mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-21 10:05:32 +00:00
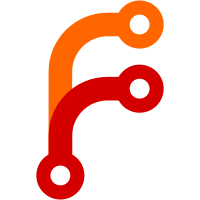
SPDX License Identifiers are a more compact / standardized way of representing file license information. See: https://spdx.dev/resources/use/#identifiers This was done with the `ambr` search and replace tool. ambr --no-parent-ignore --key-from-file --rep-from-file key.txt rep.txt *
43 lines
886 B
C++
43 lines
886 B
C++
/*
|
|
* Copyright (c) 2020, Liav A. <liavalb@hotmail.co.il>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Array.h>
|
|
#include <AK/ByteBuffer.h>
|
|
#include <AK/StringView.h>
|
|
#include <AK/Types.h>
|
|
|
|
namespace AK {
|
|
|
|
class UUID {
|
|
public:
|
|
UUID();
|
|
UUID(Array<u8, 16> uuid_buffer);
|
|
UUID(const StringView&);
|
|
~UUID() = default;
|
|
|
|
bool operator==(const UUID&) const;
|
|
bool operator!=(const UUID& other) const { return !(*this == other); }
|
|
bool operator<=(const UUID&) const = delete;
|
|
bool operator>=(const UUID&) const = delete;
|
|
bool operator<(const UUID&) const = delete;
|
|
bool operator>(const UUID&) const = delete;
|
|
|
|
String to_string() const;
|
|
bool is_zero() const;
|
|
|
|
private:
|
|
void convert_string_view_to_uuid(const StringView&);
|
|
void fill_buffer(ByteBuffer);
|
|
|
|
Array<u8, 16> m_uuid_buffer {};
|
|
};
|
|
|
|
}
|
|
|
|
using AK::UUID;
|