mirror of
https://github.com/SerenityOS/serenity
synced 2024-09-06 17:06:31 +00:00
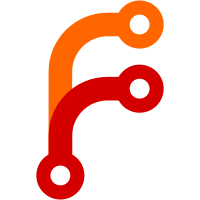
This patch reduces the O(n) tab completion to something like O(log(n)). The cache is just a sorted vector of strings and we binary search it to get a string matching our input, and then check the surrounding strings to see if we need to remove any characters. Also we no longer stat each file every time. Also added an #include in BinarySearch since it was using size_t. Oops. If `export` is called, we recache. Need to implement the `hash` builtin for when an executable has been added to a directory in PATH.
35 lines
695 B
C++
35 lines
695 B
C++
#pragma once
|
|
|
|
#include <AK/Types.h>
|
|
|
|
namespace AK {
|
|
|
|
template<typename T>
|
|
int integral_compare(const T& a, const T& b)
|
|
{
|
|
return a - b;
|
|
}
|
|
|
|
template<typename T, typename Compare>
|
|
T* binary_search(T* haystack, size_t haystack_size, const T& needle, Compare compare = integral_compare)
|
|
{
|
|
int low = 0;
|
|
int high = haystack_size - 1;
|
|
while (low <= high) {
|
|
int middle = (low + high) / 2;
|
|
int comparison = compare(needle, haystack[middle]);
|
|
if (comparison < 0)
|
|
high = middle - 1;
|
|
else if (comparison > 0)
|
|
low = middle + 1;
|
|
else
|
|
return &haystack[middle];
|
|
}
|
|
|
|
return nullptr;
|
|
}
|
|
|
|
}
|
|
|
|
using AK::binary_search;
|