mirror of
git://source.winehq.org/git/wine.git
synced 2024-11-02 17:43:44 +00:00
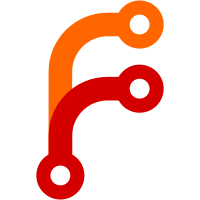
Wed Dec 7 14:52:25 1994 Alexandre Julliard (julliard@lamisun.epfl.ch) * [controls/listbox.c] Fixed problems due to new scroll-bar code. * [loader/signal.c] [miscemu/ioports.c] Handle I/O opcodes that use an absolute address. * [objects/text.c] Implemented TabbedTextOut(). Sat Dec 3 18:53:08 1994 Kenneth MacDonald <K.MacDonald@ed.ac.uk> * [objects/metafile.c] Implemented GetMetafile(). Fixed bug in PlayMetaFile() when reading disc based metafile records. Added META_POLYPOLYGON, META_DELETEOBJECT and META_EOF to PlayMetaFileRecord(). Wed Nov 30 06:32:25 1994 Martin von Loewis (martin@cs.csufresno.edu) * [Imakefile] wine.sym: Remove gcc2_compiled and friends * [controls/listbox.c][if1632/relay.c][if1632/relay.c] [loader/resource.c][memory/heap.c][objects/dib.c][windows/dialog.c] Replace #ifdef DEBUG_XXX with if(debugging_xxx){ * [if1632/call.S] CallToLibMain: New function * [if1632/relay.c][include/options.h][misc/main.c] [miscemu/int1a.c][miscemu/int21.c][miscemu/kernel.c] removed Options.relay_debug * [include/heap.h] HEAP_OWNER: Use ds instead of cs:ip * [loader/ne_image.c] LoadNEImage: Remember current exe, handle nodata dlls InitNEDLL: handle nodata dlls, call CallToLibMain * [loader/selector.c] CreateSelectors: Initialize auto_data_sel with 0 * [memory/heap.c] HEAP_CheckHeap: Check prev HEAP_CheckLocalHeaps: new function * [misc/profile] Remember and dump only changed profiles * [tools/makedebug] Introduce debugging_xxx flags Sun Nov 27 23:13:22 MET 1994 <erik@xs4all.nl> * [clipboard.h color.h dc.h dos_fs.h event.h font.h graphics.h if1632.h kernel.h library.h miscemu.h ne_image.h nonclient.h pe_image.h selectors.h wintypes.h] Added. * [*/*] - Commented all 'static char copyright statements', see misc/main.c - moved prototypes to headers files, fixed wrong prototypes. - *please* add a header file for each .c if you need to export things. * [misc/main.c] Added one static string which list the names of the contributors. Fri Nov 25 16:24:27 MET 1994 Dag Asheim (dash@ifi.uio.no) * [Configure] Made the support for multiple languages more automatic. Added a [fonts] section to the wine.conf file. Made the defaults better. Generally cleaned it up. * [rc/sysres_No.rc] [rc/sysres_De.rc] [rc/sysres.c] Norwegian resources and small fixes to the german resources. Wed Nov 23 20:28:59 1994 Martin von Loewis (martin@cs.csufresno.edu) * [debugger/break.c] bark(), toggle_next(), should_continue(): New functions insert_break(): Fixed, adds write access to page before writing wine_bp.next_addr: new structure field * [debugger/dbg.y] Changed symbol's value to be it's value instead of the value pointed to by the symbol. Changed SIGTRAP handling to allow continuation after break point * [misc/shell.c] ShellAbout(): Load resource from memory
313 lines
9 KiB
C
313 lines
9 KiB
C
/*
|
|
* GDI definitions
|
|
*
|
|
* Copyright 1993 Alexandre Julliard
|
|
*/
|
|
|
|
#ifndef GDI_H
|
|
#define GDI_H
|
|
|
|
#include <X11/Xlib.h>
|
|
#include <X11/Xutil.h>
|
|
|
|
#include "windows.h"
|
|
#include "segmem.h"
|
|
#include "heap.h"
|
|
|
|
/* GDI objects magic numbers */
|
|
#define PEN_MAGIC 0x4f47
|
|
#define BRUSH_MAGIC 0x4f48
|
|
#define FONT_MAGIC 0x4f49
|
|
#define PALETTE_MAGIC 0x4f4a
|
|
#define BITMAP_MAGIC 0x4f4b
|
|
#define REGION_MAGIC 0x4f4c
|
|
#define DC_MAGIC 0x4f4d
|
|
#define DISABLED_DC_MAGIC 0x4f4e
|
|
#define META_DC_MAGIC 0x4f4f
|
|
#define METAFILE_MAGIC 0x4f50
|
|
#define METAFILE_DC_MAGIC 0x4f51
|
|
|
|
#pragma pack(1)
|
|
|
|
typedef struct tagREGION
|
|
{
|
|
WORD type;
|
|
RECT box;
|
|
Pixmap pixmap;
|
|
Region xrgn;
|
|
} REGION;
|
|
|
|
typedef struct tagGDIOBJHDR
|
|
{
|
|
HANDLE hNext;
|
|
WORD wMagic;
|
|
DWORD dwCount;
|
|
WORD wMetaList;
|
|
} GDIOBJHDR;
|
|
|
|
typedef struct tagBRUSHOBJ
|
|
{
|
|
GDIOBJHDR header;
|
|
LOGBRUSH logbrush WINE_PACKED;
|
|
} BRUSHOBJ;
|
|
|
|
typedef struct tagPENOBJ
|
|
{
|
|
GDIOBJHDR header;
|
|
LOGPEN logpen WINE_PACKED;
|
|
} PENOBJ;
|
|
|
|
typedef struct tagPALETTEOBJ
|
|
{
|
|
GDIOBJHDR header;
|
|
LOGPALETTE logpalette WINE_PACKED;
|
|
} PALETTEOBJ;
|
|
|
|
typedef struct tagFONTOBJ
|
|
{
|
|
GDIOBJHDR header;
|
|
LOGFONT logfont WINE_PACKED;
|
|
} FONTOBJ;
|
|
|
|
typedef struct tagBITMAPOBJ
|
|
{
|
|
GDIOBJHDR header;
|
|
BITMAP bitmap;
|
|
Pixmap pixmap;
|
|
SIZE size; /* For SetBitmapDimension() */
|
|
} BITMAPOBJ;
|
|
|
|
typedef struct tagRGNOBJ
|
|
{
|
|
GDIOBJHDR header;
|
|
REGION region;
|
|
} RGNOBJ;
|
|
|
|
#pragma pack(4)
|
|
|
|
typedef struct
|
|
{
|
|
WORD version; /* 0: driver version */
|
|
WORD technology; /* 2: device technology */
|
|
WORD horzSize; /* 4: width of display in mm */
|
|
WORD vertSize; /* 6: height of display in mm */
|
|
WORD horzRes; /* 8: width of display in pixels */
|
|
WORD vertRes; /* 10: width of display in pixels */
|
|
WORD bitsPixel; /* 12: bits per pixel */
|
|
WORD planes; /* 14: color planes */
|
|
WORD numBrushes; /* 16: device-specific brushes */
|
|
WORD numPens; /* 18: device-specific pens */
|
|
WORD numMarkers; /* 20: device-specific markers */
|
|
WORD numFonts; /* 22: device-specific fonts */
|
|
WORD numColors; /* 24: size of color table */
|
|
WORD pdeviceSize; /* 26: size of PDEVICE structure */
|
|
WORD curveCaps; /* 28: curve capabilities */
|
|
WORD lineCaps; /* 30: line capabilities */
|
|
WORD polygonalCaps; /* 32: polygon capabilities */
|
|
WORD textCaps; /* 34: text capabilities */
|
|
WORD clipCaps; /* 36: clipping capabilities */
|
|
WORD rasterCaps; /* 38: raster capabilities */
|
|
WORD aspectX; /* 40: relative width of device pixel */
|
|
WORD aspectY; /* 42: relative height of device pixel */
|
|
WORD aspectXY; /* 44: relative diagonal width of device pixel */
|
|
WORD pad1[21]; /* 46-86: reserved */
|
|
WORD logPixelsX; /* 88: pixels / logical X inch */
|
|
WORD logPixelsY; /* 90: pixels / logical Y inch */
|
|
WORD pad2[6]; /* 92-102: reserved */
|
|
WORD sizePalette; /* 104: entries in system palette */
|
|
WORD numReserved; /* 106: reserved entries */
|
|
WORD colorRes; /* 108: color resolution */
|
|
} DeviceCaps;
|
|
|
|
|
|
/* Device independent DC information */
|
|
typedef struct
|
|
{
|
|
int flags;
|
|
DeviceCaps *devCaps;
|
|
|
|
HANDLE hMetaFile;
|
|
HRGN hClipRgn; /* Clip region (may be 0) */
|
|
HRGN hVisRgn; /* Visible region (must never be 0) */
|
|
HRGN hGCClipRgn; /* GC clip region (ClipRgn AND VisRgn) */
|
|
HPEN hPen;
|
|
HBRUSH hBrush;
|
|
HFONT hFont;
|
|
HBITMAP hBitmap;
|
|
HANDLE hDevice;
|
|
HPALETTE hPalette;
|
|
|
|
WORD ROPmode;
|
|
WORD polyFillMode;
|
|
WORD stretchBltMode;
|
|
WORD relAbsMode;
|
|
WORD backgroundMode;
|
|
COLORREF backgroundColor;
|
|
COLORREF textColor;
|
|
int backgroundPixel;
|
|
int textPixel;
|
|
short brushOrgX;
|
|
short brushOrgY;
|
|
|
|
WORD textAlign; /* Text alignment from SetTextAlign() */
|
|
short charExtra; /* Spacing from SetTextCharacterExtra() */
|
|
short breakTotalExtra; /* Total extra space for justification */
|
|
short breakCount; /* Break char. count */
|
|
short breakExtra; /* breakTotalExtra / breakCount */
|
|
short breakRem; /* breakTotalExtra % breakCount */
|
|
|
|
BYTE bitsPerPixel;
|
|
|
|
WORD MapMode;
|
|
short DCOrgX; /* DC origin */
|
|
short DCOrgY;
|
|
short CursPosX; /* Current position */
|
|
short CursPosY;
|
|
short WndOrgX;
|
|
short WndOrgY;
|
|
short WndExtX;
|
|
short WndExtY;
|
|
short VportOrgX;
|
|
short VportOrgY;
|
|
short VportExtX;
|
|
short VportExtY;
|
|
} WIN_DC_INFO;
|
|
|
|
|
|
/* X physical pen */
|
|
typedef struct
|
|
{
|
|
int style;
|
|
int pixel;
|
|
int width;
|
|
char * dashes;
|
|
int dash_len;
|
|
} X_PHYSPEN;
|
|
|
|
/* X physical brush */
|
|
typedef struct
|
|
{
|
|
int style;
|
|
int fillStyle;
|
|
int pixel;
|
|
Pixmap pixmap;
|
|
} X_PHYSBRUSH;
|
|
|
|
/* X physical font */
|
|
typedef struct
|
|
{
|
|
XFontStruct * fstruct;
|
|
TEXTMETRIC metrics;
|
|
} X_PHYSFONT;
|
|
|
|
/* X physical palette information */
|
|
typedef struct
|
|
{
|
|
HANDLE hMapping;
|
|
WORD mappingSize;
|
|
} X_PHYSPALETTE;
|
|
|
|
/* X-specific DC information */
|
|
typedef struct
|
|
{
|
|
GC gc; /* X Window GC */
|
|
Drawable drawable;
|
|
X_PHYSFONT font;
|
|
X_PHYSPEN pen;
|
|
X_PHYSBRUSH brush;
|
|
X_PHYSPALETTE pal;
|
|
} X_DC_INFO;
|
|
|
|
|
|
typedef struct tagDC
|
|
{
|
|
GDIOBJHDR header;
|
|
WORD saveLevel;
|
|
WIN_DC_INFO w;
|
|
union
|
|
{
|
|
X_DC_INFO x;
|
|
/* other devices (e.g. printer) */
|
|
} u;
|
|
} DC;
|
|
|
|
/* DC flags */
|
|
#define DC_MEMORY 1 /* It is a memory DC */
|
|
#define DC_SAVED 2 /* It is a saved DC */
|
|
|
|
/* Last 32 bytes are reserved for stock object handles */
|
|
#define GDI_HEAP_SIZE 0xffe0
|
|
|
|
/* First handle possible for stock objects (must be >= GDI_HEAP_SIZE) */
|
|
#define FIRST_STOCK_HANDLE GDI_HEAP_SIZE
|
|
|
|
/* Stock objects handles */
|
|
|
|
#define STOCK_WHITE_BRUSH (FIRST_STOCK_HANDLE + WHITE_BRUSH)
|
|
#define STOCK_LTGRAY_BRUSH (FIRST_STOCK_HANDLE + LTGRAY_BRUSH)
|
|
#define STOCK_GRAY_BRUSH (FIRST_STOCK_HANDLE + GRAY_BRUSH)
|
|
#define STOCK_DKGRAY_BRUSH (FIRST_STOCK_HANDLE + DKGRAY_BRUSH)
|
|
#define STOCK_BLACK_BRUSH (FIRST_STOCK_HANDLE + BLACK_BRUSH)
|
|
#define STOCK_NULL_BRUSH (FIRST_STOCK_HANDLE + NULL_BRUSH)
|
|
#define STOCK_HOLLOW_BRUSH (FIRST_STOCK_HANDLE + HOLLOW_BRUSH)
|
|
#define STOCK_WHITE_PEN (FIRST_STOCK_HANDLE + WHITE_PEN)
|
|
#define STOCK_BLACK_PEN (FIRST_STOCK_HANDLE + BLACK_PEN)
|
|
#define STOCK_NULL_PEN (FIRST_STOCK_HANDLE + NULL_PEN)
|
|
#define STOCK_OEM_FIXED_FONT (FIRST_STOCK_HANDLE + OEM_FIXED_FONT)
|
|
#define STOCK_ANSI_FIXED_FONT (FIRST_STOCK_HANDLE + ANSI_FIXED_FONT)
|
|
#define STOCK_ANSI_VAR_FONT (FIRST_STOCK_HANDLE + ANSI_VAR_FONT)
|
|
#define STOCK_SYSTEM_FONT (FIRST_STOCK_HANDLE + SYSTEM_FONT)
|
|
#define STOCK_DEVICE_DEFAULT_FONT (FIRST_STOCK_HANDLE + DEVICE_DEFAULT_FONT)
|
|
#define STOCK_DEFAULT_PALETTE (FIRST_STOCK_HANDLE + DEFAULT_PALETTE)
|
|
#define STOCK_SYSTEM_FIXED_FONT (FIRST_STOCK_HANDLE + SYSTEM_FIXED_FONT)
|
|
|
|
#define NB_STOCK_OBJECTS (SYSTEM_FIXED_FONT + 1)
|
|
|
|
#define FIRST_STOCK_FONT STOCK_OEM_FIXED_FONT
|
|
#define LAST_STOCK_FONT STOCK_SYSTEM_FIXED_FONT
|
|
|
|
|
|
|
|
/* Device <-> logical coords conversion */
|
|
|
|
#define XDPTOLP(dc,x) \
|
|
(((x)-(dc)->w.VportOrgX) * (dc)->w.WndExtX / (dc)->w.VportExtX+(dc)->w.WndOrgX)
|
|
#define YDPTOLP(dc,y) \
|
|
(((y)-(dc)->w.VportOrgY) * (dc)->w.WndExtY / (dc)->w.VportExtY+(dc)->w.WndOrgY)
|
|
#define XLPTODP(dc,x) \
|
|
(((x)-(dc)->w.WndOrgX) * (dc)->w.VportExtX / (dc)->w.WndExtX+(dc)->w.VportOrgX)
|
|
#define YLPTODP(dc,y) \
|
|
(((y)-(dc)->w.WndOrgY) * (dc)->w.VportExtY / (dc)->w.WndExtY+(dc)->w.VportOrgY)
|
|
|
|
|
|
/* GDI local heap */
|
|
|
|
#ifdef WINELIB
|
|
|
|
#define GDI_HEAP_ALLOC(f,size) LocalAlloc (f,size)
|
|
#define GDI_HEAP_ADDR(handle) LocalLock (handle)
|
|
#define GDI_HEAP_FREE(handle) LocalFree (handle)
|
|
|
|
#else
|
|
|
|
extern MDESC *GDI_Heap;
|
|
|
|
#define GDI_HEAP_ALLOC(f,size) ((int)HEAP_Alloc(&GDI_Heap,f,size) & 0xffff)
|
|
#define GDI_HEAP_FREE(handle) (HEAP_Free(&GDI_Heap,GDI_HEAP_ADDR(handle)))
|
|
#define GDI_HEAP_ADDR(handle) \
|
|
((void *)((handle) ? ((handle) | ((int)GDI_Heap & 0xffff0000)) : 0))
|
|
|
|
#endif
|
|
|
|
extern BOOL GDI_Init(void);
|
|
extern HANDLE GDI_AllocObject( WORD, WORD );
|
|
extern BOOL GDI_FreeObject( HANDLE );
|
|
extern GDIOBJHDR * GDI_GetObjPtr( HANDLE, WORD );
|
|
|
|
extern Display * display;
|
|
extern Screen * screen;
|
|
extern Window rootWindow;
|
|
extern int screenWidth, screenHeight, screenDepth;
|
|
extern int desktopX, desktopY; /* misc/main.c */
|
|
|
|
#endif /* GDI_H */
|