mirror of
git://source.winehq.org/git/wine.git
synced 2024-09-14 17:16:18 +00:00
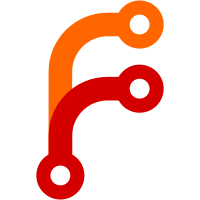
Sun Oct 12 15:03:01 1997 Alexandre Julliard <julliard@lrc.epfl.ch> * [if1632/builtin.c] [if1632/relay.c] Relay debugging entry points are now generated on the fly for Win32 DLLs. * [include/stackframe.h] Added VA_LIST16 type and macros to access arguments on the 16-bit stack. * [memory/global.c] Fixed GlobalHandle32 to work with fixed blocks. * [misc/ddeml.c] (New file) Added a lot of stubs for DDEML functions. * [objects/dc.c] Added Get/SetGraphicsMode(). * [objects/gdiobj.c] [windows/winpos.c] Added a few stubs. * [tools/build.c] Removed 'byte', 'word', 'long' and 'return' entry points for Win32. 'register' functions can no longer take arguments in Win32. The Win32 NE module is now generated by MODULE_CreateDummyModule. CallFrom32 callbacks removed except for register functions. Fri Oct 10 18:22:18 1997 John Harvey <john@division.co.uk> * [graphics/win16drv/Makefile.in] [graphics/win16drv/brush.c] [graphics/win16drv/graphics.c] [graphics/win16drv/init.c] [graphics/win16drv/objects.c] [graphics/win16drv/pen.c] [graphics/win16drv/prtdrv.c] [graphics/win16drv/text.c] [include/callback.h] [include/win16drv.h] Added support for pens and brushes in SelectObject. Added support for LineTo, MoveToEx, PatBlt (very preliminary), Polygon and Rectangle. Text is drawn in the correct place more often. These changes may only work with the Windows Postscript driver since many other drivers now need more GDI support. Tue Oct 7 21:06:23 1997 Kristian Nielsen <kristian.nielsen@risoe.dk> * [debugger/expr.c] Fixed typo for the >> operator. * [loader/task.c] Fixed SwitchStackTo(); it used to return with the new stack placed four bytes too high in memory. * [loader/ne_resource.c] Removed problematic nametable code introduced in Wine 970914. Tue Oct 7 02:24:12 1997 Dimitrie O. Paun <dimi@cs.toronto.edu> * [controls/commctrl.c] Added this files to hold functions from the comctl32.dll Added to this files some functions scattered in different places (such as InitCommonControls) and added some new ones as well. * [include/syscolor.h] [windows/syscolor.c] Added proper entries for all possible COLOR_* values. * [objects/brush.c] Modified GetSysColorBrush to return the correct brush for all possible COLOR_* constants. Sat Oct 4 23:35:20 1997 U.Bonnes <bon@elektron.ikp.physik.th-darmstadt.de> * [loader/module.c] [scheduler/process.c] [win32/environment.c] Another approach to get access to an unrestricted commandline. * [misc/crtdll.c] Make fclose work again. * [if1632/crtdll.spec] Use sprintf for crtdll-sprintf again as e.g. %g is not available for wsprintf. * [misc/wsprintf.c] Make WPR_STRING work in more situations. Added debug output for the wsprintf functions. * [misc/crtdll.c] [misc/main.c] Use argv[0] as comand with CRTDLL_system. Fri Oct 3 14:00:29 MET DST 1997 Jan Willamowius <jan@janhh.shnet.org> * [*/*] Removed some compiler warnings. * [msdos/int15.c] New INT 15 handler.
387 lines
9.5 KiB
C
387 lines
9.5 KiB
C
/*
|
|
* Generate include file dependencies
|
|
*
|
|
* Copyright 1996 Alexandre Julliard
|
|
*/
|
|
|
|
#include <ctype.h>
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
#include <unistd.h>
|
|
|
|
/* Max first-level includes per file */
|
|
#define MAX_INCLUDES 124
|
|
|
|
typedef struct _INCL_FILE
|
|
{
|
|
struct _INCL_FILE *next;
|
|
char *name;
|
|
char *filename;
|
|
struct _INCL_FILE *owner;
|
|
struct _INCL_FILE *files[MAX_INCLUDES];
|
|
} INCL_FILE;
|
|
|
|
static INCL_FILE *firstSrc;
|
|
static INCL_FILE *firstInclude;
|
|
|
|
typedef struct _INCL_PATH
|
|
{
|
|
struct _INCL_PATH *next;
|
|
const char *name;
|
|
} INCL_PATH;
|
|
|
|
static INCL_PATH *firstPath;
|
|
|
|
static const char *SrcDir = NULL;
|
|
static const char *OutputFileName = "Makefile";
|
|
static const char *Separator = "### Dependencies";
|
|
static const char *ProgramName;
|
|
|
|
static const char Usage[] =
|
|
"Usage: %s [options] [files]\n"
|
|
"Options:\n"
|
|
" -Idir Search for include files in directory 'dir'\n"
|
|
" -Cdir Search for source files in directory 'dir'\n"
|
|
" -fxxx Store output in file 'xxx' (default: Makefile)\n"
|
|
" -sxxx Use 'xxx' as separator (default: \"### Dependencies\")\n";
|
|
|
|
|
|
/*******************************************************************
|
|
* xmalloc
|
|
*/
|
|
static void *xmalloc( int size )
|
|
{
|
|
void *res;
|
|
if (!(res = malloc (size ? size : 1)))
|
|
{
|
|
fprintf( stderr, "%s: Virtual memory exhausted.\n", ProgramName );
|
|
exit(1);
|
|
}
|
|
return res;
|
|
}
|
|
|
|
|
|
/*******************************************************************
|
|
* xstrdup
|
|
*/
|
|
static char *xstrdup( const char *str )
|
|
{
|
|
char *res = strdup( str );
|
|
if (!res)
|
|
{
|
|
fprintf( stderr, "%s: Virtual memory exhausted.\n", ProgramName );
|
|
exit(1);
|
|
}
|
|
return res;
|
|
}
|
|
|
|
|
|
/*******************************************************************
|
|
* add_include_path
|
|
*
|
|
* Add a directory to the include path.
|
|
*/
|
|
static void add_include_path( const char *name )
|
|
{
|
|
INCL_PATH *path = xmalloc( sizeof(*path) );
|
|
INCL_PATH **p = &firstPath;
|
|
while (*p) p = &(*p)->next;
|
|
*p = path;
|
|
path->next = NULL;
|
|
path->name = name;
|
|
}
|
|
|
|
|
|
/*******************************************************************
|
|
* add_src_file
|
|
*
|
|
* Add a source file to the list.
|
|
*/
|
|
static INCL_FILE *add_src_file( const char *name )
|
|
{
|
|
INCL_FILE **p = &firstSrc;
|
|
INCL_FILE *file = xmalloc( sizeof(*file) );
|
|
memset( file, 0, sizeof(*file) );
|
|
file->name = xstrdup(name);
|
|
while (*p) p = &(*p)->next;
|
|
*p = file;
|
|
return file;
|
|
}
|
|
|
|
|
|
/*******************************************************************
|
|
* add_include
|
|
*
|
|
* Add an include file if it doesn't already exists.
|
|
*/
|
|
static INCL_FILE *add_include( INCL_FILE *pFile, const char *name )
|
|
{
|
|
INCL_FILE **p = &firstInclude;
|
|
int pos;
|
|
|
|
for (pos = 0; pos < MAX_INCLUDES; pos++) if (!pFile->files[pos]) break;
|
|
if (pos >= MAX_INCLUDES)
|
|
{
|
|
fprintf( stderr, "%s: %s: too many included files, please fix MAX_INCLUDES\n",
|
|
ProgramName, pFile->name );
|
|
exit(1);
|
|
}
|
|
|
|
while (*p && strcmp( name, (*p)->name )) p = &(*p)->next;
|
|
if (!*p)
|
|
{
|
|
*p = xmalloc( sizeof(INCL_FILE) );
|
|
memset( *p, 0, sizeof(INCL_FILE) );
|
|
(*p)->name = xstrdup(name);
|
|
}
|
|
pFile->files[pos] = *p;
|
|
return *p;
|
|
}
|
|
|
|
|
|
/*******************************************************************
|
|
* open_src_file
|
|
*/
|
|
static FILE *open_src_file( INCL_FILE *pFile )
|
|
{
|
|
FILE *file;
|
|
|
|
if (SrcDir)
|
|
{
|
|
pFile->filename = xmalloc( strlen(SrcDir) + strlen(pFile->name) + 2 );
|
|
strcpy( pFile->filename, SrcDir );
|
|
strcat( pFile->filename, "/" );
|
|
strcat( pFile->filename, pFile->name );
|
|
}
|
|
else pFile->filename = xstrdup( pFile->name );
|
|
|
|
if (!(file = fopen( pFile->filename, "r" )))
|
|
{
|
|
perror( pFile->filename );
|
|
exit(1);
|
|
}
|
|
return file;
|
|
}
|
|
|
|
|
|
/*******************************************************************
|
|
* open_include_file
|
|
*/
|
|
static FILE *open_include_file( INCL_FILE *pFile )
|
|
{
|
|
FILE *file = NULL;
|
|
INCL_PATH *path;
|
|
|
|
for (path = firstPath; path; path = path->next)
|
|
{
|
|
char *filename = xmalloc(strlen(path->name) + strlen(pFile->name) + 2);
|
|
strcpy( filename, path->name );
|
|
strcat( filename, "/" );
|
|
strcat( filename, pFile->name );
|
|
if ((file = fopen( filename, "r" )))
|
|
{
|
|
pFile->filename = filename;
|
|
break;
|
|
}
|
|
free( filename );
|
|
}
|
|
if (!file)
|
|
{
|
|
if (firstPath) perror( pFile->name );
|
|
else fprintf( stderr, "%s: %s: File not found\n",
|
|
ProgramName, pFile->name );
|
|
exit(1);
|
|
}
|
|
return file;
|
|
}
|
|
|
|
|
|
/*******************************************************************
|
|
* parse_file
|
|
*/
|
|
static void parse_file( INCL_FILE *pFile, int src )
|
|
{
|
|
char buffer[1024];
|
|
char *include;
|
|
int line = 0;
|
|
|
|
FILE *file = src ? open_src_file( pFile ) : open_include_file( pFile );
|
|
while (fgets( buffer, sizeof(buffer)-1, file ))
|
|
{
|
|
char *p = buffer;
|
|
line++;
|
|
while (*p && isspace(*p)) p++;
|
|
if (*p++ != '#') continue;
|
|
while (*p && isspace(*p)) p++;
|
|
if (strncmp( p, "include", 7 )) continue;
|
|
p += 7;
|
|
while (*p && isspace(*p)) p++;
|
|
if (*p++ != '\"') continue;
|
|
include = p;
|
|
while (*p && (*p != '\"')) p++;
|
|
if (!*p)
|
|
{
|
|
fprintf( stderr, "%s:%d: Malformed #include directive\n",
|
|
pFile->filename, line );
|
|
exit(1);
|
|
}
|
|
*p = 0;
|
|
add_include( pFile, include );
|
|
}
|
|
fclose(file);
|
|
}
|
|
|
|
|
|
/*******************************************************************
|
|
* output_include
|
|
*/
|
|
static void output_include( FILE *file, INCL_FILE *pFile,
|
|
INCL_FILE *owner, int *column )
|
|
{
|
|
int i;
|
|
|
|
if (pFile->owner == owner) return;
|
|
pFile->owner = owner;
|
|
if (*column + strlen(pFile->filename) + 1 > 70)
|
|
{
|
|
fprintf( file, " \\\n" );
|
|
*column = 0;
|
|
}
|
|
fprintf( file, " %s", pFile->filename );
|
|
*column += strlen(pFile->filename) + 1;
|
|
for (i = 0; i < MAX_INCLUDES; i++)
|
|
if (pFile->files[i]) output_include( file, pFile->files[i],
|
|
owner, column );
|
|
}
|
|
|
|
|
|
/*******************************************************************
|
|
* output_src
|
|
*/
|
|
static void output_src( FILE *file, INCL_FILE *pFile, int *column )
|
|
{
|
|
char *name = strrchr( pFile->name, '/' );
|
|
char *obj = xstrdup( name ? name + 1 : pFile->name );
|
|
char *ext = strrchr( obj, '.' );
|
|
if (ext)
|
|
{
|
|
if (!strcmp( ext, ".y" )) /* yacc file */
|
|
{
|
|
fprintf( file, "y.tab.o: y.tab.c" );
|
|
*column += 16;
|
|
}
|
|
else if (!strcmp( ext, ".l" )) /* lex file */
|
|
{
|
|
fprintf( file, "lex.yy.o: lex.yy.c" );
|
|
*column += 18;
|
|
}
|
|
else if (!strcmp( ext, ".rc" )) /* resource file */
|
|
{
|
|
*ext = '\0';
|
|
fprintf( file, "%s.c %s.h: %s", obj, obj, pFile->filename );
|
|
*column += 2 * strlen(obj) + strlen(pFile->filename) + 7;
|
|
}
|
|
else
|
|
{
|
|
strcpy( ext, ".o" );
|
|
fprintf( file, "%s: %s", obj, pFile->filename );
|
|
*column += strlen(obj) + strlen(pFile->filename) + 2;
|
|
}
|
|
}
|
|
free( obj );
|
|
}
|
|
|
|
|
|
/*******************************************************************
|
|
* output_dependencies
|
|
*/
|
|
static void output_dependencies(void)
|
|
{
|
|
INCL_FILE *pFile;
|
|
int i, column;
|
|
FILE *file = NULL;
|
|
char buffer[1024];
|
|
|
|
if (Separator && ((file = fopen( OutputFileName, "r+" ))))
|
|
{
|
|
while (fgets( buffer, sizeof(buffer), file ))
|
|
if (!strncmp( buffer, Separator, strlen(Separator) )) break;
|
|
ftruncate( fileno(file), ftell(file) );
|
|
fseek( file, 0L, SEEK_END );
|
|
}
|
|
if (!file)
|
|
{
|
|
if (!(file = fopen( OutputFileName, "a" )))
|
|
{
|
|
perror( OutputFileName );
|
|
exit(1);
|
|
}
|
|
}
|
|
for( pFile = firstSrc; pFile; pFile = pFile->next)
|
|
{
|
|
column = 0;
|
|
output_src( file, pFile, &column );
|
|
for (i = 0; i < MAX_INCLUDES; i++)
|
|
if (pFile->files[i]) output_include( file, pFile->files[i],
|
|
pFile, &column );
|
|
fprintf( file, "\n" );
|
|
}
|
|
fclose(file);
|
|
}
|
|
|
|
|
|
/*******************************************************************
|
|
* parse_option
|
|
*/
|
|
static void parse_option( const char *opt )
|
|
{
|
|
switch(opt[1])
|
|
{
|
|
case 'I':
|
|
if (opt[2]) add_include_path( opt + 2 );
|
|
break;
|
|
case 'C':
|
|
if (opt[2]) SrcDir = opt + 2;
|
|
else SrcDir = NULL;
|
|
break;
|
|
case 'f':
|
|
if (opt[2]) OutputFileName = opt + 2;
|
|
break;
|
|
case 's':
|
|
if (opt[2]) Separator = opt + 2;
|
|
else Separator = NULL;
|
|
break;
|
|
default:
|
|
fprintf( stderr, "Unknown option '%s'\n", opt );
|
|
fprintf( stderr, Usage, ProgramName );
|
|
exit(1);
|
|
}
|
|
}
|
|
|
|
|
|
/*******************************************************************
|
|
* main
|
|
*/
|
|
int main( int argc, char *argv[] )
|
|
{
|
|
INCL_FILE *pFile;
|
|
|
|
ProgramName = argv[0];
|
|
while (argc > 1)
|
|
{
|
|
if (*argv[1] == '-') parse_option( argv[1] );
|
|
else
|
|
{
|
|
pFile = add_src_file( argv[1] );
|
|
parse_file( pFile, 1 );
|
|
}
|
|
argc--;
|
|
argv++;
|
|
}
|
|
for (pFile = firstInclude; pFile; pFile = pFile->next)
|
|
parse_file( pFile, 0 );
|
|
output_dependencies();
|
|
return 0;
|
|
}
|