mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-21 18:15:58 +00:00
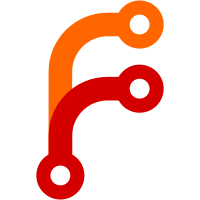
The main thread in the WebContent process is often busy with layout and running JavaScript. This can cause audio to sound jittery and crack. To avoid this behavior, we now drive audio on a secondary thread. Note: Browser on Serenity uses AudioServer, the connection for which is already handled on a secondary thread within LibAudio. So this only applies to Lagom. Rather than using LibThreading, our hands are tied to QThread for now. Internally, the Qt media objects use a QTimer, which is forbidden from running on a thread that is not a QThread (the debug console is spammed with messages pointing this out). Ideally, in the future AudioServer will be able to run for non-Serenity platforms, and most of this can be aligned with the Serenity implementation.
43 lines
971 B
C++
43 lines
971 B
C++
/*
|
|
* Copyright (c) 2023, Tim Flynn <trflynn89@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Error.h>
|
|
#include <AK/NonnullOwnPtr.h>
|
|
#include <AK/NonnullRefPtr.h>
|
|
#include <LibAudio/Forward.h>
|
|
#include <LibWeb/Platform/AudioCodecPlugin.h>
|
|
#include <QObject>
|
|
|
|
namespace Ladybird {
|
|
|
|
class AudioThread;
|
|
|
|
class AudioCodecPluginLadybird final
|
|
: public QObject
|
|
, public Web::Platform::AudioCodecPlugin {
|
|
Q_OBJECT
|
|
|
|
public:
|
|
static ErrorOr<NonnullOwnPtr<AudioCodecPluginLadybird>> create(NonnullRefPtr<Audio::Loader>);
|
|
virtual ~AudioCodecPluginLadybird() override;
|
|
|
|
virtual void resume_playback() override;
|
|
virtual void pause_playback() override;
|
|
virtual void set_volume(double) override;
|
|
virtual void seek(double) override;
|
|
|
|
virtual Duration duration() override;
|
|
|
|
private:
|
|
explicit AudioCodecPluginLadybird(NonnullOwnPtr<AudioThread>);
|
|
|
|
NonnullOwnPtr<AudioThread> m_audio_thread;
|
|
};
|
|
|
|
}
|