mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-22 02:26:11 +00:00
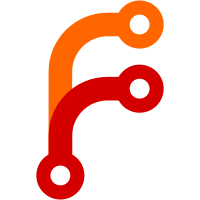
NoAllocationGuard is an RAII stack guard that prevents allocations while it exists. This is done through a thread-local global flag which causes malloc to crash on a VERIFY if it is false. The guard allows for recursion. The intended use case for this class is in real-time audio code. In such code, allocations are really bad, and this is an easy way of dynamically enforcing the no-allocations rule while giving the user good feedback if it is violated. Before real-time audio code is executed, e.g. in LibDSP, a NoAllocationGuard is instantiated. This is not done with this commit, as currently some code in LibDSP may still incorrectly allocate in real- time situations. Other use cases for the Kernel have also been added, so this commit builds on the previous to add the support both in Userland and in the Kernel.
62 lines
1.2 KiB
C++
62 lines
1.2 KiB
C++
/*
|
|
* Copyright (c) 2022, kleines Filmröllchen <malu.bertsch@gmail.com>.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Forward.h>
|
|
#include <AK/Noncopyable.h>
|
|
|
|
#if defined(KERNEL)
|
|
# include <Kernel/Arch/Processor.h>
|
|
# include <Kernel/Heap/kmalloc.h>
|
|
#else
|
|
# include <LibC/mallocdefs.h>
|
|
#endif
|
|
|
|
namespace AK {
|
|
|
|
class NoAllocationGuard {
|
|
AK_MAKE_NONCOPYABLE(NoAllocationGuard);
|
|
AK_MAKE_NONMOVABLE(NoAllocationGuard);
|
|
|
|
public:
|
|
NoAllocationGuard()
|
|
: m_allocation_enabled_previously(get_thread_allocation_state())
|
|
{
|
|
set_thread_allocation_state(false);
|
|
}
|
|
|
|
~NoAllocationGuard()
|
|
{
|
|
set_thread_allocation_state(m_allocation_enabled_previously);
|
|
}
|
|
|
|
private:
|
|
static bool get_thread_allocation_state()
|
|
{
|
|
#if defined(KERNEL)
|
|
return Processor::current_thread()->get_allocation_enabled();
|
|
#else
|
|
return s_allocation_enabled;
|
|
#endif
|
|
}
|
|
|
|
static void set_thread_allocation_state(bool value)
|
|
{
|
|
#if defined(KERNEL)
|
|
Processor::current_thread()->set_allocation_enabled(value);
|
|
#else
|
|
s_allocation_enabled = value;
|
|
#endif
|
|
}
|
|
|
|
bool m_allocation_enabled_previously;
|
|
};
|
|
|
|
}
|
|
|
|
using AK::NoAllocationGuard;
|