mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-23 11:04:40 +00:00
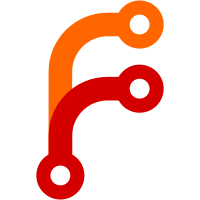
Before this change, we had File::mmap() which did all the work of setting up a VMObject, and then creating a Region in the current process's address space. This patch simplifies the interface by removing the region part. Files now only have to return a suitable VMObject from vmobject_for_mmap(), and then sys$mmap() itself will take care of actually mapping it into the address space. This fixes an issue where we'd try to block on I/O (for inode metadata lookup) while holding the address space spinlock. It also reduces time spent holding the address space lock.
39 lines
1.4 KiB
C++
39 lines
1.4 KiB
C++
/*
|
|
* Copyright (c) 2021-2022, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <Kernel/FileSystem/File.h>
|
|
#include <Kernel/Memory/AnonymousVMObject.h>
|
|
|
|
namespace Kernel {
|
|
|
|
class AnonymousFile final : public File {
|
|
public:
|
|
static ErrorOr<NonnullLockRefPtr<AnonymousFile>> try_create(NonnullLockRefPtr<Memory::AnonymousVMObject> vmobject)
|
|
{
|
|
return adopt_nonnull_lock_ref_or_enomem(new (nothrow) AnonymousFile(move(vmobject)));
|
|
}
|
|
|
|
virtual ~AnonymousFile() override;
|
|
|
|
virtual ErrorOr<NonnullLockRefPtr<Memory::VMObject>> vmobject_for_mmap(Process&, Memory::VirtualRange const&, u64& offset, bool shared) override;
|
|
|
|
private:
|
|
virtual StringView class_name() const override { return "AnonymousFile"sv; }
|
|
virtual ErrorOr<NonnullOwnPtr<KString>> pseudo_path(OpenFileDescription const&) const override;
|
|
virtual bool can_read(OpenFileDescription const&, u64) const override { return false; }
|
|
virtual bool can_write(OpenFileDescription const&, u64) const override { return false; }
|
|
virtual ErrorOr<size_t> read(OpenFileDescription&, u64, UserOrKernelBuffer&, size_t) override { return ENOTSUP; }
|
|
virtual ErrorOr<size_t> write(OpenFileDescription&, u64, UserOrKernelBuffer const&, size_t) override { return ENOTSUP; }
|
|
|
|
explicit AnonymousFile(NonnullLockRefPtr<Memory::AnonymousVMObject>);
|
|
|
|
NonnullLockRefPtr<Memory::AnonymousVMObject> m_vmobject;
|
|
};
|
|
|
|
}
|