mirror of
https://github.com/SerenityOS/serenity
synced 2024-10-17 13:22:58 +00:00
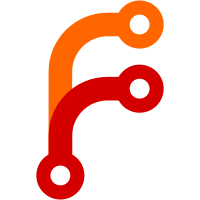
In its current state, ScummVM seems to invoke these methods just after destroying the current GL context. According to the OpenGL spec: "Issuing GL commands when the program does not have a current context results in undefined behavior, up to and including program termination." Our old behavior was to deref a `nullptr`, which isn't that great. For now, protect these two methods. If other ports seem to misbehave as well, we can always expand the check to other methods.
43 lines
798 B
C++
43 lines
798 B
C++
/*
|
|
* Copyright (c) 2021, Jesse Buhagiar <jooster669@gmail.com>
|
|
* Copyright (c) 2021, Stephan Unverwerth <s.unverwerth@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include "GLContext.h"
|
|
#include "SoftwareGLContext.h"
|
|
#include <LibGfx/Bitmap.h>
|
|
|
|
__attribute__((visibility("hidden"))) GL::GLContext* g_gl_context;
|
|
|
|
namespace GL {
|
|
|
|
GLContext::~GLContext()
|
|
{
|
|
if (g_gl_context == this)
|
|
make_context_current(nullptr);
|
|
}
|
|
|
|
OwnPtr<GLContext> create_context(Gfx::Bitmap& bitmap)
|
|
{
|
|
auto context = adopt_own(*new SoftwareGLContext(bitmap));
|
|
|
|
if (!g_gl_context)
|
|
g_gl_context = context;
|
|
|
|
return context;
|
|
}
|
|
|
|
void make_context_current(GLContext* context)
|
|
{
|
|
g_gl_context = context;
|
|
}
|
|
|
|
void present_context(GLContext* context)
|
|
{
|
|
context->present();
|
|
}
|
|
|
|
}
|