mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-22 02:26:11 +00:00
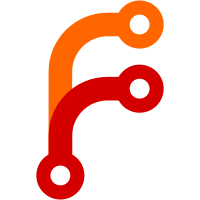
Similar to POSIX read, the basic read and write functions of AK::Stream do not have a lower limit of how much data they read or write (apart from "none at all"). Rename the functions to "read some [data]" and "write some [data]" (with "data" being omitted, since everything here is reading and writing data) to make them sufficiently distinct from the functions that ensure to use the entire buffer (which should be the go-to function for most usages). No functional changes, just a lot of new FIXMEs.
50 lines
1.4 KiB
C++
50 lines
1.4 KiB
C++
/*
|
|
* Copyright (c) 2018-2021, Andreas Kling <kling@serenityos.org>
|
|
* Copyright (c) 2022, Lucas Chollet <lucas.chollet@free.fr>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <AK/Vector.h>
|
|
#include <LibCore/ArgsParser.h>
|
|
#include <LibCore/File.h>
|
|
#include <LibCore/System.h>
|
|
#include <LibMain/Main.h>
|
|
|
|
ErrorOr<int> serenity_main(Main::Arguments arguments)
|
|
{
|
|
TRY(Core::System::pledge("stdio rpath"));
|
|
|
|
Vector<StringView> paths;
|
|
|
|
Core::ArgsParser args_parser;
|
|
args_parser.set_general_help("Concatenate files or pipes to stdout.");
|
|
args_parser.add_positional_argument(paths, "File path", "path", Core::ArgsParser::Required::No);
|
|
args_parser.parse(arguments);
|
|
|
|
if (paths.is_empty())
|
|
paths.append("-"sv);
|
|
|
|
Vector<NonnullOwnPtr<Core::File>> files;
|
|
TRY(files.try_ensure_capacity(paths.size()));
|
|
|
|
for (auto const& path : paths) {
|
|
if (auto result = Core::File::open_file_or_standard_stream(path, Core::File::OpenMode::Read); result.is_error())
|
|
warnln("Failed to open {}: {}", path, result.release_error());
|
|
else
|
|
files.unchecked_append(result.release_value());
|
|
}
|
|
|
|
TRY(Core::System::pledge("stdio"));
|
|
|
|
Array<u8, 32768> buffer;
|
|
for (auto const& file : files) {
|
|
while (!file->is_eof()) {
|
|
auto const buffer_span = TRY(file->read_some(buffer));
|
|
out("{:s}", buffer_span);
|
|
}
|
|
}
|
|
|
|
return files.size() != paths.size();
|
|
}
|