mirror of
https://github.com/SerenityOS/serenity
synced 2024-09-06 17:06:31 +00:00
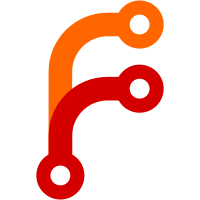
This commit un-deprecates DeprecatedString, and repurposes it as a byte string. As the null state has already been removed, there are no other particularly hairy blockers in repurposing this type as a byte string (what it _really_ is). This commit is auto-generated: $ xs=$(ack -l \bDeprecatedString\b\|deprecated_string AK Userland \ Meta Ports Ladybird Tests Kernel) $ perl -pie 's/\bDeprecatedString\b/ByteString/g; s/deprecated_string/byte_string/g' $xs $ clang-format --style=file -i \ $(git diff --name-only | grep \.cpp\|\.h) $ gn format $(git ls-files '*.gn' '*.gni')
67 lines
1.6 KiB
C++
67 lines
1.6 KiB
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <LibTest/TestCase.h>
|
|
|
|
#include <AK/ByteString.h>
|
|
#include <AK/WeakPtr.h>
|
|
#include <AK/Weakable.h>
|
|
|
|
#if defined(AK_COMPILER_CLANG)
|
|
# pragma clang diagnostic push
|
|
# pragma clang diagnostic ignored "-Wunused-private-field"
|
|
#endif
|
|
|
|
class SimpleWeakable : public Weakable<SimpleWeakable>
|
|
, public RefCounted<SimpleWeakable> {
|
|
public:
|
|
SimpleWeakable() = default;
|
|
|
|
private:
|
|
int m_member { 123 };
|
|
};
|
|
|
|
#if defined(AK_COMPILER_CLANG)
|
|
# pragma clang diagnostic pop
|
|
#endif
|
|
|
|
TEST_CASE(basic_weak)
|
|
{
|
|
WeakPtr<SimpleWeakable> weak1;
|
|
WeakPtr<SimpleWeakable> weak2;
|
|
|
|
{
|
|
auto simple = adopt_ref(*new SimpleWeakable);
|
|
weak1 = simple;
|
|
weak2 = simple;
|
|
EXPECT_EQ(weak1.is_null(), false);
|
|
EXPECT_EQ(weak2.is_null(), false);
|
|
EXPECT_EQ(weak1.strong_ref().ptr(), simple.ptr());
|
|
EXPECT_EQ(weak1.strong_ref().ptr(), weak2.strong_ref().ptr());
|
|
}
|
|
|
|
EXPECT_EQ(weak1.is_null(), true);
|
|
EXPECT_EQ(weak1.strong_ref().ptr(), nullptr);
|
|
EXPECT_EQ(weak1.strong_ref().ptr(), weak2.strong_ref().ptr());
|
|
}
|
|
|
|
TEST_CASE(weakptr_move)
|
|
{
|
|
WeakPtr<SimpleWeakable> weak1;
|
|
WeakPtr<SimpleWeakable> weak2;
|
|
|
|
{
|
|
auto simple = adopt_ref(*new SimpleWeakable);
|
|
weak1 = simple;
|
|
weak2 = move(weak1);
|
|
EXPECT_EQ(weak1.is_null(), true);
|
|
EXPECT_EQ(weak2.is_null(), false);
|
|
EXPECT_EQ(weak2.strong_ref().ptr(), simple.ptr());
|
|
}
|
|
|
|
EXPECT_EQ(weak2.is_null(), true);
|
|
}
|