mirror of
https://github.com/SerenityOS/serenity
synced 2024-11-02 22:04:47 +00:00
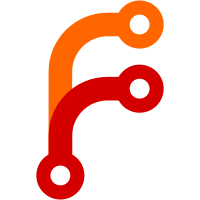
This makes it much clearer what this cast actually does: it will VERIFY that the thing we're casting is a T (using is<T>()).
49 lines
1.3 KiB
C++
49 lines
1.3 KiB
C++
/*
|
|
* Copyright (c) 2020-2021, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Assertions.h>
|
|
#include <AK/Platform.h>
|
|
#include <AK/StdLibExtras.h>
|
|
|
|
namespace AK {
|
|
|
|
template<typename OutputType, typename InputType>
|
|
ALWAYS_INLINE bool is(InputType& input)
|
|
{
|
|
if constexpr (requires { input.template fast_is<OutputType>(); }) {
|
|
return input.template fast_is<OutputType>();
|
|
}
|
|
return dynamic_cast<CopyConst<InputType, OutputType>*>(&input);
|
|
}
|
|
|
|
template<typename OutputType, typename InputType>
|
|
ALWAYS_INLINE bool is(InputType* input)
|
|
{
|
|
return input && is<OutputType>(*input);
|
|
}
|
|
|
|
template<typename OutputType, typename InputType>
|
|
ALWAYS_INLINE CopyConst<InputType, OutputType>* verify_cast(InputType* input)
|
|
{
|
|
static_assert(IsBaseOf<InputType, OutputType>);
|
|
VERIFY(!input || is<OutputType>(*input));
|
|
return static_cast<CopyConst<InputType, OutputType>*>(input);
|
|
}
|
|
|
|
template<typename OutputType, typename InputType>
|
|
ALWAYS_INLINE CopyConst<InputType, OutputType>& verify_cast(InputType& input)
|
|
{
|
|
static_assert(IsBaseOf<InputType, OutputType>);
|
|
VERIFY(is<OutputType>(input));
|
|
return static_cast<CopyConst<InputType, OutputType>&>(input);
|
|
}
|
|
|
|
}
|
|
|
|
using AK::is;
|
|
using AK::verify_cast;
|