mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-23 11:04:40 +00:00
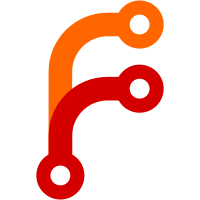
This commit reached that goal of "safely discarding" a filesystem by doing the following: 1. Stop using the s_file_system_map HashMap as it was an unsafe measure to access pointers of FileSystems. Instead, make sure to register all FileSystems at the VFS layer, with an IntrusiveList, to avoid problems related to OOM conditions. 2. Make sure to cleanly remove the DiskCache object from a BlockBased filesystem, so the destructor of such object will not need to do that in the destruction point. 3. For ext2 filesystems, don't cache the root inode at m_inode_cache HashMap. The reason for this is that when unmounting an ext2 filesystem, we lookup at the cache to see if there's a reference to a cached inode and if that's the case, we fail with EBUSY. If we keep the m_root_inode also being referenced at the m_inode_cache map, we have 2 references to that object, which will lead to fail with EBUSY. Also, it's much simpler to always ask for a root inode and get it immediately from m_root_inode, instead of looking up the cache for that inode.
96 lines
2.7 KiB
C++
96 lines
2.7 KiB
C++
/*
|
|
* Copyright (c) 2018-2021, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/AtomicRefCounted.h>
|
|
#include <AK/Error.h>
|
|
#include <AK/StringView.h>
|
|
#include <Kernel/FileSystem/InodeIdentifier.h>
|
|
#include <Kernel/Forward.h>
|
|
#include <Kernel/Library/LockRefPtr.h>
|
|
#include <Kernel/Locking/Mutex.h>
|
|
#include <Kernel/UnixTypes.h>
|
|
#include <Kernel/UserOrKernelBuffer.h>
|
|
|
|
namespace Kernel {
|
|
|
|
class FileSystem : public AtomicRefCounted<FileSystem> {
|
|
friend class Inode;
|
|
friend class VirtualFileSystem;
|
|
|
|
public:
|
|
virtual ~FileSystem();
|
|
|
|
FileSystemID fsid() const { return m_fsid; }
|
|
static void sync();
|
|
static void lock_all();
|
|
|
|
virtual ErrorOr<void> initialize() = 0;
|
|
virtual StringView class_name() const = 0;
|
|
virtual Inode& root_inode() = 0;
|
|
virtual bool supports_watchers() const { return false; }
|
|
|
|
bool is_readonly() const { return m_readonly; }
|
|
|
|
virtual unsigned total_block_count() const { return 0; }
|
|
virtual unsigned free_block_count() const { return 0; }
|
|
virtual unsigned total_inode_count() const { return 0; }
|
|
virtual unsigned free_inode_count() const { return 0; }
|
|
|
|
ErrorOr<void> prepare_to_unmount();
|
|
|
|
struct DirectoryEntryView {
|
|
DirectoryEntryView(StringView name, InodeIdentifier, u8 file_type);
|
|
|
|
StringView name;
|
|
InodeIdentifier inode;
|
|
u8 file_type { 0 };
|
|
};
|
|
|
|
virtual void flush_writes() { }
|
|
|
|
u64 block_size() const { return m_block_size; }
|
|
size_t fragment_size() const { return m_fragment_size; }
|
|
|
|
virtual bool is_file_backed() const { return false; }
|
|
|
|
// Converts file types that are used internally by the filesystem to DT_* types
|
|
virtual u8 internal_file_type_to_directory_entry_type(DirectoryEntryView const& entry) const { return entry.file_type; }
|
|
|
|
SpinlockProtected<size_t>& mounted_count(Badge<VirtualFileSystem>) { return m_attach_count; }
|
|
|
|
protected:
|
|
FileSystem();
|
|
|
|
void set_block_size(u64 size) { m_block_size = size; }
|
|
void set_fragment_size(size_t size) { m_fragment_size = size; }
|
|
|
|
virtual ErrorOr<void> prepare_to_clear_last_mount() { return {}; }
|
|
|
|
mutable Mutex m_lock { "FS"sv };
|
|
|
|
private:
|
|
FileSystemID m_fsid;
|
|
u64 m_block_size { 0 };
|
|
size_t m_fragment_size { 0 };
|
|
bool m_readonly { false };
|
|
|
|
SpinlockProtected<size_t> m_attach_count { LockRank::FileSystem, 0 };
|
|
IntrusiveListNode<FileSystem> m_file_system_node;
|
|
};
|
|
|
|
}
|
|
|
|
namespace AK {
|
|
|
|
template<>
|
|
struct Traits<Kernel::InodeIdentifier> : public GenericTraits<Kernel::InodeIdentifier> {
|
|
static unsigned hash(Kernel::InodeIdentifier const& inode) { return pair_int_hash(inode.fsid().value(), inode.index().value()); }
|
|
};
|
|
|
|
}
|