mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-22 02:26:11 +00:00
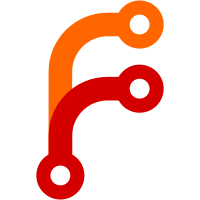
This aligns the Ladybird console implementation with the Browser console a bit more, which uses OutOfProcessWebView for rendering console output. This allows us to style the console output to try and match the system theme. Using a WebContentView is simpler than trying to style the old QTextEdit widget, as the console output is HTML with built-in "-libweb-palette-*" colors. These will override any color we set on the QTextEdit widget.
52 lines
1.3 KiB
C++
52 lines
1.3 KiB
C++
/*
|
|
* Copyright (c) 2020, Hunter Salyer <thefalsehonesty@gmail.com>
|
|
* Copyright (c) 2021-2022, Andreas Kling <kling@serenityos.org>
|
|
* Copyright (c) 2021, Sam Atkins <atkinssj@serenityos.org>
|
|
* Copyright (c) 2022, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/DeprecatedString.h>
|
|
#include <AK/Function.h>
|
|
#include <AK/Vector.h>
|
|
#include <QWidget>
|
|
|
|
class QLineEdit;
|
|
class WebContentView;
|
|
|
|
namespace Ladybird {
|
|
|
|
class ConsoleWidget final : public QWidget {
|
|
Q_OBJECT
|
|
public:
|
|
ConsoleWidget();
|
|
virtual ~ConsoleWidget() = default;
|
|
|
|
void notify_about_new_console_message(i32 message_index);
|
|
void handle_console_messages(i32 start_index, Vector<DeprecatedString> const& message_types, Vector<DeprecatedString> const& messages);
|
|
void print_source_line(StringView);
|
|
void print_html(StringView);
|
|
void reset();
|
|
|
|
WebContentView& view() { return *m_output_view; }
|
|
|
|
Function<void(DeprecatedString const&)> on_js_input;
|
|
Function<void(i32)> on_request_messages;
|
|
|
|
private:
|
|
void request_console_messages();
|
|
void clear_output();
|
|
|
|
WebContentView* m_output_view { nullptr };
|
|
QLineEdit* m_input { nullptr };
|
|
|
|
i32 m_highest_notified_message_index { -1 };
|
|
i32 m_highest_received_message_index { -1 };
|
|
bool m_waiting_for_messages { false };
|
|
};
|
|
|
|
}
|