mirror of
https://github.com/SerenityOS/serenity
synced 2024-09-06 17:06:31 +00:00
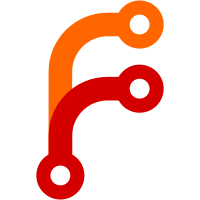
These instances were detected by searching for files that include AK/Format.h, but don't match the regex: \\b(CheckedFormatString|critical_dmesgln|dbgln|dbgln_if|dmesgln|FormatBu ilder|__FormatIfSupported|FormatIfSupported|FormatParser|FormatString|Fo rmattable|Formatter|__format_value|HasFormatter|max_format_arguments|out |outln|set_debug_enabled|StandardFormatter|TypeErasedFormatParams|TypeEr asedParameter|VariadicFormatParams|v_critical_dmesgln|vdbgln|vdmesgln|vf ormat|vout|warn|warnln|warnln_if)\\b (Without the linebreaks.) This regex is pessimistic, so there might be more files that don't actually use any formatting functions. Observe that this revealed that Userland/Libraries/LibC/signal.cpp is missing an include. In theory, one might use LibCPP to detect things like this automatically, but let's do this one step after another.
89 lines
1.9 KiB
C++
89 lines
1.9 KiB
C++
/*
|
|
* Copyright (c) 2018-2021, Andreas Kling <kling@serenityos.org>
|
|
* Copyright (c) 2021, kleines Filmröllchen <malu.bertsch@gmail.com>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Assertions.h>
|
|
#include <AK/Noncopyable.h>
|
|
#include <AK/Types.h>
|
|
#include <pthread.h>
|
|
|
|
namespace Threading {
|
|
|
|
class Mutex {
|
|
AK_MAKE_NONCOPYABLE(Mutex);
|
|
AK_MAKE_NONMOVABLE(Mutex);
|
|
friend class ConditionVariable;
|
|
|
|
public:
|
|
Mutex()
|
|
: m_lock_count(0)
|
|
{
|
|
#ifndef AK_OS_SERENITY
|
|
pthread_mutexattr_t attr;
|
|
pthread_mutexattr_init(&attr);
|
|
pthread_mutexattr_settype(&attr, PTHREAD_MUTEX_RECURSIVE);
|
|
pthread_mutex_init(&m_mutex, &attr);
|
|
#endif
|
|
}
|
|
~Mutex()
|
|
{
|
|
VERIFY(m_lock_count == 0);
|
|
// FIXME: pthread_mutex_destroy() is not implemented.
|
|
}
|
|
|
|
void lock();
|
|
void unlock();
|
|
|
|
private:
|
|
#ifdef AK_OS_SERENITY
|
|
pthread_mutex_t m_mutex = PTHREAD_RECURSIVE_MUTEX_INITIALIZER_NP;
|
|
#else
|
|
pthread_mutex_t m_mutex;
|
|
#endif
|
|
unsigned m_lock_count { 0 };
|
|
};
|
|
|
|
class MutexLocker {
|
|
AK_MAKE_NONCOPYABLE(MutexLocker);
|
|
AK_MAKE_NONMOVABLE(MutexLocker);
|
|
|
|
public:
|
|
ALWAYS_INLINE explicit MutexLocker(Mutex& mutex)
|
|
: m_mutex(mutex)
|
|
{
|
|
lock();
|
|
}
|
|
ALWAYS_INLINE ~MutexLocker()
|
|
{
|
|
unlock();
|
|
}
|
|
ALWAYS_INLINE void unlock() { m_mutex.unlock(); }
|
|
ALWAYS_INLINE void lock() { m_mutex.lock(); }
|
|
|
|
private:
|
|
Mutex& m_mutex;
|
|
};
|
|
|
|
ALWAYS_INLINE void Mutex::lock()
|
|
{
|
|
pthread_mutex_lock(&m_mutex);
|
|
m_lock_count++;
|
|
}
|
|
|
|
ALWAYS_INLINE void Mutex::unlock()
|
|
{
|
|
VERIFY(m_lock_count > 0);
|
|
// FIXME: We need to protect the lock count with the mutex itself.
|
|
// This may be bad because we're not *technically* unlocked yet,
|
|
// but we're not handling any errors from pthread_mutex_unlock anyways.
|
|
m_lock_count--;
|
|
pthread_mutex_unlock(&m_mutex);
|
|
}
|
|
|
|
}
|