mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-22 18:46:18 +00:00
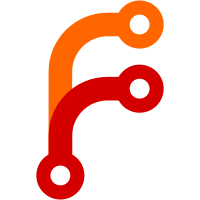
This class inherits from CircularQueue and adds the ability dequeue from the end of the queue using dequeue_end(). Note that I had to make some of CircularQueue's fields protected to properly implement dequeue_end.
25 lines
439 B
C++
25 lines
439 B
C++
#pragma once
|
|
|
|
#include <AK/Assertions.h>
|
|
#include <AK/CircularQueue.h>
|
|
#include <AK/Types.h>
|
|
|
|
namespace AK {
|
|
|
|
template<typename T, int Capacity>
|
|
class CircularDeque : public CircularQueue<T, Capacity> {
|
|
|
|
public:
|
|
T dequeue_end()
|
|
{
|
|
ASSERT(!this->is_empty());
|
|
T value = this->m_elements[(this->m_head + this->m_size - 1) % Capacity];
|
|
this->m_size--;
|
|
return value;
|
|
}
|
|
};
|
|
|
|
}
|
|
|
|
using AK::CircularDeque;
|