mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-21 10:05:32 +00:00
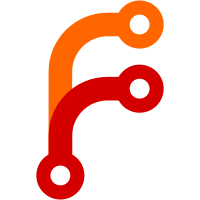
For non-x86 targets, it's not very nice to define inline functions in AK/Memory.h with asm volatile implementations. Guard this inline assembly with ARCH(I386) and provide portable alternatives. Since we always compile with optimizations, the hand-vectorized memset and memcpy seem to be of dubious value, but we'll keep them here until proven one way or another. This should fix the Lagom build on native M1 macOS that was reported on Discord the other day.
43 lines
828 B
C
43 lines
828 B
C
/*
|
|
* Copyright (c) 2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Types.h>
|
|
|
|
#if defined(KERNEL)
|
|
# include <Kernel/StdLib.h>
|
|
#else
|
|
# include <stdlib.h>
|
|
# include <string.h>
|
|
#endif
|
|
|
|
ALWAYS_INLINE void fast_u32_copy(u32* dest, const u32* src, size_t count)
|
|
{
|
|
#if ARCH(I386)
|
|
asm volatile(
|
|
"rep movsl\n"
|
|
: "+S"(src), "+D"(dest), "+c"(count)::"memory");
|
|
#else
|
|
__builtin_memcpy(dest, src, count * 4);
|
|
#endif
|
|
}
|
|
|
|
ALWAYS_INLINE void fast_u32_fill(u32* dest, u32 value, size_t count)
|
|
{
|
|
#if ARCH(I386)
|
|
asm volatile(
|
|
"rep stosl\n"
|
|
: "=D"(dest), "=c"(count)
|
|
: "D"(dest), "c"(count), "a"(value)
|
|
: "memory");
|
|
#else
|
|
for (auto* p = dest; p < (dest + count); ++p) {
|
|
*p = value;
|
|
}
|
|
#endif
|
|
}
|