mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-22 10:36:24 +00:00
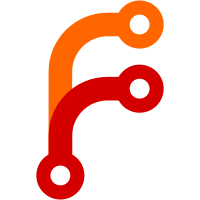
Previously, calling `.right()` on a `Gfx::Rect` would return the last column's coordinate still inside the rectangle, or `left + width - 1`. This is called 'endpoint inclusive' and does not make a lot of sense for `Gfx::Rect<float>` where a rectangle of width 5 at position (0, 0) would return 4 as its right side. This same problem exists for `.bottom()`. This changes `Gfx::Rect` to be endpoint exclusive, which gives us the nice property that `width = right - left` and `height = bottom - top`. It enables us to treat `Gfx::Rect<int>` and `Gfx::Rect<float>` exactly the same. All users of `Gfx::Rect` have been updated accordingly.
71 lines
2.1 KiB
C++
71 lines
2.1 KiB
C++
/*
|
|
* Copyright (c) 2021, Andreas Kling <kling@serenityos.org>
|
|
* Copyright (c) 2021, Mathias Jakobsen <mathias@jbcoding.com>
|
|
* Copyright (c) 2022, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include "DoubleClickArrowWidget.h"
|
|
#include <LibGUI/Painter.h>
|
|
#include <LibGfx/Font/Font.h>
|
|
|
|
REGISTER_WIDGET(MouseSettings, DoubleClickArrowWidget);
|
|
|
|
namespace MouseSettings {
|
|
|
|
void DoubleClickArrowWidget::set_double_click_speed(int speed)
|
|
{
|
|
if (m_double_click_speed == speed)
|
|
return;
|
|
m_double_click_speed = speed;
|
|
update();
|
|
}
|
|
|
|
DoubleClickArrowWidget::DoubleClickArrowWidget()
|
|
{
|
|
m_arrow_bitmap = Gfx::Bitmap::load_from_file("/res/graphics/double-click-down-arrow.png"sv).release_value_but_fixme_should_propagate_errors();
|
|
}
|
|
|
|
void DoubleClickArrowWidget::paint_event(GUI::PaintEvent& event)
|
|
{
|
|
GUI::Painter painter(*this);
|
|
painter.add_clip_rect(event.rect());
|
|
|
|
auto bottom_arrow_rect = m_arrow_bitmap->rect().centered_within(rect()).translated(0, m_arrow_bitmap->height() / 2);
|
|
|
|
painter.blit_filtered(bottom_arrow_rect.location(), *m_arrow_bitmap, m_arrow_bitmap->rect(), [&](Color color) {
|
|
return m_inverted ? color.inverted() : color;
|
|
});
|
|
|
|
auto top_arrow_rect = bottom_arrow_rect;
|
|
top_arrow_rect.translate_by(0, -(m_double_click_speed / 50));
|
|
|
|
painter.blit_filtered(top_arrow_rect.location(), *m_arrow_bitmap, m_arrow_bitmap->rect(), [&](Color color) {
|
|
return m_inverted ? color.inverted() : color;
|
|
});
|
|
|
|
auto text_rect = rect();
|
|
text_rect.set_y(bottom_arrow_rect.bottom() - 1);
|
|
text_rect.set_height(font().pixel_size_rounded_up());
|
|
}
|
|
|
|
void DoubleClickArrowWidget::mousedown_event(GUI::MouseEvent&)
|
|
{
|
|
auto double_click_in_progress = m_double_click_timer.is_valid();
|
|
auto elapsed_ms = double_click_in_progress ? m_double_click_timer.elapsed() : 0;
|
|
|
|
if (!double_click_in_progress || elapsed_ms > m_double_click_speed) {
|
|
m_double_click_timer.start();
|
|
return;
|
|
}
|
|
|
|
dbgln("Double-click in {}ms", elapsed_ms);
|
|
m_inverted = !m_inverted;
|
|
update();
|
|
|
|
m_double_click_timer.reset();
|
|
}
|
|
|
|
}
|