mirror of
git://source.winehq.org/git/wine.git
synced 2024-11-05 18:01:34 +00:00
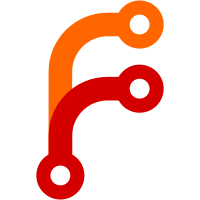
Sat Feb 24 16:17:05 1996 Alexandre Julliard <julliard@lrc.epfl.ch> * [files/profile.c] Added \r when writing profile files, for DOS compatibility. * [memory/global.c] Fixed bug in GlobalReAlloc() that caused a discarded block not to be reallocated if its size was not changed. * [memory/selector.c] Avoid setting a valid LDT entry with base and limit set to 0, as this causes the kernel to clear the entry. This fixes a crash when exiting Windows program manager. * [objects/metafile.c] Removed call to creat() instead of _lcreat() for WINELIB. Fri Feb 23 00:35:54 1996 Thomas Sandford <tdgsandf@prds-grn.demon.co.uk> * [if1632/gdi32.spec] GetTextExtentPointA now has win32 specific implementation. * [include/struct32.h] Define new structure tagSIZE32 and typedef SIZE32 to it. Define prototype for function PARAM32_SIZE16to32 * [win32/param32.c] New functions PARAM32_SIZE16to32 and WIN32_GetTextExtentPointA * [win32/memory.c] Added missing file pointer parameter to fprintf. Thu Feb 22 01:14:21 1996 Eric Warnke <ew2193@csc.albany.edu> * [windows/nonclient.c] Added more familiar icon activity, ie single click brings up system menu. Wed Feb 21 13:07:04 1996 Frans van Dorsselaer <dorssel@rulhm1.leidenuniv.nl> * [controls/menu.c] Added calls to HideCaret() and ShowCaret() from within TrackPopupMenu(), MENU_TrackMouseMenuBar() and MENU_TrackKbdMenuBar(). Are there any more places where this should be done? * [controls/static.c] Fixed a FIXME in STATIC_SetIcon(), which now returns a handle to the previous icon. Added a new FIXME at the point where WM_SETTEXT is handled for a SS_ICON static control. * [misc/commdlg.c] Implemented FindText() and ReplaceText() Still missing : Templates and Hooks handling / error checking * [resources/sysres_En.c] Redesigned FIND_TEXT and REPLACE_TEXT dialogs, so they now work. Languages other than En should update these too, though, as well as redimension the controls because some of the text doesn't fit. Created file resources/TODO to explain this. * [windows/caret.c] Re-written. It now uses the correct R2_XORPEN. It resets the blink timer on SetCaretPos(). It does its own hide/show scheme when SetCaretPos() is called (should be faster). Mon Feb 19 21:50:00 1996 Alex Korobka <alex@phm30.pharm.sunysb.edu> * [controls/listbox.c] Miscellaneous changes for better LBS_EXTENDEDSEL support. Removed several superfluous redrawals of item list. * [controls/scroll.c] WM_GETDLGCODE return value. * [windows/win.c] FlashWindow function. * [windows/painting.c] [windows/scroll.c] Added HideCaret/ShowCaret calls. * [objects/font.c] Added GetCharABCWidths stub. * [include/windows.h] "#define"s needed for changes mentioned above. Mon Feb 19 20:12:03 1996 Hans de Graaff <Hans.deGraaff@twi72.twi.tudelft.nl> * [include/winsock.h] Change order of includes to get in_addr struct defined in time. (Note: Linux 1.3.66, libc 5.2.18) * [misc/main.c] [include/options.h] [miscemu/int2f.c] Changed the -enhanced option into a -mode option, which can be either 'standard' or 'enhanced'. 'enhanced' is the default.
183 lines
5.3 KiB
C
183 lines
5.3 KiB
C
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
#include <unistd.h>
|
|
#include "registers.h"
|
|
#include "ldt.h"
|
|
#include "wine.h"
|
|
#include "drive.h"
|
|
#include "msdos.h"
|
|
#include "miscemu.h"
|
|
#include "module.h"
|
|
#include "options.h"
|
|
#include "stddebug.h"
|
|
/* #define DEBUG_INT */
|
|
#include "debug.h"
|
|
|
|
/* base WINPROC module number for VxDs */
|
|
#define VXD_BASE 400
|
|
|
|
static void do_int2f_16(struct sigcontext_struct *context);
|
|
void do_mscdex(struct sigcontext_struct *context);
|
|
|
|
/**********************************************************************
|
|
* INT_Int2fHandler
|
|
*
|
|
* Handler for int 2fh (multiplex).
|
|
*/
|
|
void INT_Int2fHandler( struct sigcontext_struct context )
|
|
{
|
|
switch(AH_reg(&context))
|
|
{
|
|
case 0x10:
|
|
AL_reg(&context) = 0xff; /* share is installed */
|
|
break;
|
|
|
|
case 0x15: /* mscdex */
|
|
do_mscdex(&context);
|
|
break;
|
|
|
|
case 0x16:
|
|
do_int2f_16( &context );
|
|
break;
|
|
|
|
case 0x4a:
|
|
switch(AL_reg(&context))
|
|
{
|
|
case 0x10: /* smartdrv */
|
|
break; /* not installed */
|
|
case 0x11: /* dblspace */
|
|
break; /* not installed */
|
|
case 0x12: /* realtime compression interface */
|
|
break; /* not installed */
|
|
default:
|
|
INT_BARF( &context, 0x2f );
|
|
}
|
|
break;
|
|
default:
|
|
INT_BARF( &context, 0x2f );
|
|
break;
|
|
}
|
|
}
|
|
|
|
|
|
/**********************************************************************
|
|
* do_int2f_16
|
|
*/
|
|
static void do_int2f_16(struct sigcontext_struct *context)
|
|
{
|
|
DWORD addr;
|
|
|
|
switch(AL_reg(context))
|
|
{
|
|
case 0x00: /* Windows enhanced mode installation check */
|
|
AX_reg(context) = (Options.mode == MODE_ENHANCED) ? WINVERSION : 0;
|
|
break;
|
|
|
|
case 0x0a: /* Get Windows version and type */
|
|
AX_reg(context) = 0;
|
|
BX_reg(context) = (WINVERSION >> 8) | ((WINVERSION << 8) & 0xff00);
|
|
CX_reg(context) = (Options.mode == MODE_ENHANCED) ? 3 : 2;
|
|
break;
|
|
|
|
case 0x80: /* Release time-slice */
|
|
AL_reg(context) = 0;
|
|
/* FIXME: We need to do something that lets some other process run
|
|
here. */
|
|
sleep(0);
|
|
break;
|
|
|
|
case 0x83: /* Return Current Virtual Machine ID */
|
|
/* Virtual Machines are usually created/destroyed when Windows runs
|
|
* DOS programs. Since we never do, we are always in the System VM.
|
|
* According to Ralf Brown's Interrupt List, never return 0. But it
|
|
* seems to work okay (returning 0), just to be sure we return 1.
|
|
*/
|
|
BX_reg(context) = 1; /* VM 1 is probably the System VM */
|
|
break;
|
|
|
|
case 0x84: /* Get device API entry point */
|
|
addr = MODULE_GetEntryPoint( GetModuleHandle("WINPROCS"),
|
|
VXD_BASE + BX_reg(context) );
|
|
if (!addr) /* not supported */
|
|
{
|
|
fprintf( stderr,"Application attempted to access VxD %04x\n",
|
|
BX_reg(context) );
|
|
fprintf( stderr,"This device is not known to Wine.");
|
|
fprintf( stderr,"Expect a failure now\n");
|
|
}
|
|
ES_reg(context) = SELECTOROF(addr);
|
|
DI_reg(context) = OFFSETOF(addr);
|
|
break;
|
|
|
|
case 0x86: /* DPMI detect mode */
|
|
AX_reg(context) = 0; /* Running under DPMI */
|
|
break;
|
|
|
|
/* FIXME: is this right? Specs say that this should only be callable
|
|
in real (v86) mode which we never enter. */
|
|
case 0x87: /* DPMI installation check */
|
|
AX_reg(context) = 0x0000; /* DPMI Installed */
|
|
BX_reg(context) = 0x0001; /* 32bits available */
|
|
CL_reg(context) = runtime_cpu();
|
|
DX_reg(context) = 0x005a; /* DPMI major/minor 0.90 */
|
|
SI_reg(context) = 0; /* # of para. of DOS extended private data */
|
|
ES_reg(context) = 0; /* ES:DI is DPMI switch entry point */
|
|
DI_reg(context) = 0;
|
|
break;
|
|
|
|
case 0x8a: /* DPMI get vendor-specific API entry point. */
|
|
/* The 1.0 specs say this should work with all 0.9 hosts. */
|
|
break;
|
|
|
|
default:
|
|
INT_BARF( context, 0x2f );
|
|
}
|
|
}
|
|
|
|
void do_mscdex(struct sigcontext_struct *context)
|
|
{
|
|
int drive, count;
|
|
char *p;
|
|
|
|
switch(AL_reg(context))
|
|
{
|
|
case 0x00: /* Installation check */
|
|
/* Count the number of contiguous CDROM drives
|
|
*/
|
|
for (drive = count = 0; drive < MAX_DOS_DRIVES; drive++)
|
|
{
|
|
if (DRIVE_GetType(drive) == TYPE_CDROM)
|
|
{
|
|
while (DRIVE_GetType(drive + count) == TYPE_CDROM) count++;
|
|
break;
|
|
}
|
|
}
|
|
|
|
BX_reg(context) = count;
|
|
CX_reg(context) = (drive < MAX_DOS_DRIVES) ? drive : 0;
|
|
break;
|
|
|
|
case 0x0B: /* drive check */
|
|
AX_reg(context) = (DRIVE_GetType(CX_reg(context)) == TYPE_CDROM);
|
|
BX_reg(context) = 0xADAD;
|
|
break;
|
|
|
|
case 0x0C: /* get version */
|
|
BX_reg(context) = 0x020a;
|
|
break;
|
|
|
|
case 0x0D: /* get drive letters */
|
|
p = PTR_SEG_OFF_TO_LIN(ES_reg(context), BX_reg(context));
|
|
memset( p, 0, MAX_DOS_DRIVES );
|
|
for (drive = 0; drive < MAX_DOS_DRIVES; drive++)
|
|
{
|
|
if (DRIVE_GetType(drive) == TYPE_CDROM) *p++ = drive;
|
|
}
|
|
break;
|
|
|
|
default:
|
|
fprintf(stderr, "Unimplemented MSCDEX function 0x%02.2X.\n", AL_reg(context));
|
|
break;
|
|
}
|
|
}
|