mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-23 19:15:55 +00:00
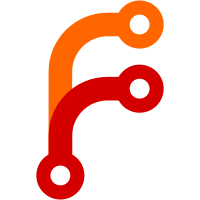
This patch switches away from {Nonnull,}LockRefPtr to the non-locking smart pointers throughout the kernel. I've looked at the handful of places where these were being persisted and I don't see any race situations. Note that the process file descriptor table (Process::m_fds) was already guarded via MutexProtected.
41 lines
1.4 KiB
C++
41 lines
1.4 KiB
C++
/*
|
|
* Copyright (c) 2021, Patrick Meyer <git@the-space.agency>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <Kernel/Devices/CharacterDevice.h>
|
|
#include <Kernel/Devices/KCOVInstance.h>
|
|
|
|
namespace Kernel {
|
|
class KCOVDevice final : public CharacterDevice {
|
|
friend class DeviceManagement;
|
|
|
|
public:
|
|
static HashMap<ProcessID, KCOVInstance*>* proc_instance;
|
|
static HashMap<ThreadID, KCOVInstance*>* thread_instance;
|
|
|
|
static NonnullLockRefPtr<KCOVDevice> must_create();
|
|
static void free_thread();
|
|
static void free_process();
|
|
|
|
// ^File
|
|
ErrorOr<NonnullLockRefPtr<Memory::VMObject>> vmobject_for_mmap(Process&, Memory::VirtualRange const&, u64& offset, bool shared) override;
|
|
virtual ErrorOr<NonnullRefPtr<OpenFileDescription>> open(int options) override;
|
|
|
|
protected:
|
|
KCOVDevice();
|
|
|
|
virtual StringView class_name() const override { return "KCOVDevice"sv; }
|
|
|
|
virtual bool can_read(OpenFileDescription const&, u64) const override final { return true; }
|
|
virtual bool can_write(OpenFileDescription const&, u64) const override final { return true; }
|
|
virtual ErrorOr<size_t> read(OpenFileDescription&, u64, UserOrKernelBuffer&, size_t) override { return EINVAL; }
|
|
virtual ErrorOr<size_t> write(OpenFileDescription&, u64, UserOrKernelBuffer const&, size_t) override { return EINVAL; }
|
|
virtual ErrorOr<void> ioctl(OpenFileDescription&, unsigned request, Userspace<void*> arg) override;
|
|
};
|
|
|
|
}
|