mirror of
git://source.winehq.org/git/wine.git
synced 2024-09-15 02:44:46 +00:00
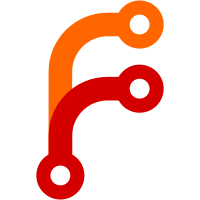
Wed Apr 20 14:53:35 1994 Bob Amstadt (bob@pooh) * [tools/build.c] [if1632/call.S] [if1632/Imakefile] Fixed bug for non-Linux systems. Apr 18, 94 martin2@trgcorp.solucorp.qc.ca (Martin Ayotte) * [windows/win.c] Bug fixed in CreateWindowEx() : Now use SetMenu() for menubar setup. New empty stub for function SetSysModalWindow(). * [misc/exec.c] New empty stub for function ExitWindows(). * [objects/font.c] New empty stub for function EnumFonts(). * New file [misc/property.c] New functions RemoveProp(), GetProp(), SetProp() & EnumProps(). * New file [misc/shell.c] New empty stubs for function RegisterShellProc(), ShellExecute() & ShellProc(). * New files [loader/task.c] & [include/task.h] Move functions GetWindowTask(), GetNumTask(), EnumTaskWindows() from 'loader/library.c'. * [if1632/user.c] [if1632/kernel.c] Put Atoms functions entries. * [controls/combo.c] New functions DirDlgSelectComboBox() & DirDlgListComboBox(). * [controls/listbox.c] New functions DirDlgSelect() & DirDlgList(). Sun Apr 17 20:57:59 1994 Erik Bos (erik@trashcan.hacktic.nl) * [objects/test.c] GrayString() added. * [if1632/callback.c] CallGrayStringProc() added. * [if1632/relay.c] [if1632/mmsystem.spec] Added. * [if1632/kernel.spec] [if1632/user.spec] Added forgotten specs for atom functions. Tue Apr 12 00:05:31 1994 Bob Amstadt (bob@pooh) * misc/spy.c (SpyInit): Added more message types * [windows/mdi.c] [include/mdi.h] Maximizing and restoring child windows. Tiling of child windows. Mon Apr 11 20:48:28 1994 Alexandre Julliard (julliard@lamisun.epfl.ch) * [windows/winpos.c] Revert focus and activation to previous window when hiding a window. * [windows/syscolor.c] Implemented system color objects (brushes and pens created at SetSysColor() time for better performance). * [windows/graphics.c] [windows/nonclient.c] [controls/button.c] Changed painting code to use system color objects. * [windows/message.c] New function MSG_InternalGetMessage() for internal messages loops (e.g. for dialogs or menus). * [windows/hook.c] [include/hook.h] (New files) Beginning of the window hooks implementation. * [windows/dialog.c] Use new function MSG_InternalGetMessage() in DialogBox(). * [if1632/callback.c] Added function CallHookProc(). Apr 11, 94 martin2@trgcorp.solucorp.qc.ca (Martin Ayotte) * [windows/event.c] Bug fix : WM_CHARs are sent to focused window like WM_KEY???. * [misc/exec.c] Nothing much more than a stub for LoadModule(), I saw there a lot to be done in that corner, I will come back later ... * [loader/library.c] New functions GetWindowTask(), GetNumTask(), EnumTaskWindows() and associated modules & tasks linked-lists. (it's only an 'emerging bud', more to come next weeks). * [loader/wine.c] Use LoadLibrary() instead of LoadImage() for 'sysres.dll'. * [control/menu.c] You can now click outside menu region without problem. Keyboard navig more smootly, even if a child has the focus. Bug fix in InsertItem(), (bad linklist when insert point not found). change Realloc for Free & Alloc in ModifyItem(). MF_STRING now set BLACK_PEN to fix bug of bad color of the underscores done by DrawText(), (maybe it should done in DrawText() itself ?). Sun Apr 10 14:06:08 1994 Erik Bos (erik@trashcan.hacktic.nl) * [misc/profile.c] .INI files will now be stored in / loaded from the windows dir if no path is supplied. * [if1632/kernel.spec] Fixed GetDriveType's prototype. * [if1632/winsock.spec] [include/winsock.h] [misc/winsocket.c] Fixed prototypes: winsock uses a word as socket handle not an int. * [misc/winsocket.c] Added heap allocation for returned structures. Added non-blocking WSAAsyncGetXbyY() functions as blocking ones. * [loader/wine.c] Added IsDLLLoaded(), used in LoadImage() to prevent loading a dll multiple times. Directory is added to wine's path when a fullpath is supplied when starting wine. LoadImage(): DLL filename used instead DLL's own internal name, fixes 'Bad DLL name' errors. Sat Apr 9 08:26:03 1994 David Metcalfe <david@prism.demon.co.uk> * [controls/edit.c] [controls/widgets.c] First release of edit control.
130 lines
3 KiB
C
130 lines
3 KiB
C
/*
|
|
* Windows Exec & Help
|
|
*
|
|
*/
|
|
|
|
#include <stdlib.h>
|
|
#include <stdio.h>
|
|
#include <string.h>
|
|
#include <unistd.h>
|
|
#include "windows.h"
|
|
|
|
#define HELP_CONTEXT 0x0001
|
|
#define HELP_QUIT 0x0002
|
|
#define HELP_INDEX 0x0003
|
|
#define HELP_CONTENTS 0x0003
|
|
#define HELP_HELPONHELP 0x0004
|
|
#define HELP_SETINDEX 0x0005
|
|
#define HELP_SETCONTENTS 0x0005
|
|
#define HELP_CONTEXTPOPUP 0x0008
|
|
#define HELP_FORCEFILE 0x0009
|
|
#define HELP_KEY 0x0101
|
|
#define HELP_COMMAND 0x0102
|
|
#define HELP_PARTIALKEY 0x0105
|
|
#define HELP_MULTIKEY 0x0201
|
|
#define HELP_SETWINPOS 0x0203
|
|
|
|
typedef struct {
|
|
WORD wEnvSeg;
|
|
LPSTR lpCmdLine;
|
|
LPVOID lpCmdShow;
|
|
DWORD dwReserved;
|
|
} PARAMBLOCK;
|
|
|
|
HANDLE CreateNewTask(HINSTANCE hInst);
|
|
|
|
/**********************************************************************
|
|
* LoadModule [KERNEL.45]
|
|
*/
|
|
HANDLE LoadModule(LPSTR modulefile, LPVOID lpParamBlk)
|
|
{
|
|
PARAMBLOCK *pblk = lpParamBlk;
|
|
WORD *lpCmdShow;
|
|
printf("LoadModule '%s' %08X\n", modulefile, lpParamBlk);
|
|
if (lpParamBlk == NULL) return 0;
|
|
lpCmdShow = (WORD *)pblk->lpCmdShow;
|
|
return WinExec(pblk->lpCmdLine, lpCmdShow[1]);
|
|
}
|
|
|
|
|
|
/**********************************************************************
|
|
* WinExec [KERNEL.166]
|
|
*/
|
|
WORD WinExec(LPSTR lpCmdLine, WORD nCmdShow)
|
|
{
|
|
int X, X2, C;
|
|
char *ArgV[20];
|
|
HANDLE hTask = 0;
|
|
printf("WinExec('%s', %04X)\n", lpCmdLine, nCmdShow);
|
|
ArgV[0] = "wine";
|
|
C = 1;
|
|
for (X = X2 = 0; X < strlen(lpCmdLine) + 1; X++) {
|
|
if ((lpCmdLine[X] == ' ') || (lpCmdLine[X] == '\0')) {
|
|
ArgV[C] = (char *)malloc(X - X2 + 1);
|
|
strncpy(ArgV[C], &lpCmdLine[X2], X - X2);
|
|
ArgV[C][X - X2] = '\0';
|
|
C++; X2 = X + 1;
|
|
}
|
|
}
|
|
ArgV[C] = NULL;
|
|
for (C = 0; ; C++) {
|
|
if (ArgV[C] == NULL) break;
|
|
printf("--> '%s' \n", ArgV[C]);
|
|
}
|
|
switch(fork()) {
|
|
case -1:
|
|
printf("Can't 'fork' process !\n");
|
|
break;
|
|
case 0:
|
|
hTask = CreateNewTask(0);
|
|
printf("WinExec // New Task hTask=%04X !\n", hTask);
|
|
execvp(ArgV[0], ArgV);
|
|
printf("Child process died !\n");
|
|
exit(1);
|
|
default:
|
|
printf("WinExec (Main process stay alive) hTask=%04X !\n", hTask);
|
|
break;
|
|
}
|
|
for (C = 0; ; C++) {
|
|
if (ArgV[C] == NULL) break;
|
|
free(ArgV[C]);
|
|
}
|
|
return hTask;
|
|
}
|
|
|
|
|
|
/**********************************************************************
|
|
* ExitWindows [USER.7]
|
|
*/
|
|
BOOL ExitWindows(DWORD dwReserved, WORD wRetCode)
|
|
{
|
|
printf("EMPTY STUB !!! ExitWindows(%08X, %04X) !\n", dwReserved, wRetCode);
|
|
}
|
|
|
|
|
|
/**********************************************************************
|
|
* WinHelp [USER.171]
|
|
*/
|
|
BOOL WinHelp(HWND hWnd, LPSTR lpHelpFile, WORD wCommand, DWORD dwData)
|
|
{
|
|
char str[256];
|
|
printf("WinHelp(%s, %u, %lu)\n", lpHelpFile, wCommand, dwData);
|
|
switch(wCommand) {
|
|
case 0:
|
|
case HELP_HELPONHELP:
|
|
GetWindowsDirectory(str, sizeof(str));
|
|
strcat(str, "\\winhelp.exe");
|
|
printf("'%s'\n", str);
|
|
break;
|
|
case HELP_INDEX:
|
|
GetWindowsDirectory(str, sizeof(str));
|
|
strcat(str, "\\winhelp.exe");
|
|
printf("'%s'\n", str);
|
|
break;
|
|
default:
|
|
return FALSE;
|
|
}
|
|
WinExec(str, SW_SHOWNORMAL);
|
|
return(TRUE);
|
|
}
|