mirror of
https://gitlab.freedesktop.org/wayland/weston
synced 2024-07-22 11:14:21 +00:00
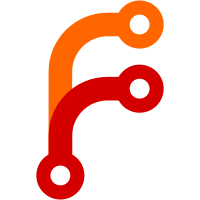
Add yet another flavor of assertion macros. Unlike libc assert.h assert(), these cannot be easily disabled by the build. They also print both the implied expression and the compared values. Unlike ZUC macros, there is much less framework code and it can handle also floating-point types. The function custom_assert_fail_ can be redefined, meaning that different compilation units can do different things on failure. Also the 'compositor' parameter was added to the new macros because we plan to use these asserts in our log infrastructure, and we want to print the "failure" messages in the right log scopes. Having the compositor already in the macros will avoid double work. Another future possibility is to write specific asserts for the test suite. So we would be able to write a test suite failure function that just print what "failed" without aborting. There is also limited support for custom types. These are actually pretty similar to libinput's litest macros. Signed-off-by: Pekka Paalanen <pekka.paalanen@collabora.com> Signed-off-by: Leandro Ribeiro <leandro.ribeiro@collabora.com>
93 lines
3 KiB
C
93 lines
3 KiB
C
/*
|
|
* Copyright 2022 Collabora, Ltd.
|
|
*
|
|
* Permission is hereby granted, free of charge, to any person obtaining
|
|
* a copy of this software and associated documentation files (the
|
|
* "Software"), to deal in the Software without restriction, including
|
|
* without limitation the rights to use, copy, modify, merge, publish,
|
|
* distribute, sublicense, and/or sell copies of the Software, and to
|
|
* permit persons to whom the Software is furnished to do so, subject to
|
|
* the following conditions:
|
|
*
|
|
* The above copyright notice and this permission notice (including the
|
|
* next paragraph) shall be included in all copies or substantial
|
|
* portions of the Software.
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
|
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
|
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
|
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS
|
|
* BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN
|
|
* ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
|
|
* CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
|
* SOFTWARE.
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <stdarg.h>
|
|
#include <string.h>
|
|
|
|
struct weston_compositor;
|
|
|
|
__attribute__((noreturn, format(printf, 2, 3)))
|
|
static inline void
|
|
weston_assert_fail_(const struct weston_compositor *compositor, const char *fmt, ...)
|
|
{
|
|
va_list ap;
|
|
|
|
va_start(ap, fmt);
|
|
vfprintf(stderr, fmt, ap);
|
|
va_end(ap);
|
|
|
|
abort();
|
|
}
|
|
|
|
#ifndef custom_assert_fail_
|
|
#define custom_assert_fail_ weston_assert_fail_
|
|
#endif
|
|
|
|
#define weston_assert_(compositor, a, b, val_type, val_fmt, cmp) \
|
|
({ \
|
|
struct weston_compositor *ec = compositor; \
|
|
val_type a_ = (a); \
|
|
val_type b_ = (b); \
|
|
bool cond = a_ cmp b_; \
|
|
if (!cond) \
|
|
custom_assert_fail_(ec, "%s:%u: Assertion %s %s %s (" val_fmt " %s " val_fmt ") failed!\n", \
|
|
__FILE__, __LINE__, #a, #cmp, #b, a_, #cmp, b_); \
|
|
cond; \
|
|
})
|
|
|
|
#define weston_assert_fn_(compositor, fn, a, b, val_type, val_fmt, cmp) \
|
|
({ \
|
|
struct weston_compositor *ec = compositor; \
|
|
val_type a_ = (a); \
|
|
val_type b_ = (b); \
|
|
bool cond = fn(a_, b_) cmp 0; \
|
|
if (!cond) \
|
|
custom_assert_fail_(ec, "%s:%u: Assertion %s %s %s (" val_fmt " %s " val_fmt ") failed!\n", \
|
|
__FILE__, __LINE__, #a, #cmp, #b, a_, #cmp, b_); \
|
|
cond; \
|
|
})
|
|
|
|
#define weston_assert_true(compositor, a) \
|
|
weston_assert_(compositor, a, true, bool, "%d", ==)
|
|
|
|
#define weston_assert_ptr(compositor, a) \
|
|
weston_assert_(compositor, a, NULL, const void *, "%p", !=)
|
|
|
|
#define weston_assert_ptr_is_null(compositor, a) \
|
|
weston_assert_(compositor, a, NULL, const void *, "%p", ==)
|
|
|
|
#define weston_assert_ptr_eq(compositor, a, b) \
|
|
weston_assert_(compositor, a, b, const void *, "%p", ==)
|
|
|
|
#define weston_assert_double_eq(compositor, a, b) \
|
|
weston_assert_(compositor, a, b, double, "%.10g", ==)
|
|
|
|
#define weston_assert_str_eq(compositor, a, b) \
|
|
weston_assert_fn_(compositor, strcmp, a, b, const char *, "%s", ==)
|