mirror of
https://github.com/SerenityOS/serenity
synced 2024-11-03 03:29:38 +00:00
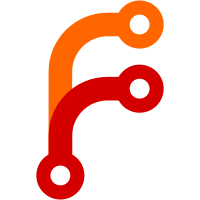
We have a new, improved string type coming up in AK (OOM aware, no null state), and while it's going to use UTF-8, the name UTF8String is a mouthful - so let's free up the String name by renaming the existing class. Making the old one have an annoying name will hopefully also help with quick adoption :^)
46 lines
879 B
C++
46 lines
879 B
C++
/*
|
|
* Copyright (c) 2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/ByteBuffer.h>
|
|
#include <AK/Error.h>
|
|
#include <AK/StringView.h>
|
|
|
|
#ifdef KERNEL
|
|
# include <Kernel/KString.h>
|
|
#else
|
|
# include <AK/DeprecatedString.h>
|
|
#endif
|
|
|
|
namespace AK {
|
|
|
|
constexpr u8 decode_hex_digit(char digit)
|
|
{
|
|
if (digit >= '0' && digit <= '9')
|
|
return digit - '0';
|
|
if (digit >= 'a' && digit <= 'f')
|
|
return 10 + (digit - 'a');
|
|
if (digit >= 'A' && digit <= 'F')
|
|
return 10 + (digit - 'A');
|
|
return 255;
|
|
}
|
|
|
|
ErrorOr<ByteBuffer> decode_hex(StringView);
|
|
|
|
#ifdef KERNEL
|
|
ErrorOr<NonnullOwnPtr<Kernel::KString>> encode_hex(ReadonlyBytes);
|
|
#else
|
|
DeprecatedString encode_hex(ReadonlyBytes);
|
|
#endif
|
|
|
|
}
|
|
|
|
#if USING_AK_GLOBALLY
|
|
using AK::decode_hex;
|
|
using AK::decode_hex_digit;
|
|
using AK::encode_hex;
|
|
#endif
|