mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-21 18:15:58 +00:00
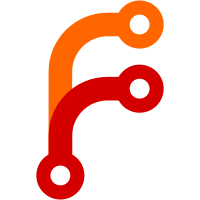
In standard C++, operators `new` and `new[]` are guaranteed to return a valid (non-null) pointer and throw an exception if the allocation couldn't be performed. Based on this, compilers did not check the returned pointer before attempting to use them for object construction. To avoid this, the allocator operators were changed to be `noexcept` in PR #7026, which made GCC emit the desired null checks. Unfortunately, this is a non-standard feature which meant that Clang would not accept these function definitions, as it did not match its expected declaration. To make compiling using Clang possible, the special "nothrow" versions of `new` are implemented in this commit. These take a tag type of `std::nothrow_t` (used for disambiguating from placement new/etc.), and are allowed by the standard to return null. There is a global variable, `std::nothrow`, declared with this type, which is also exported into the global namespace. To perform fallible allocations, the following syntax should be used: ```cpp auto ptr = new (nothrow) T; ``` As we don't support exceptions in the kernel, the only way of uphold the "throwing" new's guarantee is to abort if the allocation couldn't be performed. Once we have proper OOM handling in the kernel, this should only be used for critical allocations, where we wouldn't be able to recover from allocation failures anyway.
96 lines
1.8 KiB
C++
96 lines
1.8 KiB
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#ifndef __serenity__
|
|
# include <new>
|
|
|
|
# ifndef AK_OS_MACOS
|
|
extern "C" {
|
|
inline size_t malloc_good_size(size_t size) { return size; }
|
|
}
|
|
# else
|
|
# include <malloc/malloc.h>
|
|
# endif
|
|
#endif
|
|
|
|
#ifdef KERNEL
|
|
# define AK_MAKE_ETERNAL \
|
|
public: \
|
|
void* operator new(size_t size) { return kmalloc_eternal(size); } \
|
|
\
|
|
private:
|
|
#else
|
|
# define AK_MAKE_ETERNAL
|
|
#endif
|
|
|
|
#if defined(KERNEL)
|
|
# include <Kernel/Heap/kmalloc.h>
|
|
#else
|
|
# include <stdlib.h>
|
|
|
|
# define kcalloc calloc
|
|
# define kmalloc malloc
|
|
# define kmalloc_good_size malloc_good_size
|
|
# define kfree free
|
|
# define krealloc realloc
|
|
|
|
# ifdef __serenity__
|
|
|
|
# include <AK/Assertions.h>
|
|
# include <new>
|
|
|
|
inline void* operator new(size_t size)
|
|
{
|
|
void* ptr = kmalloc(size);
|
|
VERIFY(ptr);
|
|
return ptr;
|
|
}
|
|
|
|
inline void* operator new(size_t size, const std::nothrow_t&) noexcept
|
|
{
|
|
return kmalloc(size);
|
|
}
|
|
|
|
inline void operator delete(void* ptr) noexcept
|
|
{
|
|
return kfree(ptr);
|
|
}
|
|
|
|
inline void operator delete(void* ptr, size_t) noexcept
|
|
{
|
|
return kfree(ptr);
|
|
}
|
|
|
|
inline void* operator new[](size_t size)
|
|
{
|
|
void* ptr = kmalloc(size);
|
|
VERIFY(ptr);
|
|
return ptr;
|
|
}
|
|
|
|
inline void* operator new[](size_t size, const std::nothrow_t&) noexcept
|
|
{
|
|
return kmalloc(size);
|
|
}
|
|
|
|
inline void operator delete[](void* ptr) noexcept
|
|
{
|
|
return kfree(ptr);
|
|
}
|
|
|
|
inline void operator delete[](void* ptr, size_t) noexcept
|
|
{
|
|
return kfree(ptr);
|
|
}
|
|
|
|
# endif
|
|
|
|
#endif
|
|
|
|
using std::nothrow;
|