mirror of
https://github.com/SerenityOS/serenity
synced 2024-10-07 16:40:59 +00:00
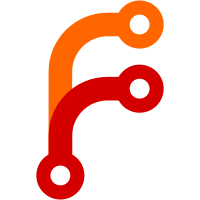
Adds an *almost fully featured BMP loader to process .bmp files. Features: - All header formats are supported - Full RLE4/8/24 support - Color scaling (e.g. distributing a 5-bit color throughout the 8-bit color spectrum, so 5-bit white is still 0xffffff) - Full BITMASK/ALPHABITMASK support *Not included: - 1D Huffman compression. Good luck actually finding a bmp in the wild that uses this - Use of any field in the V4/V5 header. Color spaces? Endpoints? No thanks :) This loader was tested with the images at https://entropymine.com/jason/bmpsuite/bmpsuite/html/bmpsuite.html. This loader correctly displays 81 out of the 90 total images (for reference, firefox displays 64 correctly). Note that not rendering the images at the bottom is counted as displaying correctly.
59 lines
2.2 KiB
C++
59 lines
2.2 KiB
C++
/*
|
|
* Copyright (c) 2020, Matthew Olsson <matthewcolsson@gmail.com>
|
|
* All rights reserved.
|
|
*
|
|
* Redistribution and use in source and binary forms, with or without
|
|
* modification, are permitted provided that the following conditions are met:
|
|
*
|
|
* 1. Redistributions of source code must retain the above copyright notice, this
|
|
* list of conditions and the following disclaimer.
|
|
*
|
|
* 2. Redistributions in binary form must reproduce the above copyright notice,
|
|
* this list of conditions and the following disclaimer in the documentation
|
|
* and/or other materials provided with the distribution.
|
|
*
|
|
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
|
|
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
|
|
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
|
|
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
|
|
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
|
|
* DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
|
|
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
|
|
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
|
|
* OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
|
|
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Vector.h>
|
|
#include <LibGfx/Bitmap.h>
|
|
#include <LibGfx/ImageDecoder.h>
|
|
|
|
namespace Gfx {
|
|
|
|
RefPtr<Gfx::Bitmap> load_bmp(const StringView& path);
|
|
|
|
struct BMPLoadingContext;
|
|
|
|
class BMPImageDecoderPlugin final : public ImageDecoderPlugin {
|
|
public:
|
|
virtual ~BMPImageDecoderPlugin() override;
|
|
BMPImageDecoderPlugin(const u8*, size_t);
|
|
|
|
virtual IntSize size() override;
|
|
virtual RefPtr<Gfx::Bitmap> bitmap() override;
|
|
virtual void set_volatile() override;
|
|
[[nodiscard]] virtual bool set_nonvolatile() override;
|
|
virtual bool sniff() override;
|
|
virtual bool is_animated() override;
|
|
virtual size_t loop_count() override;
|
|
virtual size_t frame_count() override;
|
|
virtual ImageFrameDescriptor frame(size_t i) override;
|
|
|
|
private:
|
|
OwnPtr<BMPLoadingContext> m_context;
|
|
};
|
|
|
|
}
|