mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-21 10:05:32 +00:00
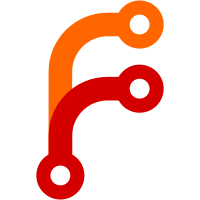
DeprecatedString (formerly String) has been with us since the start, and it has served us well. However, it has a number of shortcomings that I'd like to address. Some of these issues are hard if not impossible to solve incrementally inside of DeprecatedString, so instead of doing that, let's build a new String class and then incrementally move over to it instead. Problems in DeprecatedString: - It assumes string allocation never fails. This makes it impossible to use in allocation-sensitive contexts, and is the reason we had to ban DeprecatedString from the kernel entirely. - The awkward null state. DeprecatedString can be null. It's different from the empty state, although null strings are considered empty. All code is immediately nicer when using Optional<DeprecatedString> but DeprecatedString came before Optional, which is how we ended up like this. - The encoding of the underlying data is ambiguous. For the most part, we use it as if it's always UTF-8, but there have been cases where we pass around strings in other encodings (e.g ISO8859-1) - operator[] and length() are used to iterate over DeprecatedString one byte at a time. This is done all over the codebase, and will *not* give the right results unless the string is all ASCII. How we solve these issues in the new String: - Functions that may allocate now return ErrorOr<String> so that ENOMEM errors can be passed to the caller. - String has no null state. Use Optional<String> when needed. - String is always UTF-8. This is validated when constructing a String. We may need to add a bypass for this in the future, for cases where you have a known-good string, but for now: validate all the things! - There is no operator[] or length(). You can get the underlying data with bytes(), but for iterating over code points, you should be using an UTF-8 iterator. Furthermore, it has two nifty new features: - String implements a small string optimization (SSO) for strings that can fit entirely within a pointer. This means up to 3 bytes on 32-bit platforms, and 7 bytes on 64-bit platforms. Such small strings will not be heap-allocated. - String can create substrings without making a deep copy of the substring. Instead, the superstring gets +1 refcount from the substring, and it acts like a view into the superstring. To make substrings like this, use the substring_with_shared_superstring() API. One caveat: - String does not guarantee that the underlying data is null-terminated like DeprecatedString does today. While this was nifty in a handful of places where we were calling C functions, it did stand in the way of shared-superstring substrings.
38 lines
808 B
CMake
38 lines
808 B
CMake
set(AK_SOURCES
|
|
Assertions.cpp
|
|
Base64.cpp
|
|
DeprecatedString.cpp
|
|
FloatingPointStringConversions.cpp
|
|
FlyString.cpp
|
|
Format.cpp
|
|
FuzzyMatch.cpp
|
|
GenericLexer.cpp
|
|
Hex.cpp
|
|
JsonParser.cpp
|
|
JsonPath.cpp
|
|
JsonValue.cpp
|
|
kmalloc.cpp
|
|
LexicalPath.cpp
|
|
Random.cpp
|
|
StackInfo.cpp
|
|
String.cpp
|
|
StringBuilder.cpp
|
|
StringFloatingPointConversions.cpp
|
|
StringImpl.cpp
|
|
StringUtils.cpp
|
|
StringView.cpp
|
|
Time.cpp
|
|
URL.cpp
|
|
URLParser.cpp
|
|
Utf16View.cpp
|
|
Utf8View.cpp
|
|
UUID.cpp
|
|
)
|
|
# AK sources are included from many different places, such as the Kernel, LibC, and Loader
|
|
list(TRANSFORM AK_SOURCES PREPEND "${CMAKE_CURRENT_SOURCE_DIR}/")
|
|
|
|
set(AK_SOURCES ${AK_SOURCES} PARENT_SCOPE)
|
|
|
|
serenity_install_headers(AK)
|
|
serenity_install_sources(AK)
|