mirror of
https://github.com/SerenityOS/serenity
synced 2024-10-17 05:12:58 +00:00
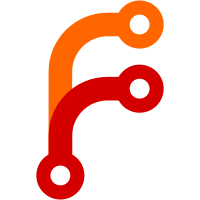
The handling of filesystem level errors was basically non-existing or consisting of `VERIFY_NOT_REACHED` assertions. Addressed this by * Adding `open` methods to `Heap` and `Database` which return errors. * Changing the interface of methods of these classes and clients downstream to propagate these errors. The constructors of `Heap` and `Database` don't open the underlying filesystem file anymore. The SQL statement handlers return an `SQLErrorCode::InternalError` error code if an error comes back from the lower levels. Note that some of these errors are things like duplicate index entry errors that should be caught before the SQL layer attempts to actually update the database. Added tests to catch attempts to open weird or non-existent files as databases. Finally, in between me writing this patch and submitting the PR the AK::Result<Foo, Bar> template got deprecated in favour of ErrorOr<Foo>. This resulted in more busywork.
61 lines
1.5 KiB
C++
61 lines
1.5 KiB
C++
/*
|
|
* Copyright (c) 2021, Jan de Visser <jan@de-visser.net>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/RefPtr.h>
|
|
#include <AK/String.h>
|
|
#include <LibCore/Object.h>
|
|
#include <LibSQL/Forward.h>
|
|
#include <LibSQL/Heap.h>
|
|
#include <LibSQL/Meta.h>
|
|
|
|
namespace SQL {
|
|
|
|
/**
|
|
* A Database object logically connects a Heap with the SQL data we want
|
|
* to store in it. It has BTree pointers for B-Trees holding the definitions
|
|
* of tables, columns, indexes, and other SQL objects.
|
|
*/
|
|
class Database : public Core::Object {
|
|
C_OBJECT(Database);
|
|
|
|
public:
|
|
~Database() override;
|
|
|
|
ErrorOr<void> open();
|
|
bool is_open() const { return m_open; }
|
|
ErrorOr<void> commit();
|
|
|
|
ErrorOr<void> add_schema(SchemaDef const&);
|
|
static Key get_schema_key(String const&);
|
|
ErrorOr<RefPtr<SchemaDef>> get_schema(String const&);
|
|
|
|
ErrorOr<void> add_table(TableDef& table);
|
|
static Key get_table_key(String const&, String const&);
|
|
ErrorOr<RefPtr<TableDef>> get_table(String const&, String const&);
|
|
|
|
ErrorOr<Vector<Row>> select_all(TableDef const&);
|
|
ErrorOr<Vector<Row>> match(TableDef const&, Key const&);
|
|
ErrorOr<void> insert(Row&);
|
|
ErrorOr<void> update(Row&);
|
|
|
|
private:
|
|
explicit Database(String);
|
|
|
|
bool m_open { false };
|
|
NonnullRefPtr<Heap> m_heap;
|
|
Serializer m_serializer;
|
|
RefPtr<BTree> m_schemas;
|
|
RefPtr<BTree> m_tables;
|
|
RefPtr<BTree> m_table_columns;
|
|
|
|
HashMap<u32, RefPtr<SchemaDef>> m_schema_cache;
|
|
HashMap<u32, RefPtr<TableDef>> m_table_cache;
|
|
};
|
|
|
|
}
|