mirror of
https://github.com/SerenityOS/serenity
synced 2024-09-06 08:56:40 +00:00
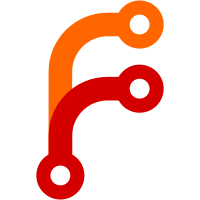
This changes the m_parts, m_dirname, m_basename, m_title and m_extension member variables to StringViews onto the m_string String. It also removes the m_is_absolute member in favour of computing if a path is absolute in the is_absolute() getter. Due to this, the canonicalize() method has been completely rewritten. The parts() getter still returns a Vector<String>, although it is no longer a const reference as m_parts is no longer a Vector<String>. Rather, it is constructed from the StringViews in m_parts upon request. The parts_view() getter has been added, which returns Vector<StringView> const&. Most previous users of parts() have been changed to use parts_view(), except where Strings are required. Due to this change, it's is now no longer allow to create temporary LexicalPath objects to call the dirname, basename, title, or extension getters on them because the returned StringViews will point to possible freed memory.
94 lines
2.3 KiB
C++
94 lines
2.3 KiB
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
* Copyright (c) 2021, Max Wipfli <max.wipfli@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/String.h>
|
|
#include <AK/Vector.h>
|
|
|
|
namespace AK {
|
|
|
|
class LexicalPath {
|
|
public:
|
|
LexicalPath() = default;
|
|
explicit LexicalPath(String);
|
|
|
|
bool is_absolute() const { return !m_string.is_empty() && m_string[0] == '/'; }
|
|
String const& string() const { return m_string; }
|
|
|
|
StringView const& dirname() const { return m_dirname; }
|
|
StringView const& basename() const { return m_basename; }
|
|
StringView const& title() const { return m_title; }
|
|
StringView const& extension() const { return m_extension; }
|
|
|
|
Vector<StringView> const& parts_view() const { return m_parts; }
|
|
Vector<String> parts() const;
|
|
|
|
bool has_extension(StringView const&) const;
|
|
|
|
void append(String const& component);
|
|
|
|
static String canonicalized_path(String);
|
|
static String relative_path(String absolute_path, String const& prefix);
|
|
|
|
template<typename... S>
|
|
static LexicalPath join(String const& first, S&&... rest)
|
|
{
|
|
StringBuilder builder;
|
|
builder.append(first);
|
|
((builder.append('/'), builder.append(forward<S>(rest))), ...);
|
|
|
|
return LexicalPath { builder.to_string() };
|
|
}
|
|
|
|
static String dirname(String path)
|
|
{
|
|
auto lexical_path = LexicalPath(move(path));
|
|
return lexical_path.dirname();
|
|
}
|
|
|
|
static String basename(String path)
|
|
{
|
|
auto lexical_path = LexicalPath(move(path));
|
|
return lexical_path.basename();
|
|
}
|
|
|
|
static String title(String path)
|
|
{
|
|
auto lexical_path = LexicalPath(move(path));
|
|
return lexical_path.title();
|
|
}
|
|
|
|
static String extension(String path)
|
|
{
|
|
auto lexical_path = LexicalPath(move(path));
|
|
return lexical_path.extension();
|
|
}
|
|
|
|
private:
|
|
void canonicalize();
|
|
|
|
Vector<StringView> m_parts;
|
|
String m_string;
|
|
StringView m_dirname;
|
|
StringView m_basename;
|
|
StringView m_title;
|
|
StringView m_extension;
|
|
};
|
|
|
|
template<>
|
|
struct Formatter<LexicalPath> : Formatter<StringView> {
|
|
void format(FormatBuilder& builder, LexicalPath const& value)
|
|
{
|
|
Formatter<StringView>::format(builder, value.string());
|
|
}
|
|
};
|
|
|
|
};
|
|
|
|
using AK::LexicalPath;
|