mirror of
https://github.com/SerenityOS/serenity
synced 2024-09-20 00:12:20 +00:00
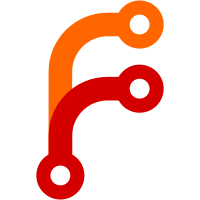
Instead of checking it at every call site (to generate EBADF), we make file_description(fd) return a KResultOr<NonnullRefPtr<FileDescription>>. This allows us to wrap all the calls in TRY(). :^) The only place that got a little bit messier from this is sys$mount(), and there's a whole bunch of things there in need of cleanup.
56 lines
1.6 KiB
C++
56 lines
1.6 KiB
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <Kernel/FileSystem/FileDescription.h>
|
|
#include <Kernel/Net/LocalSocket.h>
|
|
#include <Kernel/Process.h>
|
|
|
|
namespace Kernel {
|
|
|
|
KResultOr<FlatPtr> Process::sys$sendfd(int sockfd, int fd)
|
|
{
|
|
VERIFY_PROCESS_BIG_LOCK_ACQUIRED(this)
|
|
REQUIRE_PROMISE(sendfd);
|
|
auto socket_description = TRY(fds().file_description(sockfd));
|
|
if (!socket_description->is_socket())
|
|
return ENOTSOCK;
|
|
auto& socket = *socket_description->socket();
|
|
if (!socket.is_local())
|
|
return EAFNOSUPPORT;
|
|
if (!socket.is_connected())
|
|
return ENOTCONN;
|
|
|
|
auto passing_descriptor = TRY(fds().file_description(fd));
|
|
auto& local_socket = static_cast<LocalSocket&>(socket);
|
|
return local_socket.sendfd(*socket_description, *passing_descriptor);
|
|
}
|
|
|
|
KResultOr<FlatPtr> Process::sys$recvfd(int sockfd, int options)
|
|
{
|
|
VERIFY_PROCESS_BIG_LOCK_ACQUIRED(this)
|
|
REQUIRE_PROMISE(recvfd);
|
|
auto socket_description = TRY(fds().file_description(sockfd));
|
|
if (!socket_description->is_socket())
|
|
return ENOTSOCK;
|
|
auto& socket = *socket_description->socket();
|
|
if (!socket.is_local())
|
|
return EAFNOSUPPORT;
|
|
|
|
auto fd_allocation = TRY(m_fds.allocate());
|
|
|
|
auto& local_socket = static_cast<LocalSocket&>(socket);
|
|
auto received_description = TRY(local_socket.recvfd(*socket_description));
|
|
|
|
u32 fd_flags = 0;
|
|
if (options & O_CLOEXEC)
|
|
fd_flags |= FD_CLOEXEC;
|
|
|
|
m_fds[fd_allocation.fd].set(move(received_description), fd_flags);
|
|
return fd_allocation.fd;
|
|
}
|
|
|
|
}
|