mirror of
https://github.com/SerenityOS/serenity
synced 2024-09-06 17:06:31 +00:00
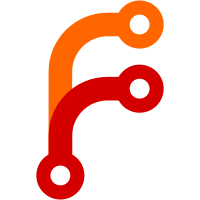
In the continuous effort of better handling OOM in the kernel, we want to move away from all AK::String usage. One of the final pieces left to accomplish this goal is replacing all of the usages of `String::formatted` with something that can actually propagate failure. The StringBuilder API was enhanced in the recent past to propagate failure and thus a slightly modified version of what exists in `AK::format` will work well for implementing failable format with `KString`.
72 lines
2.1 KiB
C++
72 lines
2.1 KiB
C++
/*
|
|
* Copyright (c) 2021, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <AK/Format.h>
|
|
#include <AK/StringBuilder.h>
|
|
#include <Kernel/KString.h>
|
|
|
|
extern bool g_in_early_boot;
|
|
|
|
namespace Kernel {
|
|
|
|
ErrorOr<NonnullOwnPtr<KString>> KString::try_create(StringView string)
|
|
{
|
|
char* characters = nullptr;
|
|
size_t length = string.length();
|
|
auto new_string = TRY(KString::try_create_uninitialized(length, characters));
|
|
if (!string.is_empty())
|
|
__builtin_memcpy(characters, string.characters_without_null_termination(), length);
|
|
characters[length] = '\0';
|
|
return new_string;
|
|
}
|
|
|
|
ErrorOr<NonnullOwnPtr<KString>> KString::vformatted(StringView fmtstr, AK::TypeErasedFormatParams& params)
|
|
{
|
|
StringBuilder builder;
|
|
TRY(AK::vformat(builder, fmtstr, params));
|
|
return try_create(builder.string_view());
|
|
}
|
|
|
|
NonnullOwnPtr<KString> KString::must_create(StringView string)
|
|
{
|
|
// We can only enforce success during early boot.
|
|
VERIFY(g_in_early_boot);
|
|
return KString::try_create(string).release_value();
|
|
}
|
|
|
|
ErrorOr<NonnullOwnPtr<KString>> KString::try_create_uninitialized(size_t length, char*& characters)
|
|
{
|
|
size_t allocation_size = sizeof(KString) + (sizeof(char) * length) + sizeof(char);
|
|
auto* slot = kmalloc(allocation_size);
|
|
if (!slot)
|
|
return ENOMEM;
|
|
auto new_string = TRY(adopt_nonnull_own_or_enomem(new (slot) KString(length)));
|
|
characters = new_string->m_characters;
|
|
return new_string;
|
|
}
|
|
|
|
NonnullOwnPtr<KString> KString::must_create_uninitialized(size_t length, char*& characters)
|
|
{
|
|
// We can only enforce success during early boot.
|
|
VERIFY(g_in_early_boot);
|
|
return KString::try_create_uninitialized(length, characters).release_value();
|
|
}
|
|
|
|
ErrorOr<NonnullOwnPtr<KString>> KString::try_clone() const
|
|
{
|
|
return try_create(view());
|
|
}
|
|
|
|
void KString::operator delete(void* string)
|
|
{
|
|
if (!string)
|
|
return;
|
|
size_t allocation_size = sizeof(KString) + (sizeof(char) * static_cast<KString*>(string)->m_length) + sizeof(char);
|
|
kfree_sized(string, allocation_size);
|
|
}
|
|
|
|
}
|