mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-22 18:46:18 +00:00
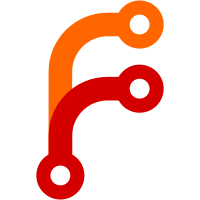
This implements the macOS API malloc_good_size() which returns the true allocation size for a given requested allocation size. This allows us to make use of all the available memory in a malloc chunk. For example, for a malloc request of 35 bytes our malloc would internally use a chunk of size 64, however the remaining 29 bytes would be unused. Knowing the true allocation size allows us to request more usable memory that would otherwise be wasted and make that available for Vector, HashTable and potentially other callers in the future.
77 lines
1.4 KiB
C++
77 lines
1.4 KiB
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#ifndef __serenity__
|
|
# include <new>
|
|
|
|
# ifndef AK_OS_MACOS
|
|
inline size_t malloc_good_size(size_t size) { return size; }
|
|
# else
|
|
# include <malloc/malloc.h>
|
|
# endif
|
|
#endif
|
|
|
|
#ifdef KERNEL
|
|
# define AK_MAKE_ETERNAL \
|
|
public: \
|
|
void* operator new(size_t size) { return kmalloc_eternal(size); } \
|
|
\
|
|
private:
|
|
#else
|
|
# define AK_MAKE_ETERNAL
|
|
#endif
|
|
|
|
#if defined(KERNEL)
|
|
# include <Kernel/Heap/kmalloc.h>
|
|
#else
|
|
# include <stdlib.h>
|
|
|
|
# define kcalloc calloc
|
|
# define kmalloc malloc
|
|
# define kmalloc_good_size malloc_good_size
|
|
# define kfree free
|
|
# define krealloc realloc
|
|
|
|
# ifdef __serenity__
|
|
|
|
# include <new>
|
|
|
|
inline void* operator new(size_t size)
|
|
{
|
|
return kmalloc(size);
|
|
}
|
|
|
|
inline void operator delete(void* ptr)
|
|
{
|
|
return kfree(ptr);
|
|
}
|
|
|
|
inline void operator delete(void* ptr, size_t)
|
|
{
|
|
return kfree(ptr);
|
|
}
|
|
|
|
inline void* operator new[](size_t size)
|
|
{
|
|
return kmalloc(size);
|
|
}
|
|
|
|
inline void operator delete[](void* ptr)
|
|
{
|
|
return kfree(ptr);
|
|
}
|
|
|
|
inline void operator delete[](void* ptr, size_t)
|
|
{
|
|
return kfree(ptr);
|
|
}
|
|
|
|
# endif
|
|
|
|
#endif
|