mirror of
https://github.com/SerenityOS/serenity
synced 2024-09-20 08:22:19 +00:00
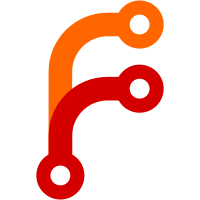
Instead of cowboy-calling the VESA BIOS in the bootloader, find the emulator VGA adapter by scanning the PCI bus. Then set up the desired video mode by sending device commands.
43 lines
727 B
C++
43 lines
727 B
C++
#pragma once
|
|
|
|
#include "types.h"
|
|
|
|
namespace IO {
|
|
|
|
inline byte in8(word port)
|
|
{
|
|
byte value;
|
|
asm volatile("inb %1, %0":"=a"(value):"Nd"(port));
|
|
return value;
|
|
}
|
|
|
|
inline word in16(word port)
|
|
{
|
|
word value;
|
|
asm volatile("inw %1, %0":"=a"(value):"Nd"(port));
|
|
return value;
|
|
}
|
|
|
|
inline dword in32(word port)
|
|
{
|
|
dword value;
|
|
asm volatile("inl %1, %0":"=a"(value):"Nd"(port));
|
|
return value;
|
|
}
|
|
|
|
inline void out8(word port, byte value)
|
|
{
|
|
asm volatile("outb %0, %1"::"a"(value), "Nd"(port));
|
|
}
|
|
|
|
inline void out16(word port, word value)
|
|
{
|
|
asm volatile("outw %0, %1"::"a"(value), "Nd"(port));
|
|
}
|
|
|
|
inline void out32(word port, dword value)
|
|
{
|
|
asm volatile("outl %0, %1"::"a"(value), "Nd"(port));
|
|
}
|
|
}
|