mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-21 01:55:07 +00:00
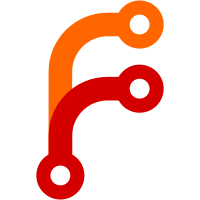
Problem: - `any_of` is implemented in 2 different ways, one for the entire container and a different implementation for a partial container using iterators. Solution: - Follow the "don't repeat yourself" (DRY) idiom and implement the entire container version in terms of the partial version.
33 lines
638 B
C++
33 lines
638 B
C++
/*
|
|
* Copyright (c) 2021, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Concepts.h>
|
|
#include <AK/Find.h>
|
|
#include <AK/Iterator.h>
|
|
|
|
namespace AK {
|
|
|
|
template<typename TEndIterator, IteratorPairWith<TEndIterator> TIterator>
|
|
constexpr bool any_of(
|
|
TIterator const& begin,
|
|
TEndIterator const& end,
|
|
auto const& predicate)
|
|
{
|
|
return find_if(begin, end, predicate) != end;
|
|
}
|
|
|
|
template<IterableContainer Container>
|
|
constexpr bool any_of(Container&& container, auto const& predicate)
|
|
{
|
|
return any_of(container.begin(), container.end(), predicate);
|
|
}
|
|
|
|
}
|
|
|
|
using AK::any_of;
|