mirror of
https://github.com/SerenityOS/serenity
synced 2024-10-15 20:33:10 +00:00
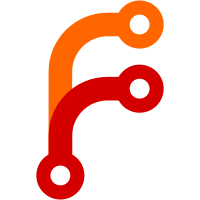
This allows us to use standard Serenity IPC infrastructure rather than manually creating FD-passing sockets. This also lets us use Serenity's WebDriver Session class, removing the copy previously used in Ladybird. This ensures any changes to Session in the future will be picked up by Ladybird for free.
70 lines
1.6 KiB
C++
70 lines
1.6 KiB
C++
/*
|
|
* Copyright (c) 2022, Andreas Kling <kling@serenityos.org>
|
|
* Copyright (c) 2022, Matthew Costa <ucosty@gmail.com>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#define AK_DONT_REPLACE_STD
|
|
|
|
#include "WebContentView.h"
|
|
#include <Browser/History.h>
|
|
#include <QBoxLayout>
|
|
#include <QLabel>
|
|
#include <QLineEdit>
|
|
#include <QToolBar>
|
|
#include <QWidget>
|
|
|
|
class BrowserWindow;
|
|
|
|
class Tab final : public QWidget {
|
|
Q_OBJECT
|
|
public:
|
|
Tab(BrowserWindow* window, StringView webdriver_content_ipc_path);
|
|
|
|
WebContentView& view() { return *m_view; }
|
|
|
|
void navigate(QString);
|
|
|
|
void debug_request(DeprecatedString const& request, DeprecatedString const& argument);
|
|
|
|
public slots:
|
|
void focus_location_editor();
|
|
void location_edit_return_pressed();
|
|
void page_title_changed(QString);
|
|
void page_favicon_changed(QIcon);
|
|
void back();
|
|
void forward();
|
|
void home();
|
|
void reload();
|
|
|
|
signals:
|
|
void title_changed(int id, QString);
|
|
void favicon_changed(int id, QIcon);
|
|
|
|
private:
|
|
virtual void resizeEvent(QResizeEvent*) override;
|
|
|
|
void update_hover_label();
|
|
|
|
QBoxLayout* m_layout;
|
|
QToolBar* m_toolbar { nullptr };
|
|
QLineEdit* m_location_edit { nullptr };
|
|
WebContentView* m_view { nullptr };
|
|
BrowserWindow* m_window { nullptr };
|
|
Browser::History m_history;
|
|
QString m_title;
|
|
QLabel* m_hover_label { nullptr };
|
|
|
|
OwnPtr<QAction> m_back_action;
|
|
OwnPtr<QAction> m_forward_action;
|
|
OwnPtr<QAction> m_home_action;
|
|
OwnPtr<QAction> m_reload_action;
|
|
|
|
int tab_index();
|
|
|
|
bool m_is_history_navigation { false };
|
|
};
|