mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-21 01:55:07 +00:00
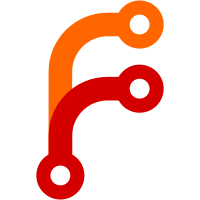
This is the initial port of Lagom to win32. This will enable developers to use Lagom as an alternative to vanilla STL/StandardC++Library - which gives a much richer environment (think QtCore - but modern). My main incentive - is to have a native Windows Ladybird working. I am starting with AK, which does not yet fully compile (on mingw). When AK is compiling (currently fails building StringBuffer.cpp) - I will continue to LibCore and then the rest of the user space libraries (excluding the GUI, which will be another different effort). Most of the code is happily stollen from Andrew Kaster's fork - he deserves the credit. Co-authored-by: Andrew Kaster <akaster@serenityos.org>
61 lines
1.1 KiB
C++
61 lines
1.1 KiB
C++
/*
|
|
* Copyright (c) 2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Platform.h>
|
|
#include <AK/Types.h>
|
|
|
|
#if defined(__serenity__) || defined(AK_OS_ANDROID)
|
|
# include <stdlib.h>
|
|
#endif
|
|
|
|
#if defined(__unix__)
|
|
# include <unistd.h>
|
|
#endif
|
|
|
|
#if defined(AK_OS_MACOS)
|
|
# include <sys/random.h>
|
|
#endif
|
|
|
|
#if defined(AK_OS_WINDOWS)
|
|
# include <random>
|
|
# include <unistd.h>
|
|
#endif
|
|
|
|
namespace AK {
|
|
|
|
inline void fill_with_random([[maybe_unused]] void* buffer, [[maybe_unused]] size_t length)
|
|
{
|
|
#if defined(__serenity__) || defined(AK_OS_ANDROID)
|
|
arc4random_buf(buffer, length);
|
|
#elif defined(OSS_FUZZ)
|
|
#elif defined(__unix__) or defined(AK_OS_MACOS)
|
|
[[maybe_unused]] int rc = getentropy(buffer, length);
|
|
#else
|
|
char* char_buffer = static_cast<char*>(buffer);
|
|
for (size_t i = 0; i < length; i++) {
|
|
char_buffer[i] = std::rand();
|
|
}
|
|
#endif
|
|
}
|
|
|
|
template<typename T>
|
|
inline T get_random()
|
|
{
|
|
T t;
|
|
fill_with_random(&t, sizeof(T));
|
|
return t;
|
|
}
|
|
|
|
u32 get_random_uniform(u32 max_bounds);
|
|
|
|
}
|
|
|
|
using AK::fill_with_random;
|
|
using AK::get_random;
|
|
using AK::get_random_uniform;
|