mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-22 02:26:11 +00:00
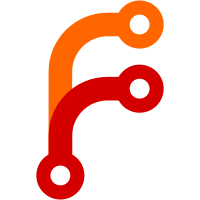
There was a small mishmash of argument order, as seen on the table: | Traits<T>::equals(U, T) | Traits<T>::equals(T, U) ============= | ======================= | ======================= uses equals() | HashMap | Vector, HashTable defines equals() | *String[^1] | ByteBuffer [^1]: String, DeprecatedString, their Fly-type equivalents and KString. This mostly meant that you couldn't use a StringView for finding a value in Vector<String>. I'm changing the order of arguments to make the trait type itself first (`Traits<T>::equals(T, U)`), as I think it's more expected and makes us more consistent with the rest of the functions that put the stored type first (like StringUtils functions and binary_serach). I've also renamed the variable name "other" in find functions to "entry" to give more importance to the value. With this change, each of the following lines will now compile successfully: Vector<String>().contains_slow("WHF!"sv); HashTable<String>().contains("WHF!"sv); HashMap<ByteBuffer, int>().contains("WHF!"sv.bytes());
42 lines
1.3 KiB
C++
42 lines
1.3 KiB
C++
/*
|
|
* Copyright (c) 2021, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Concepts.h>
|
|
#include <AK/Traits.h>
|
|
#include <AK/Types.h>
|
|
|
|
namespace AK {
|
|
|
|
template<typename TEndIterator, IteratorPairWith<TEndIterator> TIterator, typename TUnaryPredicate>
|
|
[[nodiscard]] constexpr TIterator find_if(TIterator first, TEndIterator last, TUnaryPredicate&& pred)
|
|
{
|
|
for (; first != last; ++first) {
|
|
if (pred(*first)) {
|
|
return first;
|
|
}
|
|
}
|
|
return last;
|
|
}
|
|
|
|
template<typename TEndIterator, IteratorPairWith<TEndIterator> TIterator, typename T>
|
|
[[nodiscard]] constexpr TIterator find(TIterator first, TEndIterator last, T const& value)
|
|
{
|
|
// FIXME: Use the iterator's trait type, and swap arguments in equals call.
|
|
return find_if(first, last, [&](auto const& v) { return Traits<T>::equals(value, v); });
|
|
}
|
|
|
|
template<typename TEndIterator, IteratorPairWith<TEndIterator> TIterator, typename T>
|
|
[[nodiscard]] constexpr size_t find_index(TIterator first, TEndIterator last, T const& value)
|
|
requires(requires(TIterator it) { it.index(); })
|
|
{
|
|
// FIXME: Use the iterator's trait type, and swap arguments in equals call.
|
|
return find_if(first, last, [&](auto const& v) { return Traits<T>::equals(value, v); }).index();
|
|
}
|
|
|
|
}
|