mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-22 10:36:24 +00:00
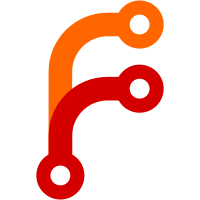
This commit un-deprecates DeprecatedString, and repurposes it as a byte string. As the null state has already been removed, there are no other particularly hairy blockers in repurposing this type as a byte string (what it _really_ is). This commit is auto-generated: $ xs=$(ack -l \bDeprecatedString\b\|deprecated_string AK Userland \ Meta Ports Ladybird Tests Kernel) $ perl -pie 's/\bDeprecatedString\b/ByteString/g; s/deprecated_string/byte_string/g' $xs $ clang-format --style=file -i \ $(git diff --name-only | grep \.cpp\|\.h) $ gn format $(git ls-files '*.gn' '*.gni')
81 lines
1.9 KiB
C++
81 lines
1.9 KiB
C++
/*
|
|
* Copyright (c) 2021, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/ByteString.h>
|
|
#include <AK/Hex.h>
|
|
#include <AK/NonnullRefPtr.h>
|
|
#include <AK/Utf8View.h>
|
|
#include <AK/Vector.h>
|
|
#include <LibGUI/Model.h>
|
|
|
|
struct Match {
|
|
u64 offset;
|
|
String value;
|
|
};
|
|
|
|
class SearchResultsModel final : public GUI::Model {
|
|
public:
|
|
enum Column {
|
|
Offset,
|
|
Value
|
|
};
|
|
|
|
explicit SearchResultsModel(Vector<Match> const&& matches)
|
|
: m_matches(move(matches))
|
|
{
|
|
}
|
|
|
|
virtual int row_count(const GUI::ModelIndex& = GUI::ModelIndex()) const override
|
|
{
|
|
return m_matches.size();
|
|
}
|
|
|
|
virtual int column_count(const GUI::ModelIndex&) const override
|
|
{
|
|
return 2;
|
|
}
|
|
|
|
ErrorOr<String> column_name(int column) const override
|
|
{
|
|
switch (column) {
|
|
case Column::Offset:
|
|
return "Offset"_string;
|
|
case Column::Value:
|
|
return "Value"_string;
|
|
}
|
|
VERIFY_NOT_REACHED();
|
|
}
|
|
|
|
virtual GUI::Variant data(const GUI::ModelIndex& index, GUI::ModelRole role) const override
|
|
{
|
|
if (role == GUI::ModelRole::TextAlignment)
|
|
return Gfx::TextAlignment::CenterLeft;
|
|
if (role == GUI::ModelRole::Custom) {
|
|
auto& match = m_matches.at(index.row());
|
|
return match.offset;
|
|
}
|
|
if (role == GUI::ModelRole::Display) {
|
|
auto& match = m_matches.at(index.row());
|
|
switch (index.column()) {
|
|
case Column::Offset:
|
|
return ByteString::formatted("{:#08X}", match.offset);
|
|
case Column::Value: {
|
|
Utf8View utf8_view(match.value);
|
|
if (!utf8_view.validate())
|
|
return {};
|
|
return StringView(match.value);
|
|
}
|
|
}
|
|
}
|
|
return {};
|
|
}
|
|
|
|
private:
|
|
Vector<Match> m_matches;
|
|
};
|