mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-22 10:36:24 +00:00
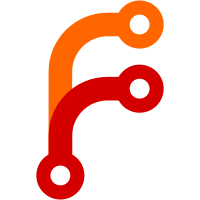
These were easy to pick-up as these pointers are assigned during the construction point and are never changed afterwards. This small change to these pointers will ensure that our code will not accidentally assign these pointers with a new object which is always a kind of bug we will want to prevent.
45 lines
1.1 KiB
C++
45 lines
1.1 KiB
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Bitmap.h>
|
|
#include <Kernel/Memory/VMObject.h>
|
|
#include <Kernel/UnixTypes.h>
|
|
|
|
namespace Kernel::Memory {
|
|
|
|
class InodeVMObject : public VMObject {
|
|
public:
|
|
virtual ~InodeVMObject() override;
|
|
|
|
Inode& inode() { return *m_inode; }
|
|
Inode const& inode() const { return *m_inode; }
|
|
|
|
size_t amount_dirty() const;
|
|
size_t amount_clean() const;
|
|
|
|
int release_all_clean_pages();
|
|
int try_release_clean_pages(int page_amount);
|
|
|
|
u32 writable_mappings() const;
|
|
|
|
protected:
|
|
explicit InodeVMObject(Inode&, FixedArray<RefPtr<PhysicalPage>>&&, Bitmap dirty_pages);
|
|
explicit InodeVMObject(InodeVMObject const&, FixedArray<RefPtr<PhysicalPage>>&&, Bitmap dirty_pages);
|
|
|
|
InodeVMObject& operator=(InodeVMObject const&) = delete;
|
|
InodeVMObject& operator=(InodeVMObject&&) = delete;
|
|
InodeVMObject(InodeVMObject&&) = delete;
|
|
|
|
virtual bool is_inode() const final { return true; }
|
|
|
|
NonnullRefPtr<Inode> const m_inode;
|
|
Bitmap m_dirty_pages;
|
|
};
|
|
|
|
}
|