle; /* handle to the console input */
|
|
int flush; /* flush the retrieved records from the queue? */
|
|
@REPLY
|
|
int read; /* number of records read */
|
|
VARARG(rec,input_records); /* input records */
|
|
@END
|
|
|
|
|
|
/* write data (chars and/or attributes) in a screen buffer */
|
|
@REQ(write_console_output)
|
|
obj_handle_t handle; /* handle to the console output */
|
|
int x; /* position where to start writing */
|
|
int y;
|
|
int mode; /* char info (see below) */
|
|
int wrap; /* wrap around at end of line? */
|
|
VARARG(data,bytes); /* info to write */
|
|
@REPLY
|
|
int written; /* number of char infos actually written */
|
|
int width; /* width of screen buffer */
|
|
int height; /* height of screen buffer */
|
|
@END
|
|
enum char_info_mode
|
|
{
|
|
CHAR_INFO_MODE_TEXT, /* characters only */
|
|
CHAR_INFO_MODE_ATTR, /* attributes only */
|
|
CHAR_INFO_MODE_TEXTATTR, /* both characters and attributes */
|
|
CHAR_INFO_MODE_TEXTSTDATTR /* characters but use standard attributes */
|
|
};
|
|
|
|
|
|
/* fill a screen buffer with constant data (chars and/or attributes) */
|
|
@REQ(fill_console_output)
|
|
obj_handle_t handle; /* handle to the console output */
|
|
int x; /* position where to start writing */
|
|
int y;
|
|
int mode; /* char info mode */
|
|
int count; /* number to write */
|
|
int wrap; /* wrap around at end of line? */
|
|
char_info_t data; /* data to write */
|
|
@REPLY
|
|
int written; /* number of char infos actually written */
|
|
@END
|
|
|
|
|
|
/* read data (chars and/or attributes) from a screen buffer */
|
|
@REQ(read_console_output)
|
|
obj_handle_t handle; /* handle to the console output */
|
|
int x; /* position (x,y) where to start reading */
|
|
int y;
|
|
int mode; /* char info mode */
|
|
int wrap; /* wrap around at end of line? */
|
|
@REPLY
|
|
int width; /* width of screen buffer */
|
|
int height; /* height of screen buffer */
|
|
VARARG(data,bytes);
|
|
@END
|
|
|
|
|
|
/* move a rect (of data) in screen buffer content */
|
|
@REQ(move_console_output)
|
|
obj_handle_t handle; /* handle to the console output */
|
|
short int x_src; /* position (x, y) of rect to start moving from */
|
|
short int y_src;
|
|
short int x_dst; /* position (x, y) of rect to move to */
|
|
short int y_dst;
|
|
short int w; /* size of the rect (width, height) to move */
|
|
short int h;
|
|
@END
|
|
|
|
|
|
/* Sends a signal to a process group */
|
|
@REQ(send_console_signal)
|
|
int signal; /* the signal to send */
|
|
process_id_t group_id; /* the group to send the signal to */
|
|
@END
|
|
|
|
|
|
/* enable directory change notifications */
|
|
@REQ(read_directory_changes)
|
|
unsigned int filter; /* notification filter */
|
|
obj_handle_t handle; /* handle to the directory */
|
|
int subtree; /* watch the subtree? */
|
|
int want_data; /* flag indicating whether change data should be collected */
|
|
async_data_t async; /* async I/O parameters */
|
|
@END
|
|
|
|
|
|
@REQ(read_change)
|
|
obj_handle_t handle;
|
|
@REPLY
|
|
int action; /* type of change */
|
|
VARARG(name,string); /* name of directory entry that changed */
|
|
@END
|
|
|
|
|
|
/* Create a file mapping */
|
|
@REQ(create_mapping)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
int size_high; /* mapping size */
|
|
int size_low; /* mapping size */
|
|
int protect; /* protection flags (see below) */
|
|
obj_handle_t file_handle; /* file handle */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the mapping */
|
|
@END
|
|
/* protection flags */
|
|
#define VPROT_READ 0x01
|
|
#define VPROT_WRITE 0x02
|
|
#define VPROT_EXEC 0x04
|
|
#define VPROT_WRITECOPY 0x08
|
|
#define VPROT_GUARD 0x10
|
|
#define VPROT_NOCACHE 0x20
|
|
#define VPROT_COMMITTED 0x40
|
|
#define VPROT_IMAGE 0x80
|
|
|
|
|
|
/* Open a mapping */
|
|
@REQ(open_mapping)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the mapping */
|
|
@END
|
|
|
|
|
|
/* Get information about a file mapping */
|
|
@REQ(get_mapping_info)
|
|
obj_handle_t handle; /* handle to the mapping */
|
|
@REPLY
|
|
int size_high; /* mapping size */
|
|
int size_low; /* mapping size */
|
|
int protect; /* protection flags */
|
|
int header_size; /* header size (for VPROT_IMAGE mapping) */
|
|
void* base; /* default base addr (for VPROT_IMAGE mapping) */
|
|
obj_handle_t mapping; /* duplicate mapping handle unless removable */
|
|
obj_handle_t shared_file; /* shared mapping file handle */
|
|
int shared_size; /* shared mapping size */
|
|
@END
|
|
|
|
|
|
#define SNAP_HEAPLIST 0x00000001
|
|
#define SNAP_PROCESS 0x00000002
|
|
#define SNAP_THREAD 0x00000004
|
|
#define SNAP_MODULE 0x00000008
|
|
/* Create a snapshot */
|
|
@REQ(create_snapshot)
|
|
unsigned int attributes; /* object attributes */
|
|
int flags; /* snapshot flags (SNAP_*) */
|
|
process_id_t pid; /* process id */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the snapshot */
|
|
@END
|
|
|
|
|
|
/* Get the next process from a snapshot */
|
|
@REQ(next_process)
|
|
obj_handle_t handle; /* handle to the snapshot */
|
|
int reset; /* reset snapshot position? */
|
|
@REPLY
|
|
int count; /* process usage count */
|
|
process_id_t pid; /* process id */
|
|
process_id_t ppid; /* parent process id */
|
|
void* heap; /* heap base */
|
|
void* module; /* main module */
|
|
int threads; /* number of threads */
|
|
int priority; /* process priority */
|
|
int handles; /* number of handles */
|
|
VARARG(filename,unicode_str); /* file name of main exe */
|
|
@END
|
|
|
|
|
|
/* Get the next thread from a snapshot */
|
|
@REQ(next_thread)
|
|
obj_handle_t handle; /* handle to the snapshot */
|
|
int reset; /* reset snapshot position? */
|
|
@REPLY
|
|
int count; /* thread usage count */
|
|
process_id_t pid; /* process id */
|
|
thread_id_t tid; /* thread id */
|
|
int base_pri; /* base priority */
|
|
int delta_pri; /* delta priority */
|
|
@END
|
|
|
|
|
|
/* Get the next module from a snapshot */
|
|
@REQ(next_module)
|
|
obj_handle_t handle; /* handle to the snapshot */
|
|
int reset; /* reset snapshot position? */
|
|
@REPLY
|
|
process_id_t pid; /* process id */
|
|
void* base; /* module base address */
|
|
size_t size; /* module size */
|
|
VARARG(filename,unicode_str); /* file name of module */
|
|
@END
|
|
|
|
|
|
/* Wait for a debug event */
|
|
@REQ(wait_debug_event)
|
|
int get_handle; /* should we alloc a handle for waiting? */
|
|
@REPLY
|
|
process_id_t pid; /* process id */
|
|
thread_id_t tid; /* thread id */
|
|
obj_handle_t wait; /* wait handle if no event ready */
|
|
VARARG(event,debug_event); /* debug event data */
|
|
@END
|
|
|
|
|
|
/* Queue an exception event */
|
|
@REQ(queue_exception_event)
|
|
int first; /* first chance exception? */
|
|
VARARG(record,exc_event); /* thread context followed by exception record */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the queued event */
|
|
@END
|
|
|
|
|
|
/* Retrieve the status of an exception event */
|
|
@REQ(get_exception_status)
|
|
obj_handle_t handle; /* handle to the queued event */
|
|
@REPLY
|
|
VARARG(context,context); /* modified thread context */
|
|
@END
|
|
|
|
|
|
/* Send an output string to the debugger */
|
|
@REQ(output_debug_string)
|
|
void* string; /* string to display (in debugged process address space) */
|
|
int unicode; /* is it Unicode? */
|
|
int length; /* string length */
|
|
@END
|
|
|
|
|
|
/* Continue a debug event */
|
|
@REQ(continue_debug_event)
|
|
process_id_t pid; /* process id to continue */
|
|
thread_id_t tid; /* thread id to continue */
|
|
int status; /* continuation status */
|
|
@END
|
|
|
|
|
|
/* Start/stop debugging an existing process */
|
|
@REQ(debug_process)
|
|
process_id_t pid; /* id of the process to debug */
|
|
int attach; /* 1=attaching / 0=detaching from the process */
|
|
@END
|
|
|
|
|
|
/* Simulate a breakpoint in a process */
|
|
@REQ(debug_break)
|
|
obj_handle_t handle; /* process handle */
|
|
@REPLY
|
|
int self; /* was it the caller itself? */
|
|
@END
|
|
|
|
|
|
/* Set debugger kill on exit flag */
|
|
@REQ(set_debugger_kill_on_exit)
|
|
int kill_on_exit; /* 0=detach/1=kill debuggee when debugger dies */
|
|
@END
|
|
|
|
|
|
/* Read data from a process address space */
|
|
@REQ(read_process_memory)
|
|
obj_handle_t handle; /* process handle */
|
|
void* addr; /* addr to read from */
|
|
@REPLY
|
|
VARARG(data,bytes); /* result data */
|
|
@END
|
|
|
|
|
|
/* Write data to a process address space */
|
|
@REQ(write_process_memory)
|
|
obj_handle_t handle; /* process handle */
|
|
void* addr; /* addr to write to */
|
|
VARARG(data,bytes); /* data to write */
|
|
@END
|
|
|
|
|
|
/* Create a registry key */
|
|
@REQ(create_key)
|
|
obj_handle_t parent; /* handle to the parent key */
|
|
unsigned int access; /* desired access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
unsigned int options; /* creation options */
|
|
time_t modif; /* last modification time */
|
|
data_size_t namelen; /* length of key name in bytes */
|
|
VARARG(name,unicode_str,namelen); /* key name */
|
|
VARARG(class,unicode_str); /* class name */
|
|
@REPLY
|
|
obj_handle_t hkey; /* handle to the created key */
|
|
int created; /* has it been newly created? */
|
|
@END
|
|
|
|
/* Open a registry key */
|
|
@REQ(open_key)
|
|
obj_handle_t parent; /* handle to the parent key */
|
|
unsigned int access; /* desired access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
VARARG(name,unicode_str); /* key name */
|
|
@REPLY
|
|
obj_handle_t hkey; /* handle to the open key */
|
|
@END
|
|
|
|
|
|
/* Delete a registry key */
|
|
@REQ(delete_key)
|
|
obj_handle_t hkey; /* handle to the key */
|
|
@END
|
|
|
|
|
|
/* Flush a registry key */
|
|
@REQ(flush_key)
|
|
obj_handle_t hkey; /* handle to the key */
|
|
@END
|
|
|
|
|
|
/* Enumerate registry subkeys */
|
|
@REQ(enum_key)
|
|
obj_handle_t hkey; /* handle to registry key */
|
|
int index; /* index of subkey (or -1 for current key) */
|
|
int info_class; /* requested information class */
|
|
@REPLY
|
|
int subkeys; /* number of subkeys */
|
|
int max_subkey; /* longest subkey name */
|
|
int max_class; /* longest class name */
|
|
int values; /* number of values */
|
|
int max_value; /* longest value name */
|
|
int max_data; /* longest value data */
|
|
time_t modif; /* last modification time */
|
|
data_size_t total; /* total length needed for full name and class */
|
|
data_size_t namelen; /* length of key name in bytes */
|
|
VARARG(name,unicode_str,namelen); /* key name */
|
|
VARARG(class,unicode_str); /* class name */
|
|
@END
|
|
|
|
|
|
/* Set a value of a registry key */
|
|
@REQ(set_key_value)
|
|
obj_handle_t hkey; /* handle to registry key */
|
|
int type; /* value type */
|
|
data_size_t namelen; /* length of value name in bytes */
|
|
VARARG(name,unicode_str,namelen); /* value name */
|
|
VARARG(data,bytes); /* value data */
|
|
@END
|
|
|
|
|
|
/* Retrieve the value of a registry key */
|
|
@REQ(get_key_value)
|
|
obj_handle_t hkey; /* handle to registry key */
|
|
VARARG(name,unicode_str); /* value name */
|
|
@REPLY
|
|
int type; /* value type */
|
|
data_size_t total; /* total length needed for data */
|
|
VARARG(data,bytes); /* value data */
|
|
@END
|
|
|
|
|
|
/* Enumerate a value of a registry key */
|
|
@REQ(enum_key_value)
|
|
obj_handle_t hkey; /* handle to registry key */
|
|
int index; /* value index */
|
|
int info_class; /* requested information class */
|
|
@REPLY
|
|
int type; /* value type */
|
|
data_size_t total; /* total length needed for full name and data */
|
|
data_size_t namelen; /* length of value name in bytes */
|
|
VARARG(name,unicode_str,namelen); /* value name */
|
|
VARARG(data,bytes); /* value data */
|
|
@END
|
|
|
|
|
|
/* Delete a value of a registry key */
|
|
@REQ(delete_key_value)
|
|
obj_handle_t hkey; /* handle to registry key */
|
|
VARARG(name,unicode_str); /* value name */
|
|
@END
|
|
|
|
|
|
/* Load a registry branch from a file */
|
|
@REQ(load_registry)
|
|
obj_handle_t hkey; /* root key to load to */
|
|
obj_handle_t file; /* file to load from */
|
|
VARARG(name,unicode_str); /* subkey name */
|
|
@END
|
|
|
|
|
|
/* UnLoad a registry branch from a file */
|
|
@REQ(unload_registry)
|
|
obj_handle_t hkey; /* root key to unload to */
|
|
@END
|
|
|
|
|
|
/* Save a registry branch to a file */
|
|
@REQ(save_registry)
|
|
obj_handle_t hkey; /* key to save */
|
|
obj_handle_t file; /* file to save to */
|
|
@END
|
|
|
|
|
|
/* Add a registry key change notification */
|
|
@REQ(set_registry_notification)
|
|
obj_handle_t hkey; /* key to watch for changes */
|
|
obj_handle_t event; /* event to set */
|
|
int subtree; /* should we watch the whole subtree? */
|
|
unsigned int filter; /* things to watch */
|
|
@END
|
|
|
|
|
|
/* Create a waitable timer */
|
|
@REQ(create_timer)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
int manual; /* manual reset */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the timer */
|
|
@END
|
|
|
|
|
|
/* Open a waitable timer */
|
|
@REQ(open_timer)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the timer */
|
|
@END
|
|
|
|
/* Set a waitable timer */
|
|
@REQ(set_timer)
|
|
obj_handle_t handle; /* handle to the timer */
|
|
timeout_t expire; /* next expiration absolute time */
|
|
int period; /* timer period in ms */
|
|
void* callback; /* callback function */
|
|
void* arg; /* callback argument */
|
|
@REPLY
|
|
int signaled; /* was the timer signaled before this call ? */
|
|
@END
|
|
|
|
/* Cancel a waitable timer */
|
|
@REQ(cancel_timer)
|
|
obj_handle_t handle; /* handle to the timer */
|
|
@REPLY
|
|
int signaled; /* was the timer signaled before this calltime ? */
|
|
@END
|
|
|
|
/* Get information on a waitable timer */
|
|
@REQ(get_timer_info)
|
|
obj_handle_t handle; /* handle to the timer */
|
|
@REPLY
|
|
timeout_t when; /* absolute time when the timer next expires */
|
|
int signaled; /* is the timer signaled? */
|
|
@END
|
|
|
|
|
|
/* Retrieve the current context of a thread */
|
|
@REQ(get_thread_context)
|
|
obj_handle_t handle; /* thread handle */
|
|
unsigned int flags; /* context flags */
|
|
int suspend; /* if getting context during suspend */
|
|
@REPLY
|
|
int self; /* was it a handle to the current thread? */
|
|
VARARG(context,context); /* thread context */
|
|
@END
|
|
|
|
|
|
/* Set the current context of a thread */
|
|
@REQ(set_thread_context)
|
|
obj_handle_t handle; /* thread handle */
|
|
unsigned int flags; /* context flags */
|
|
int suspend; /* if setting context during suspend */
|
|
VARARG(context,context); /* thread context */
|
|
@REPLY
|
|
int self; /* was it a handle to the current thread? */
|
|
@END
|
|
|
|
|
|
/* Fetch a selector entry for a thread */
|
|
@REQ(get_selector_entry)
|
|
obj_handle_t handle; /* thread handle */
|
|
int entry; /* LDT entry */
|
|
@REPLY
|
|
unsigned int base; /* selector base */
|
|
unsigned int limit; /* selector limit */
|
|
unsigned char flags; /* selector flags */
|
|
@END
|
|
|
|
|
|
/* Add an atom */
|
|
@REQ(add_atom)
|
|
obj_handle_t table; /* which table to add atom to */
|
|
VARARG(name,unicode_str); /* atom name */
|
|
@REPLY
|
|
atom_t atom; /* resulting atom */
|
|
@END
|
|
|
|
|
|
/* Delete an atom */
|
|
@REQ(delete_atom)
|
|
obj_handle_t table; /* which table to delete atom from */
|
|
atom_t atom; /* atom handle */
|
|
@END
|
|
|
|
|
|
/* Find an atom */
|
|
@REQ(find_atom)
|
|
obj_handle_t table; /* which table to find atom from */
|
|
VARARG(name,unicode_str); /* atom name */
|
|
@REPLY
|
|
atom_t atom; /* atom handle */
|
|
@END
|
|
|
|
|
|
/* Get information about an atom */
|
|
@REQ(get_atom_information)
|
|
obj_handle_t table; /* which table to find atom from */
|
|
atom_t atom; /* atom handle */
|
|
@REPLY
|
|
int count; /* atom lock count */
|
|
int pinned; /* whether the atom has been pinned */
|
|
data_size_t total; /* actual length of atom name */
|
|
VARARG(name,unicode_str); /* atom name */
|
|
@END
|
|
|
|
|
|
/* Set information about an atom */
|
|
@REQ(set_atom_information)
|
|
obj_handle_t table; /* which table to find atom from */
|
|
atom_t atom; /* atom handle */
|
|
int pinned; /* whether to bump atom information */
|
|
@END
|
|
|
|
|
|
/* Empty an atom table */
|
|
@REQ(empty_atom_table)
|
|
obj_handle_t table; /* which table to find atom from */
|
|
int if_pinned; /* whether to delete pinned atoms */
|
|
@END
|
|
|
|
|
|
/* Init an atom table */
|
|
@REQ(init_atom_table)
|
|
int entries; /* number of entries (only for local) */
|
|
@REPLY
|
|
obj_handle_t table; /* handle to the atom table */
|
|
@END
|
|
|
|
|
|
/* Get the message queue of the current thread */
|
|
@REQ(get_msg_queue)
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the queue */
|
|
@END
|
|
|
|
|
|
/* Set the file descriptor associated to the current thread queue */
|
|
@REQ(set_queue_fd)
|
|
obj_handle_t handle; /* handle to the file descriptor */
|
|
@END
|
|
|
|
|
|
/* Set the current message queue wakeup mask */
|
|
@REQ(set_queue_mask)
|
|
unsigned int wake_mask; /* wakeup bits mask */
|
|
unsigned int changed_mask; /* changed bits mask */
|
|
int skip_wait; /* will we skip waiting if signaled? */
|
|
@REPLY
|
|
unsigned int wake_bits; /* current wake bits */
|
|
unsigned int changed_bits; /* current changed bits */
|
|
@END
|
|
|
|
|
|
/* Get the current message queue status */
|
|
@REQ(get_queue_status)
|
|
int clear; /* should we clear the change bits? */
|
|
@REPLY
|
|
unsigned int wake_bits; /* wake bits */
|
|
unsigned int changed_bits; /* changed bits since last time */
|
|
@END
|
|
|
|
|
|
/* Retrieve the process idle event */
|
|
@REQ(get_process_idle_event)
|
|
obj_handle_t handle; /* process handle */
|
|
@REPLY
|
|
obj_handle_t event; /* handle to idle event */
|
|
@END
|
|
|
|
|
|
/* Send a message to a thread queue */
|
|
@REQ(send_message)
|
|
thread_id_t id; /* thread id */
|
|
int type; /* message type (see below) */
|
|
int flags; /* message flags (see below) */
|
|
user_handle_t win; /* window handle */
|
|
unsigned int msg; /* message code */
|
|
unsigned long wparam; /* parameters */
|
|
unsigned long lparam; /* parameters */
|
|
timeout_t timeout; /* timeout for reply */
|
|
VARARG(data,message_data); /* message data for sent messages */
|
|
@END
|
|
|
|
@REQ(post_quit_message)
|
|
int exit_code; /* exit code to return */
|
|
@END
|
|
|
|
enum message_type
|
|
{
|
|
MSG_ASCII, /* Ascii message (from SendMessageA) */
|
|
MSG_UNICODE, /* Unicode message (from SendMessageW) */
|
|
MSG_NOTIFY, /* notify message (from SendNotifyMessageW), always Unicode */
|
|
MSG_CALLBACK, /* callback message (from SendMessageCallbackW), always Unicode */
|
|
MSG_CALLBACK_RESULT,/* result of a callback message */
|
|
MSG_OTHER_PROCESS, /* sent from other process, may include vararg data, always Unicode */
|
|
MSG_POSTED, /* posted message (from PostMessageW), always Unicode */
|
|
MSG_HARDWARE, /* hardware message */
|
|
MSG_WINEVENT /* winevent message */
|
|
};
|
|
#define SEND_MSG_ABORT_IF_HUNG 0x01
|
|
|
|
|
|
/* Send a hardware message to a thread queue */
|
|
@REQ(send_hardware_message)
|
|
thread_id_t id; /* thread id */
|
|
user_handle_t win; /* window handle */
|
|
unsigned int msg; /* message code */
|
|
unsigned int time; /* message time */
|
|
unsigned long wparam; /* parameters */
|
|
unsigned long lparam; /* parameters */
|
|
unsigned long info; /* extra info */
|
|
int x; /* x position */
|
|
int y; /* y position */
|
|
@END
|
|
|
|
|
|
/* Get a message from the current queue */
|
|
@REQ(get_message)
|
|
unsigned int flags; /* PM_* flags */
|
|
user_handle_t get_win; /* window handle to get */
|
|
unsigned int get_first; /* first message code to get */
|
|
unsigned int get_last; /* last message code to get */
|
|
unsigned int hw_id; /* id of the previous hardware message (or 0) */
|
|
@REPLY
|
|
user_handle_t win; /* window handle */
|
|
int type; /* message type */
|
|
unsigned int msg; /* message code */
|
|
unsigned long wparam; /* parameters */
|
|
unsigned long lparam; /* parameters */
|
|
unsigned long info; /* extra info */
|
|
int x; /* x position */
|
|
int y; /* y position */
|
|
unsigned int time; /* message time */
|
|
unsigned int hw_id; /* id if hardware message */
|
|
unsigned int active_hooks; /* active hooks bitmap */
|
|
data_size_t total; /* total size of extra data */
|
|
VARARG(data,message_data); /* message data for sent messages */
|
|
@END
|
|
|
|
|
|
/* Reply to a sent message */
|
|
@REQ(reply_message)
|
|
unsigned int result; /* message result */
|
|
int remove; /* should we remove the message? */
|
|
VARARG(data,bytes); /* message data for sent messages */
|
|
@END
|
|
|
|
|
|
/* Accept the current hardware message */
|
|
@REQ(accept_hardware_message)
|
|
unsigned int hw_id; /* id of the hardware message */
|
|
int remove; /* should we remove the message? */
|
|
user_handle_t new_win; /* new destination window for current message */
|
|
@END
|
|
|
|
|
|
/* Retrieve the reply for the last message sent */
|
|
@REQ(get_message_reply)
|
|
int cancel; /* cancel message if not ready? */
|
|
@REPLY
|
|
unsigned int result; /* message result */
|
|
VARARG(data,bytes); /* message data for sent messages */
|
|
@END
|
|
|
|
|
|
/* Set a window timer */
|
|
@REQ(set_win_timer)
|
|
user_handle_t win; /* window handle */
|
|
unsigned int msg; /* message to post */
|
|
unsigned int rate; /* timer rate in ms */
|
|
unsigned long id; /* timer id */
|
|
unsigned long lparam; /* message lparam (callback proc) */
|
|
@REPLY
|
|
unsigned long id; /* timer id */
|
|
@END
|
|
|
|
|
|
/* Kill a window timer */
|
|
@REQ(kill_win_timer)
|
|
user_handle_t win; /* window handle */
|
|
unsigned int msg; /* message to post */
|
|
unsigned long id; /* timer id */
|
|
@END
|
|
|
|
|
|
/* Retrieve info about a serial port */
|
|
@REQ(get_serial_info)
|
|
obj_handle_t handle; /* handle to comm port */
|
|
@REPLY
|
|
unsigned int readinterval;
|
|
unsigned int readconst;
|
|
unsigned int readmult;
|
|
unsigned int writeconst;
|
|
unsigned int writemult;
|
|
unsigned int eventmask;
|
|
@END
|
|
|
|
|
|
/* Set info about a serial port */
|
|
@REQ(set_serial_info)
|
|
obj_handle_t handle; /* handle to comm port */
|
|
int flags; /* bitmask to set values (see below) */
|
|
unsigned int readinterval;
|
|
unsigned int readconst;
|
|
unsigned int readmult;
|
|
unsigned int writeconst;
|
|
unsigned int writemult;
|
|
unsigned int eventmask;
|
|
@END
|
|
#define SERIALINFO_SET_TIMEOUTS 0x01
|
|
#define SERIALINFO_SET_MASK 0x02
|
|
|
|
|
|
/* Create an async I/O */
|
|
@REQ(register_async)
|
|
obj_handle_t handle; /* handle to comm port, socket or file */
|
|
int type; /* type of queue to look after */
|
|
int count; /* count - usually # of bytes to be read/written */
|
|
async_data_t async; /* async I/O parameters */
|
|
@END
|
|
#define ASYNC_TYPE_READ 0x01
|
|
#define ASYNC_TYPE_WRITE 0x02
|
|
#define ASYNC_TYPE_WAIT 0x03
|
|
|
|
|
|
/* Cancel all async op on a fd */
|
|
@REQ(cancel_async)
|
|
obj_handle_t handle; /* handle to comm port, socket or file */
|
|
@END
|
|
|
|
|
|
/* Perform an ioctl on a file */
|
|
@REQ(ioctl)
|
|
obj_handle_t handle; /* handle to the device */
|
|
ioctl_code_t code; /* ioctl code */
|
|
async_data_t async; /* async I/O parameters */
|
|
VARARG(in_data,bytes); /* ioctl input data */
|
|
@REPLY
|
|
obj_handle_t wait; /* handle to wait on for blocking ioctl */
|
|
unsigned int options; /* device open options */
|
|
VARARG(out_data,bytes); /* ioctl output data */
|
|
@END
|
|
|
|
|
|
/* Retrieve results of an async ioctl */
|
|
@REQ(get_ioctl_result)
|
|
obj_handle_t handle; /* handle to the device */
|
|
void* user_arg; /* user arg used to identify the request */
|
|
@REPLY
|
|
VARARG(out_data,bytes); /* ioctl output data */
|
|
@END
|
|
|
|
|
|
/* Create a named pipe */
|
|
@REQ(create_named_pipe)
|
|
unsigned int access;
|
|
unsigned int attributes; /* object attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
unsigned int options;
|
|
unsigned int flags;
|
|
unsigned int maxinstances;
|
|
unsigned int outsize;
|
|
unsigned int insize;
|
|
timeout_t timeout;
|
|
VARARG(name,unicode_str); /* pipe name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the pipe */
|
|
@END
|
|
|
|
/* flags in create_named_pipe and get_named_pipe_info */
|
|
#define NAMED_PIPE_MESSAGE_STREAM_WRITE 0x0001
|
|
#define NAMED_PIPE_MESSAGE_STREAM_READ 0x0002
|
|
#define NAMED_PIPE_NONBLOCKING_MODE 0x0004
|
|
#define NAMED_PIPE_SERVER_END 0x8000
|
|
|
|
|
|
@REQ(get_named_pipe_info)
|
|
obj_handle_t handle;
|
|
@REPLY
|
|
unsigned int flags;
|
|
unsigned int maxinstances;
|
|
unsigned int instances;
|
|
unsigned int outsize;
|
|
unsigned int insize;
|
|
@END
|
|
|
|
|
|
/* Create a window */
|
|
@REQ(create_window)
|
|
user_handle_t parent; /* parent window */
|
|
user_handle_t owner; /* owner window */
|
|
atom_t atom; /* class atom */
|
|
void* instance; /* module instance */
|
|
@REPLY
|
|
user_handle_t handle; /* created window */
|
|
user_handle_t parent; /* full handle of parent */
|
|
user_handle_t owner; /* full handle of owner */
|
|
int extra; /* number of extra bytes */
|
|
void* class_ptr; /* pointer to class in client address space */
|
|
@END
|
|
|
|
|
|
/* Destroy a window */
|
|
@REQ(destroy_window)
|
|
user_handle_t handle; /* handle to the window */
|
|
@END
|
|
|
|
|
|
/* Retrieve the desktop window for the current thread */
|
|
@REQ(get_desktop_window)
|
|
int force; /* force creation if it doesn't exist */
|
|
@REPLY
|
|
user_handle_t handle; /* handle to the desktop window */
|
|
@END
|
|
|
|
|
|
/* Set a window owner */
|
|
@REQ(set_window_owner)
|
|
user_handle_t handle; /* handle to the window */
|
|
user_handle_t owner; /* new owner */
|
|
@REPLY
|
|
user_handle_t full_owner; /* full handle of new owner */
|
|
user_handle_t prev_owner; /* full handle of previous owner */
|
|
@END
|
|
|
|
|
|
/* Get information from a window handle */
|
|
@REQ(get_window_info)
|
|
user_handle_t handle; /* handle to the window */
|
|
@REPLY
|
|
user_handle_t full_handle; /* full 32-bit handle */
|
|
user_handle_t last_active; /* last active popup */
|
|
process_id_t pid; /* process owning the window */
|
|
thread_id_t tid; /* thread owning the window */
|
|
atom_t atom; /* class atom */
|
|
int is_unicode; /* ANSI or unicode */
|
|
@END
|
|
|
|
|
|
/* Set some information in a window */
|
|
@REQ(set_window_info)
|
|
unsigned int flags; /* flags for fields to set (see below) */
|
|
user_handle_t handle; /* handle to the window */
|
|
unsigned int style; /* window style */
|
|
unsigned int ex_style; /* window extended style */
|
|
unsigned int id; /* window id */
|
|
int is_unicode; /* ANSI or unicode */
|
|
void* instance; /* creator instance */
|
|
unsigned long user_data; /* user-specific data */
|
|
int extra_offset; /* offset to set in extra bytes */
|
|
data_size_t extra_size; /* size to set in extra bytes */
|
|
unsigned long extra_value; /* value to set in extra bytes */
|
|
@REPLY
|
|
unsigned int old_style; /* old window style */
|
|
unsigned int old_ex_style; /* old window extended style */
|
|
unsigned int old_id; /* old window id */
|
|
void* old_instance; /* old creator instance */
|
|
unsigned long old_user_data; /* old user-specific data */
|
|
unsigned long old_extra_value; /* old value in extra bytes */
|
|
@END
|
|
#define SET_WIN_STYLE 0x01
|
|
#define SET_WIN_EXSTYLE 0x02
|
|
#define SET_WIN_ID 0x04
|
|
#define SET_WIN_INSTANCE 0x08
|
|
#define SET_WIN_USERDATA 0x10
|
|
#define SET_WIN_EXTRA 0x20
|
|
#define SET_WIN_UNICODE 0x40
|
|
|
|
|
|
/* Set the parent of a window */
|
|
@REQ(set_parent)
|
|
user_handle_t handle; /* handle to the window */
|
|
user_handle_t parent; /* handle to the parent */
|
|
@REPLY
|
|
user_handle_t old_parent; /* old parent window */
|
|
user_handle_t full_parent; /* full handle of new parent */
|
|
@END
|
|
|
|
|
|
/* Get a list of the window parents, up to the root of the tree */
|
|
@REQ(get_window_parents)
|
|
user_handle_t handle; /* handle to the window */
|
|
@REPLY
|
|
int count; /* total count of parents */
|
|
VARARG(parents,user_handles); /* parent handles */
|
|
@END
|
|
|
|
|
|
/* Get a list of the window children */
|
|
@REQ(get_window_children)
|
|
user_handle_t parent; /* parent window */
|
|
atom_t atom; /* class atom for the listed children */
|
|
thread_id_t tid; /* thread owning the listed children */
|
|
@REPLY
|
|
int count; /* total count of children */
|
|
VARARG(children,user_handles); /* children handles */
|
|
@END
|
|
|
|
|
|
/* Get a list of the window children that contain a given point */
|
|
@REQ(get_window_children_from_point)
|
|
user_handle_t parent; /* parent window */
|
|
int x; /* point in parent coordinates */
|
|
int y;
|
|
@REPLY
|
|
int count; /* total count of children */
|
|
VARARG(children,user_handles); /* children handles */
|
|
@END
|
|
|
|
|
|
/* Get window tree information from a window handle */
|
|
@REQ(get_window_tree)
|
|
user_handle_t handle; /* handle to the window */
|
|
@REPLY
|
|
user_handle_t parent; /* parent window */
|
|
user_handle_t owner; /* owner window */
|
|
user_handle_t next_sibling; /* next sibling in Z-order */
|
|
user_handle_t prev_sibling; /* prev sibling in Z-order */
|
|
user_handle_t first_sibling; /* first sibling in Z-order */
|
|
user_handle_t last_sibling; /* last sibling in Z-order */
|
|
user_handle_t first_child; /* first child */
|
|
user_handle_t last_child; /* last child */
|
|
@END
|
|
|
|
/* Set the position and Z order of a window */
|
|
@REQ(set_window_pos)
|
|
unsigned int flags; /* SWP_* flags */
|
|
user_handle_t handle; /* handle to the window */
|
|
user_handle_t previous; /* previous window in Z order */
|
|
rectangle_t window; /* window rectangle */
|
|
rectangle_t client; /* client rectangle */
|
|
VARARG(valid,rectangles); /* valid rectangles from WM_NCCALCSIZE */
|
|
@REPLY
|
|
unsigned int new_style; /* new window style */
|
|
@END
|
|
|
|
|
|
/* Get the window and client rectangles of a window */
|
|
@REQ(get_window_rectangles)
|
|
user_handle_t handle; /* handle to the window */
|
|
@REPLY
|
|
rectangle_t window; /* window rectangle */
|
|
rectangle_t visible; /* visible part of the window rectangle */
|
|
rectangle_t client; /* client rectangle */
|
|
@END
|
|
|
|
|
|
/* Get the window text */
|
|
@REQ(get_window_text)
|
|
user_handle_t handle; /* handle to the window */
|
|
@REPLY
|
|
VARARG(text,unicode_str); /* window text */
|
|
@END
|
|
|
|
|
|
/* Set the window text */
|
|
@REQ(set_window_text)
|
|
user_handle_t handle; /* handle to the window */
|
|
VARARG(text,unicode_str); /* window text */
|
|
@END
|
|
|
|
|
|
/* Get the coordinates offset between two windows */
|
|
@REQ(get_windows_offset)
|
|
user_handle_t from; /* handle to the first window */
|
|
user_handle_t to; /* handle to the second window */
|
|
@REPLY
|
|
int x; /* x coordinate offset */
|
|
int y; /* y coordinate offset */
|
|
@END
|
|
|
|
|
|
/* Get the visible region of a window */
|
|
@REQ(get_visible_region)
|
|
user_handle_t window; /* handle to the window */
|
|
unsigned int flags; /* DCX flags */
|
|
@REPLY
|
|
user_handle_t top_win; /* top window to clip against */
|
|
rectangle_t top_rect; /* top window visible rect with screen coords */
|
|
rectangle_t win_rect; /* window rect in screen coords */
|
|
data_size_t total_size; /* total size of the resulting region */
|
|
VARARG(region,rectangles); /* list of rectangles for the region (in screen coords) */
|
|
@END
|
|
|
|
|
|
/* Get the window region */
|
|
@REQ(get_window_region)
|
|
user_handle_t window; /* handle to the window */
|
|
@REPLY
|
|
data_size_t total_size; /* total size of the resulting region */
|
|
VARARG(region,rectangles); /* list of rectangles for the region */
|
|
@END
|
|
|
|
|
|
/* Set the window region */
|
|
@REQ(set_window_region)
|
|
user_handle_t window; /* handle to the window */
|
|
int redraw; /* redraw the window? */
|
|
VARARG(region,rectangles); /* list of rectangles for the region */
|
|
@END
|
|
|
|
|
|
/* Get the window update region */
|
|
@REQ(get_update_region)
|
|
user_handle_t window; /* handle to the window */
|
|
user_handle_t from_child; /* child to start searching from */
|
|
unsigned int flags; /* update flags (see below) */
|
|
@REPLY
|
|
user_handle_t child; /* child to repaint (or window itself) */
|
|
unsigned int flags; /* resulting update flags (see below) */
|
|
data_size_t total_size; /* total size of the resulting region */
|
|
VARARG(region,rectangles); /* list of rectangles for the region */
|
|
@END
|
|
#define UPDATE_NONCLIENT 0x01 /* get region for repainting non-client area */
|
|
#define UPDATE_ERASE 0x02 /* get region for erasing client area */
|
|
#define UPDATE_PAINT 0x04 /* get region for painting client area */
|
|
#define UPDATE_INTERNALPAINT 0x08 /* get region if internal paint is pending */
|
|
#define UPDATE_ALLCHILDREN 0x10 /* force repaint of all children */
|
|
#define UPDATE_NOCHILDREN 0x20 /* don't try to repaint any children */
|
|
#define UPDATE_NOREGION 0x40 /* don't return a region, only the flags */
|
|
|
|
|
|
/* Update the z order of a window so that a given rectangle is fully visible */
|
|
@REQ(update_window_zorder)
|
|
user_handle_t window; /* handle to the window */
|
|
rectangle_t rect; /* rectangle that must be visible */
|
|
@END
|
|
|
|
|
|
/* Mark parts of a window as needing a redraw */
|
|
@REQ(redraw_window)
|
|
user_handle_t window; /* handle to the window */
|
|
unsigned int flags; /* RDW_* flags */
|
|
VARARG(region,rectangles); /* list of rectangles for the region */
|
|
@END
|
|
|
|
|
|
/* Set a window property */
|
|
@REQ(set_window_property)
|
|
user_handle_t window; /* handle to the window */
|
|
atom_t atom; /* property atom (if no name specified) */
|
|
obj_handle_t handle; /* handle to store */
|
|
VARARG(name,unicode_str); /* property name */
|
|
@END
|
|
|
|
|
|
/* Remove a window property */
|
|
@REQ(remove_window_property)
|
|
user_handle_t window; /* handle to the window */
|
|
atom_t atom; /* property atom (if no name specified) */
|
|
VARARG(name,unicode_str); /* property name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle stored in property */
|
|
@END
|
|
|
|
|
|
/* Get a window property */
|
|
@REQ(get_window_property)
|
|
user_handle_t window; /* handle to the window */
|
|
atom_t atom; /* property atom (if no name specified) */
|
|
VARARG(name,unicode_str); /* property name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle stored in property */
|
|
@END
|
|
|
|
|
|
/* Get the list of properties of a window */
|
|
@REQ(get_window_properties)
|
|
user_handle_t window; /* handle to the window */
|
|
@REPLY
|
|
int total; /* total number of properties */
|
|
VARARG(props,properties); /* list of properties */
|
|
@END
|
|
|
|
|
|
/* Create a window station */
|
|
@REQ(create_winstation)
|
|
unsigned int flags; /* window station flags */
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the window station */
|
|
@END
|
|
|
|
|
|
/* Open a handle to a window station */
|
|
@REQ(open_winstation)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the window station */
|
|
@END
|
|
|
|
|
|
/* Close a window station */
|
|
@REQ(close_winstation)
|
|
obj_handle_t handle; /* handle to the window station */
|
|
@END
|
|
|
|
|
|
/* Get the process current window station */
|
|
@REQ(get_process_winstation)
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the window station */
|
|
@END
|
|
|
|
|
|
/* Set the process current window station */
|
|
@REQ(set_process_winstation)
|
|
obj_handle_t handle; /* handle to the window station */
|
|
@END
|
|
|
|
|
|
/* Create a desktop */
|
|
@REQ(create_desktop)
|
|
unsigned int flags; /* desktop flags */
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the desktop */
|
|
@END
|
|
|
|
|
|
/* Open a handle to a desktop */
|
|
@REQ(open_desktop)
|
|
unsigned int flags; /* desktop flags */
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the desktop */
|
|
@END
|
|
|
|
|
|
/* Close a desktop */
|
|
@REQ(close_desktop)
|
|
obj_handle_t handle; /* handle to the desktop */
|
|
@END
|
|
|
|
|
|
/* Get the thread current desktop */
|
|
@REQ(get_thread_desktop)
|
|
thread_id_t tid; /* thread id */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the desktop */
|
|
@END
|
|
|
|
|
|
/* Set the thread current desktop */
|
|
@REQ(set_thread_desktop)
|
|
obj_handle_t handle; /* handle to the desktop */
|
|
@END
|
|
|
|
|
|
/* Get/set information about a user object (window station or desktop) */
|
|
@REQ(set_user_object_info)
|
|
obj_handle_t handle; /* handle to the object */
|
|
unsigned int flags; /* information to set */
|
|
unsigned int obj_flags; /* new object flags */
|
|
@REPLY
|
|
int is_desktop; /* is object a desktop? */
|
|
unsigned int old_obj_flags; /* old object flags */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@END
|
|
#define SET_USER_OBJECT_FLAGS 1
|
|
|
|
|
|
/* Attach (or detach) thread inputs */
|
|
@REQ(attach_thread_input)
|
|
thread_id_t tid_from; /* thread to be attached */
|
|
thread_id_t tid_to; /* thread to which tid_from should be attached */
|
|
int attach; /* is it an attach? */
|
|
@END
|
|
|
|
|
|
/* Get input data for a given thread */
|
|
@REQ(get_thread_input)
|
|
thread_id_t tid; /* id of thread */
|
|
@REPLY
|
|
user_handle_t focus; /* handle to the focus window */
|
|
user_handle_t capture; /* handle to the capture window */
|
|
user_handle_t active; /* handle to the active window */
|
|
user_handle_t foreground; /* handle to the global foreground window */
|
|
user_handle_t menu_owner; /* handle to the menu owner */
|
|
user_handle_t move_size; /* handle to the moving/resizing window */
|
|
user_handle_t caret; /* handle to the caret window */
|
|
rectangle_t rect; /* caret rectangle */
|
|
@END
|
|
|
|
|
|
/* Get the time of the last input event */
|
|
@REQ(get_last_input_time)
|
|
@REPLY
|
|
unsigned int time;
|
|
@END
|
|
|
|
|
|
/* Retrieve queue keyboard state for a given thread */
|
|
@REQ(get_key_state)
|
|
thread_id_t tid; /* id of thread */
|
|
int key; /* optional key code or -1 */
|
|
@REPLY
|
|
unsigned char state; /* state of specified key */
|
|
VARARG(keystate,bytes); /* state array for all the keys */
|
|
@END
|
|
|
|
/* Set queue keyboard state for a given thread */
|
|
@REQ(set_key_state)
|
|
thread_id_t tid; /* id of thread */
|
|
VARARG(keystate,bytes); /* state array for all the keys */
|
|
@END
|
|
|
|
/* Set the system foreground window */
|
|
@REQ(set_foreground_window)
|
|
user_handle_t handle; /* handle to the foreground window */
|
|
@REPLY
|
|
user_handle_t previous; /* handle to the previous foreground window */
|
|
int send_msg_old; /* whether we have to send a msg to the old window */
|
|
int send_msg_new; /* whether we have to send a msg to the new window */
|
|
@END
|
|
|
|
/* Set the current thread focus window */
|
|
@REQ(set_focus_window)
|
|
user_handle_t handle; /* handle to the focus window */
|
|
@REPLY
|
|
user_handle_t previous; /* handle to the previous focus window */
|
|
@END
|
|
|
|
/* Set the current thread active window */
|
|
@REQ(set_active_window)
|
|
user_handle_t handle; /* handle to the active window */
|
|
@REPLY
|
|
user_handle_t previous; /* handle to the previous active window */
|
|
@END
|
|
|
|
/* Set the current thread capture window */
|
|
@REQ(set_capture_window)
|
|
user_handle_t handle; /* handle to the capture window */
|
|
unsigned int flags; /* capture flags (see below) */
|
|
@REPLY
|
|
user_handle_t previous; /* handle to the previous capture window */
|
|
user_handle_t full_handle; /* full 32-bit handle of new capture window */
|
|
@END
|
|
#define CAPTURE_MENU 0x01 /* capture is for a menu */
|
|
#define CAPTURE_MOVESIZE 0x02 /* capture is for moving/resizing */
|
|
|
|
|
|
/* Set the current thread caret window */
|
|
@REQ(set_caret_window)
|
|
user_handle_t handle; /* handle to the caret window */
|
|
int width; /* caret width */
|
|
int height; /* caret height */
|
|
@REPLY
|
|
user_handle_t previous; /* handle to the previous caret window */
|
|
rectangle_t old_rect; /* previous caret rectangle */
|
|
int old_hide; /* previous hide count */
|
|
int old_state; /* previous caret state (1=on, 0=off) */
|
|
@END
|
|
|
|
|
|
/* Set the current thread caret information */
|
|
@REQ(set_caret_info)
|
|
unsigned int flags; /* caret flags (see below) */
|
|
user_handle_t handle; /* handle to the caret window */
|
|
int x; /* caret x position */
|
|
int y; /* caret y position */
|
|
int hide; /* increment for hide count (can be negative to show it) */
|
|
int state; /* caret state (1=on, 0=off, -1=toggle current state) */
|
|
@REPLY
|
|
user_handle_t full_handle; /* handle to the current caret window */
|
|
rectangle_t old_rect; /* previous caret rectangle */
|
|
int old_hide; /* previous hide count */
|
|
int old_state; /* previous caret state (1=on, 0=off) */
|
|
@END
|
|
#define SET_CARET_POS 0x01 /* set the caret position from x,y */
|
|
#define SET_CARET_HIDE 0x02 /* increment the caret hide count */
|
|
#define SET_CARET_STATE 0x04 /* set the caret on/off state */
|
|
|
|
|
|
/* Set a window hook */
|
|
@REQ(set_hook)
|
|
int id; /* id of the hook */
|
|
process_id_t pid; /* id of process to set the hook into */
|
|
thread_id_t tid; /* id of thread to set the hook into */
|
|
int event_min;
|
|
int event_max;
|
|
int flags;
|
|
void* proc; /* hook procedure */
|
|
int unicode; /* is it a unicode hook? */
|
|
VARARG(module,unicode_str); /* module name */
|
|
@REPLY
|
|
user_handle_t handle; /* handle to the hook */
|
|
unsigned int active_hooks; /* active hooks bitmap */
|
|
@END
|
|
|
|
|
|
/* Remove a window hook */
|
|
@REQ(remove_hook)
|
|
user_handle_t handle; /* handle to the hook */
|
|
int id; /* id of the hook if handle is 0 */
|
|
void* proc; /* hook procedure if handle is 0 */
|
|
@REPLY
|
|
unsigned int active_hooks; /* active hooks bitmap */
|
|
@END
|
|
|
|
|
|
/* Start calling a hook chain */
|
|
@REQ(start_hook_chain)
|
|
int id; /* id of the hook */
|
|
int event; /* signalled event */
|
|
user_handle_t window; /* handle to the event window */
|
|
int object_id; /* object id for out of context winevent */
|
|
int child_id; /* child id for out of context winevent */
|
|
@REPLY
|
|
user_handle_t handle; /* handle to the next hook */
|
|
process_id_t pid; /* process id for low-level keyboard/mouse hooks */
|
|
thread_id_t tid; /* thread id for low-level keyboard/mouse hooks */
|
|
void* proc; /* hook procedure */
|
|
int unicode; /* is it a unicode hook? */
|
|
unsigned int active_hooks; /* active hooks bitmap */
|
|
VARARG(module,unicode_str); /* module name */
|
|
@END
|
|
|
|
|
|
/* Finished calling a hook chain */
|
|
@REQ(finish_hook_chain)
|
|
int id; /* id of the hook */
|
|
@END
|
|
|
|
|
|
/* Get the hook information */
|
|
@REQ(get_hook_info)
|
|
user_handle_t handle; /* handle to the current hook */
|
|
int get_next; /* do we want info about current or next hook? */
|
|
int event; /* signalled event */
|
|
user_handle_t window; /* handle to the event window */
|
|
int object_id; /* object id for out of context winevent */
|
|
int child_id; /* child id for out of context winevent */
|
|
@REPLY
|
|
user_handle_t handle; /* handle to the hook */
|
|
int id; /* id of the hook */
|
|
process_id_t pid; /* process id for low-level keyboard/mouse hooks */
|
|
thread_id_t tid; /* thread id for low-level keyboard/mouse hooks */
|
|
void* proc; /* hook procedure */
|
|
int unicode; /* is it a unicode hook? */
|
|
VARARG(module,unicode_str); /* module name */
|
|
@END
|
|
|
|
|
|
/* Create a window class */
|
|
@REQ(create_class)
|
|
int local; /* is it a local class? */
|
|
atom_t atom; /* class atom */
|
|
unsigned int style; /* class style */
|
|
void* instance; /* module instance */
|
|
int extra; /* number of extra class bytes */
|
|
int win_extra; /* number of window extra bytes */
|
|
void* client_ptr; /* pointer to class in client address space */
|
|
@END
|
|
|
|
|
|
/* Destroy a window class */
|
|
@REQ(destroy_class)
|
|
atom_t atom; /* class atom */
|
|
void* instance; /* module instance */
|
|
@REPLY
|
|
void* client_ptr; /* pointer to class in client address space */
|
|
@END
|
|
|
|
|
|
/* Set some information in a class */
|
|
@REQ(set_class_info)
|
|
user_handle_t window; /* handle to the window */
|
|
unsigned int flags; /* flags for info to set (see below) */
|
|
atom_t atom; /* class atom */
|
|
unsigned int style; /* class style */
|
|
int win_extra; /* number of window extra bytes */
|
|
void* instance; /* module instance */
|
|
int extra_offset; /* offset to set in extra bytes */
|
|
data_size_t extra_size; /* size to set in extra bytes */
|
|
unsigned long extra_value; /* value to set in extra bytes */
|
|
@REPLY
|
|
atom_t old_atom; /* previous class atom */
|
|
unsigned int old_style; /* previous class style */
|
|
int old_extra; /* previous number of class extra bytes */
|
|
int old_win_extra; /* previous number of window extra bytes */
|
|
void* old_instance; /* previous module instance */
|
|
unsigned long old_extra_value; /* old value in extra bytes */
|
|
@END
|
|
#define SET_CLASS_ATOM 0x0001
|
|
#define SET_CLASS_STYLE 0x0002
|
|
#define SET_CLASS_WINEXTRA 0x0004
|
|
#define SET_CLASS_INSTANCE 0x0008
|
|
#define SET_CLASS_EXTRA 0x0010
|
|
|
|
|
|
/* Set/get clipboard information */
|
|
@REQ(set_clipboard_info)
|
|
unsigned int flags; /* flags for fields to set (see below) */
|
|
user_handle_t clipboard; /* clipboard window */
|
|
user_handle_t owner; /* clipboard owner */
|
|
user_handle_t viewer; /* first clipboard viewer */
|
|
unsigned int seqno; /* change sequence number */
|
|
@REPLY
|
|
unsigned int flags; /* status flags (see below) */
|
|
user_handle_t old_clipboard; /* old clipboard window */
|
|
user_handle_t old_owner; /* old clipboard owner */
|
|
user_handle_t old_viewer; /* old clipboard viewer */
|
|
unsigned int seqno; /* current sequence number */
|
|
@END
|
|
|
|
#define SET_CB_OPEN 0x001
|
|
#define SET_CB_OWNER 0x002
|
|
#define SET_CB_VIEWER 0x004
|
|
#define SET_CB_SEQNO 0x008
|
|
#define SET_CB_RELOWNER 0x010
|
|
#define SET_CB_CLOSE 0x020
|
|
#define CB_OPEN 0x040
|
|
#define CB_OWNER 0x080
|
|
#define CB_PROCESS 0x100
|
|
|
|
|
|
/* Open a security token */
|
|
@REQ(open_token)
|
|
obj_handle_t handle; /* handle to the thread or process */
|
|
unsigned int access; /* access rights to the new token */
|
|
unsigned int attributes;/* object attributes */
|
|
unsigned int flags; /* flags (see below) */
|
|
@REPLY
|
|
obj_handle_t token; /* handle to the token */
|
|
@END
|
|
#define OPEN_TOKEN_THREAD 1
|
|
#define OPEN_TOKEN_AS_SELF 2
|
|
|
|
|
|
/* Set/get the global windows */
|
|
@REQ(set_global_windows)
|
|
unsigned int flags; /* flags for fields to set (see below) */
|
|
user_handle_t shell_window; /* handle to the new shell window */
|
|
user_handle_t shell_listview; /* handle to the new shell listview window */
|
|
user_handle_t progman_window; /* handle to the new program manager window */
|
|
user_handle_t taskman_window; /* handle to the new task manager window */
|
|
@REPLY
|
|
user_handle_t old_shell_window; /* handle to the shell window */
|
|
user_handle_t old_shell_listview; /* handle to the shell listview window */
|
|
user_handle_t old_progman_window; /* handle to the new program manager window */
|
|
user_handle_t old_taskman_window; /* handle to the new task manager window */
|
|
@END
|
|
#define SET_GLOBAL_SHELL_WINDOWS 0x01 /* set both main shell and listview windows */
|
|
#define SET_GLOBAL_PROGMAN_WINDOW 0x02
|
|
#define SET_GLOBAL_TASKMAN_WINDOW 0x04
|
|
|
|
/* Adjust the privileges held by a token */
|
|
@REQ(adjust_token_privileges)
|
|
obj_handle_t handle; /* handle to the token */
|
|
int disable_all; /* disable all privileges? */
|
|
int get_modified_state; /* get modified privileges? */
|
|
VARARG(privileges,LUID_AND_ATTRIBUTES); /* privileges to enable/disable/remove */
|
|
@REPLY
|
|
unsigned int len; /* total length in bytes required to store token privileges */
|
|
VARARG(privileges,LUID_AND_ATTRIBUTES); /* modified privileges */
|
|
@END
|
|
|
|
/* Retrieves the set of privileges held by or available to a token */
|
|
@REQ(get_token_privileges)
|
|
obj_handle_t handle; /* handle to the token */
|
|
@REPLY
|
|
unsigned int len; /* total length in bytes required to store token privileges */
|
|
VARARG(privileges,LUID_AND_ATTRIBUTES); /* privileges held by or available to a token */
|
|
@END
|
|
|
|
/* Check the token has the required privileges */
|
|
@REQ(check_token_privileges)
|
|
obj_handle_t handle; /* handle to the token */
|
|
int all_required; /* are all the privileges required for the check to succeed? */
|
|
VARARG(privileges,LUID_AND_ATTRIBUTES); /* privileges to check */
|
|
@REPLY
|
|
int has_privileges; /* does the token have the required privileges? */
|
|
VARARG(privileges,LUID_AND_ATTRIBUTES); /* privileges held by or available to a token */
|
|
@END
|
|
|
|
@REQ(duplicate_token)
|
|
obj_handle_t handle; /* handle to the token to duplicate */
|
|
unsigned int access; /* access rights to the new token */
|
|
unsigned int attributes; /* object attributes */
|
|
int primary; /* is the new token to be a primary one? */
|
|
int impersonation_level; /* impersonation level of the new token */
|
|
@REPLY
|
|
obj_handle_t new_handle; /* duplicated handle */
|
|
@END
|
|
|
|
@REQ(access_check)
|
|
obj_handle_t handle; /* handle to the token */
|
|
unsigned int desired_access; /* desired access to the object */
|
|
unsigned int mapping_read; /* mapping from generic read to specific rights */
|
|
unsigned int mapping_write; /* mapping from generic write to specific rights */
|
|
unsigned int mapping_execute; /* mapping from generic execute to specific rights */
|
|
unsigned int mapping_all; /* mapping from generic all to specific rights */
|
|
VARARG(sd,security_descriptor); /* security descriptor to check */
|
|
@REPLY
|
|
unsigned int access_granted; /* access rights actually granted */
|
|
unsigned int access_status; /* was access granted? */
|
|
unsigned int privileges_len; /* length needed to store privileges */
|
|
VARARG(privileges,LUID_AND_ATTRIBUTES); /* privileges used during access check */
|
|
@END
|
|
|
|
@REQ(get_token_user)
|
|
obj_handle_t handle; /* handle to the token */
|
|
@REPLY
|
|
data_size_t user_len; /* length needed to store user */
|
|
VARARG(user,SID); /* sid of the user the token represents */
|
|
@END
|
|
|
|
@REQ(get_token_groups)
|
|
obj_handle_t handle; /* handle to the token */
|
|
@REPLY
|
|
data_size_t user_len; /* length needed to store user */
|
|
VARARG(user,token_groups); /* groups the token's user belongs to */
|
|
@END
|
|
|
|
@REQ(set_security_object)
|
|
obj_handle_t handle; /* handle to the object */
|
|
unsigned int security_info; /* which parts of security descriptor to set */
|
|
VARARG(sd,security_descriptor); /* security descriptor to set */
|
|
@END
|
|
|
|
/* Create a mailslot */
|
|
@REQ(create_mailslot)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
unsigned int max_msgsize;
|
|
timeout_t read_timeout;
|
|
VARARG(name,unicode_str); /* mailslot name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the mailslot */
|
|
@END
|
|
|
|
|
|
/* Set mailslot information */
|
|
@REQ(set_mailslot_info)
|
|
obj_handle_t handle; /* handle to the mailslot */
|
|
unsigned int flags;
|
|
timeout_t read_timeout;
|
|
@REPLY
|
|
unsigned int max_msgsize;
|
|
timeout_t read_timeout;
|
|
@END
|
|
#define MAILSLOT_SET_READ_TIMEOUT 1
|
|
|
|
|
|
/* Create a directory object */
|
|
@REQ(create_directory)
|
|
unsigned int access; /* access flags */
|
|
unsigned int attributes; /* object attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
VARARG(directory_name,unicode_str); /* Directory name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the directory */
|
|
@END
|
|
|
|
|
|
/* Open a directory object */
|
|
@REQ(open_directory)
|
|
unsigned int access; /* access flags */
|
|
unsigned int attributes; /* object attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
VARARG(directory_name,unicode_str); /* Directory name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the directory */
|
|
@END
|
|
|
|
|
|
/* Create a symbolic link object */
|
|
@REQ(create_symlink)
|
|
unsigned int access; /* access flags */
|
|
unsigned int attributes; /* object attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
data_size_t name_len; /* length of the symlink name in bytes */
|
|
VARARG(name,unicode_str,name_len); /* symlink name */
|
|
VARARG(target_name,unicode_str); /* target name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the symlink */
|
|
@END
|
|
|
|
|
|
/* Open a symbolic link object */
|
|
@REQ(open_symlink)
|
|
unsigned int access; /* access flags */
|
|
unsigned int attributes; /* object attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
VARARG(name,unicode_str); /* symlink name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the symlink */
|
|
@END
|
|
|
|
|
|
/* Query a symbolic link object */
|
|
@REQ(query_symlink)
|
|
obj_handle_t handle; /* handle to the symlink */
|
|
@REPLY
|
|
VARARG(target_name,unicode_str); /* target name */
|
|
@END
|
|
|
|
|
|
/* Query basic object information */
|
|
@REQ(get_object_info)
|
|
obj_handle_t handle; /* handle to the object */
|
|
@REPLY
|
|
unsigned int access; /* granted access mask */
|
|
unsigned int ref_count; /* object ref count */
|
|
@END
|
|
|
|
/* Query the impersonation level of an impersonation token */
|
|
@REQ(get_token_impersonation_level)
|
|
obj_handle_t handle; /* handle to the object */
|
|
@REPLY
|
|
int impersonation_level; /* impersonation level of the impersonation token */
|
|
@END
|
|
|
|
/* Allocate a locally-unique identifier */
|
|
@REQ(allocate_locally_unique_id)
|
|
@REPLY
|
|
luid_t luid;
|
|
@END
|
|
|
|
|
|
/* Create a device manager */
|
|
@REQ(create_device_manager)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the device */
|
|
@END
|
|
|
|
|
|
/* Create a device */
|
|
@REQ(create_device)
|
|
unsigned int access; /* wanted access rights */
|
|
unsigned int attributes; /* object attributes */
|
|
obj_handle_t rootdir; /* root directory */
|
|
obj_handle_t manager; /* device manager */
|
|
void* user_ptr; /* opaque ptr for use by client */
|
|
VARARG(name,unicode_str); /* object name */
|
|
@REPLY
|
|
obj_handle_t handle; /* handle to the device */
|
|
@END
|
|
|
|
|
|
/* Delete a device */
|
|
@REQ(delete_device)
|
|
obj_handle_t handle; /* handle to the device */
|
|
@END
|
|
|
|
|
|
/* Retrieve the next pending device ioctl request */
|
|
@REQ(get_next_device_request)
|
|
obj_handle_t manager; /* handle to the device manager */
|
|
obj_handle_t prev; /* handle to the previous ioctl */
|
|
unsigned int status; /* status of the previous ioctl */
|
|
VARARG(prev_data,bytes); /* output data of the previous ioctl */
|
|
@REPLY
|
|
obj_handle_t next; /* handle to the next ioctl */
|
|
ioctl_code_t code; /* ioctl code */
|
|
void* user_ptr; /* opaque ptr for the device */
|
|
data_size_t in_size; /* total needed input size */
|
|
data_size_t out_size; /* needed output size */
|
|
VARARG(next_data,bytes); /* input data of the next ioctl */
|
|
@END
|
|
|
|
|
|
/* Make the current process a system process */
|
|
@REQ(make_process_system)
|
|
@REPLY
|
|
obj_handle_t event; /* event signaled when all user processes have exited */
|
|
@END
|