mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-21 10:05:32 +00:00
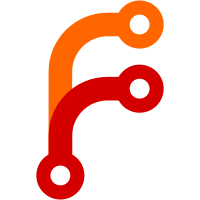
This makes it so these algorithms are usable with arbitrary iterators, as opposed to just instances of AK::SimpleIterator. This commit also makes the requirement of ::index() in find_index() explicit, as previously it was accepting any iterator.
41 lines
761 B
C++
41 lines
761 B
C++
/*
|
|
* Copyright (c) 2020, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Concepts.h>
|
|
#include <AK/Iterator.h>
|
|
|
|
namespace AK {
|
|
|
|
template<typename TEndIterator, IteratorPairWith<TEndIterator> TIterator>
|
|
constexpr bool all_of(
|
|
TIterator const& begin,
|
|
TEndIterator const& end,
|
|
auto const& predicate)
|
|
{
|
|
for (auto iter = begin; iter != end; ++iter) {
|
|
if (!predicate(*iter)) {
|
|
return false;
|
|
}
|
|
}
|
|
return true;
|
|
}
|
|
|
|
template<IterableContainer Container>
|
|
constexpr bool all_of(Container&& container, auto const& predicate)
|
|
{
|
|
for (auto&& entry : container) {
|
|
if (!predicate(entry))
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
|
|
}
|
|
|
|
using AK::all_of;
|