mirror of
https://github.com/SerenityOS/serenity
synced 2024-10-08 17:09:41 +00:00
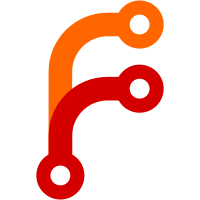
This is more logical and allows us to solve the problem of non-blocking TCP sockets getting stuck in SocketRole::None. The only complication is that a single LocalSocket may be shared between two file descriptions (on the connect and accept sides), and should have two different roles depending from which side you look at it. To deal with it, Socket::role() is made a virtual method that accepts a file description, and LocalSocket internally tracks which FileDescription is the which one and returns a correct role.
24 lines
838 B
C++
24 lines
838 B
C++
#pragma once
|
|
|
|
#include <Kernel/Net/IPv4Socket.h>
|
|
|
|
class UDPSocket final : public IPv4Socket {
|
|
public:
|
|
static NonnullRefPtr<UDPSocket> create(int protocol);
|
|
virtual ~UDPSocket() override;
|
|
|
|
static SocketHandle<UDPSocket> from_port(u16);
|
|
static void for_each(Function<void(UDPSocket&)>);
|
|
|
|
private:
|
|
explicit UDPSocket(int protocol);
|
|
virtual const char* class_name() const override { return "UDPSocket"; }
|
|
static Lockable<HashMap<u16, UDPSocket*>>& sockets_by_port();
|
|
|
|
virtual int protocol_receive(const KBuffer&, void* buffer, size_t buffer_size, int flags) override;
|
|
virtual int protocol_send(const void*, int) override;
|
|
virtual KResult protocol_connect(FileDescription&, ShouldBlock) override;
|
|
virtual int protocol_allocate_local_port() override;
|
|
virtual KResult protocol_bind() override;
|
|
};
|