mirror of
https://github.com/SerenityOS/serenity
synced 2024-09-16 06:30:41 +00:00
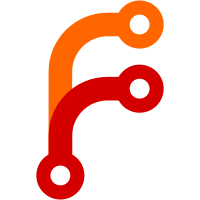
This was unintuitive, and only useful in a few cases. In the majority, users had to immediately call `stop()`, and several who did want the timer started would call `start()` on it immediately anyway. Case in point: There are only two places I had to add a manual `start()`.
68 lines
1.8 KiB
C++
68 lines
1.8 KiB
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
* Copyright (c) 2022, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Function.h>
|
|
#include <LibCore/Object.h>
|
|
|
|
namespace Core {
|
|
|
|
class Timer final : public Object {
|
|
C_OBJECT(Timer);
|
|
|
|
public:
|
|
static ErrorOr<NonnullRefPtr<Timer>> create_repeating(int interval_ms, Function<void()>&& timeout_handler, Object* parent = nullptr)
|
|
{
|
|
return adopt_nonnull_ref_or_enomem(new Timer(interval_ms, move(timeout_handler), parent));
|
|
}
|
|
static ErrorOr<NonnullRefPtr<Timer>> create_single_shot(int interval_ms, Function<void()>&& timeout_handler, Object* parent = nullptr)
|
|
{
|
|
auto timer = TRY(adopt_nonnull_ref_or_enomem(new Timer(interval_ms, move(timeout_handler), parent)));
|
|
timer->set_single_shot(true);
|
|
return timer;
|
|
}
|
|
|
|
virtual ~Timer() override = default;
|
|
|
|
void start();
|
|
void start(int interval_ms);
|
|
void restart();
|
|
void restart(int interval_ms);
|
|
void stop();
|
|
|
|
void set_active(bool);
|
|
|
|
bool is_active() const { return m_active; }
|
|
int interval() const { return m_interval_ms; }
|
|
void set_interval(int interval_ms)
|
|
{
|
|
if (m_interval_ms == interval_ms)
|
|
return;
|
|
m_interval_ms = interval_ms;
|
|
m_interval_dirty = true;
|
|
}
|
|
|
|
bool is_single_shot() const { return m_single_shot; }
|
|
void set_single_shot(bool single_shot) { m_single_shot = single_shot; }
|
|
|
|
Function<void()> on_timeout;
|
|
|
|
private:
|
|
explicit Timer(Object* parent = nullptr);
|
|
Timer(int interval_ms, Function<void()>&& timeout_handler, Object* parent = nullptr);
|
|
|
|
virtual void timer_event(TimerEvent&) override;
|
|
|
|
bool m_active { false };
|
|
bool m_single_shot { false };
|
|
bool m_interval_dirty { false };
|
|
int m_interval_ms { 0 };
|
|
};
|
|
|
|
}
|